本题要求定义一个复数类Complex,类的声明见给出的代码,请给出类的完整实现,并通过测试程序。类的声明包括如下内容: 数据成员,复数类的实部u和虚部v; 对复数类实部和虚部的访问函数; 构造函数; 加号、减号运算符重载(遵守复数的运算规则); 输入、输出运算符重载。 类的声明: class Complex{ private: double u; double v; public: Complex(double _u=0,double _v=0); Complex operator+(const Complex&b); Complex operator-(const Complex&b); double getU() const; double getV() const; friend ostream&operator<<(ostream&os,const Complex&c); friend istream&operator>>(istream&is,Complex&c); };
时间: 2024-01-19 20:04:06 浏览: 24
可以按照如下方式实现Complex类:
```cpp
#include <iostream>
using namespace std;
class Complex {
private:
double u; // 实部
double v; // 虚部
public:
Complex(double _u = 0, double _v = 0) : u(_u), v(_v) {} // 构造函数
double getU() const { return u; } // 实部访问函数
double getV() const { return v; } // 虚部访问函数
Complex operator+(const Complex& b) { // 加法运算符重载
return Complex(u + b.u, v + b.v);
}
Complex operator-(const Complex& b) { // 减法运算符重载
return Complex(u - b.u, v - b.v);
}
friend ostream& operator<<(ostream& os, const Complex& c) { // 输出运算符重载
os << c.u << "+" << c.v << "i";
return os;
}
friend istream& operator>>(istream& is, Complex& c) { // 输入运算符重载
is >> c.u >> c.v;
return is;
}
};
```
在这个实现中,我们首先定义了Complex类,包含私有属性u和v表示复数的实部和虚部。这个类还包含了一个构造函数,用来初始化u和v。对于u和v,我们提供了公有的访问函数getU和getV,用来获取它们的值。
为了支持复数的加法和减法,我们重载了加号运算符和减号运算符,分别计算两个复数对象的实部和虚部之和、之差,并返回一个新的复数对象。
为了支持复数的输入和输出,我们重载了输入运算符和输出运算符。输入运算符从标准输入流中读取两个浮点数,分别作为复数的实部和虚部;输出运算符将复数的实部和虚部以"a+bi"的形式输出到标准输出流中。
在程序中,我们可以创建Complex对象,并使用各种运算符对它们进行加减运算,也可以将它们输入输出到标准输入输出流中。例如:
```cpp
int main() {
Complex c1(1, 2), c2(3, 4);
Complex c3 = c1 + c2;
Complex c4 = c1 - c2;
cout << c1 << " + " << c2 << " = " << c3 << endl;
cout << c1 << " - " << c2 << " = " << c4 << endl;
cin >> c1 >> c2;
cout << "c1 = " << c1 << endl;
cout << "c2 = " << c2 << endl;
return 0;
}
```
相关推荐
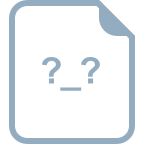
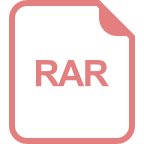
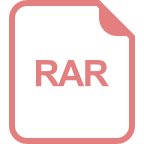











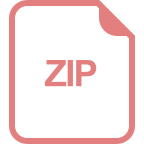
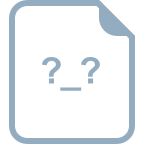