java把列表转为树结构
时间: 2023-10-11 20:15:25 浏览: 34
将列表转换为树结构可以采用递归的方式实现。具体步骤如下:
1. 定义树节点类,包含节点值和左右节点引用。
```
class TreeNode {
int val;
TreeNode left;
TreeNode right;
TreeNode(int x) { val = x; }
}
```
2. 定义转换方法,传入列表和树的深度(从0开始),返回根节点。
```
public TreeNode listToTree(List<Integer> list, int depth) {
if (list == null || list.isEmpty() || depth < 0) {
return null;
}
int size = list.size();
int index = (int)Math.pow(2, depth) - 1;
if (index >= size) {
return null;
}
TreeNode root = new TreeNode(list.get(index));
root.left = listToTree(list.subList(0, index), depth - 1);
root.right = listToTree(list.subList(index + 1, size), depth - 1);
return root;
}
```
3. 在程序中调用转换方法,将列表转换为树。
```
List<Integer> list = Arrays.asList(1, 2, 3, 4, 5, 6, 7);
TreeNode root = listToTree(list, 2);
```
其中,列表中的元素按照从上到下,从左到右的顺序排列,如上面的列表代表深度为2的满二叉树(根节点为1,左子树为2和3,右子树为4和5)。
相关推荐
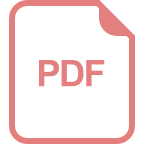
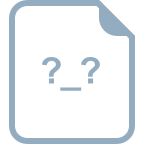













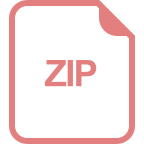