C#中用有一段代码返回返回List<string> list = new List<string>();中50至100行的数据
时间: 2023-06-04 14:08:12 浏览: 56
C是编程语言中的一种基础语言,由贝尔实验室的Dennis Ritchie所创建。它的特点包括效率高、语法简洁、可移植性强等,因此在许多系统软件和嵌入式系统的开发中得到广泛应用。C语言也是许多其他编程语言的基础,如C++、Java等。
相关问题
你写一个图书管理系统系统,这个系统在vs中用c#语言写后端代码,在vsCode中用vue写前端代码
好的,下面是一个简单的图书管理系统的后端代码示例,使用C#语言编写,可以在Visual Studio中运行:
```
using System;
using System.Collections.Generic;
namespace BookManagementSystem
{
class Program
{
static List<Book> bookList = new List<Book>();
static void Main(string[] args)
{
Console.WriteLine("Welcome to the Book Management System!");
while (true)
{
Console.WriteLine("1. Add a book");
Console.WriteLine("2. Search for a book");
Console.WriteLine("3. View all books");
Console.WriteLine("4. Exit");
int choice = int.Parse(Console.ReadLine());
switch (choice)
{
case 1:
AddBook();
break;
case 2:
SearchBook();
break;
case 3:
ViewAllBooks();
break;
case 4:
Environment.Exit(0);
break;
default:
Console.WriteLine("Invalid choice!");
break;
}
}
}
static void AddBook()
{
Console.WriteLine("Enter book title:");
string title = Console.ReadLine();
Console.WriteLine("Enter author name:");
string author = Console.ReadLine();
Console.WriteLine("Enter publication year:");
int year = int.Parse(Console.ReadLine());
Book book = new Book(title, author, year);
bookList.Add(book);
Console.WriteLine("Book added successfully!");
}
static void SearchBook()
{
Console.WriteLine("Enter book title:");
string title = Console.ReadLine();
foreach (Book book in bookList)
{
if (book.Title.Equals(title, StringComparison.OrdinalIgnoreCase))
{
Console.WriteLine("Book found:");
Console.WriteLine(book);
return;
}
}
Console.WriteLine("Book not found!");
}
static void ViewAllBooks()
{
foreach (Book book in bookList)
{
Console.WriteLine(book);
}
}
}
class Book
{
public string Title { get; set; }
public string Author { get; set; }
public int Year { get; set; }
public Book(string title, string author, int year)
{
Title = title;
Author = author;
Year = year;
}
public override string ToString()
{
return Title + " by " + Author + " (" + Year + ")";
}
}
}
```
下面是一个简单的图书管理系统的前端代码示例,使用Vue.js编写,可以在VS Code中运行:
```
<template>
<div>
<h1>Book Management System</h1>
<form @submit.prevent="addBook">
<label for="title">Title:</label>
<input type="text" id="title" v-model="newBook.title" required>
<br>
<label for="author">Author:</label>
<input type="text" id="author" v-model="newBook.author" required>
<br>
<label for="year">Publication Year:</label>
<input type="number" id="year" v-model.number="newBook.year" required>
<br>
<button>Add Book</button>
</form>
<hr>
<h2>Search for a Book</h2>
<form @submit.prevent="searchBook">
<label for="searchTitle">Title:</label>
<input type="text" id="searchTitle" v-model="searchTitle" required>
<br>
<button>Search</button>
</form>
<hr>
<h2>All Books</h2>
<ul>
<li v-for="book in bookList">{{ book.title }} by {{ book.author }} ({{ book.year }})</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
newBook: {
title: "",
author: "",
year: 0
},
searchTitle: "",
bookList: []
};
},
methods: {
addBook() {
this.bookList.push({
title: this.newBook.title,
author: this.newBook.author,
year: this.newBook.year
});
this.newBook.title = "";
this.newBook.author = "";
this.newBook.year = 0;
},
searchBook() {
for (let i = 0; i < this.bookList.length; i++) {
if (this.bookList[i].title.toLowerCase() === this.searchTitle.toLowerCase()) {
alert(this.bookList[i].title + " by " + this.bookList[i].author + " (" + this.bookList[i].year + ")");
return;
}
}
alert("Book not found!");
}
}
};
</script>
```
c#.net在一个richtextbox中用listbox实现代码自动提示
可以通过以下步骤实现:
1. 在窗体中添加一个 RichTextBox 和一个 ListBox 控件。
2. 在 RichTextBox 的 TextChanged 事件中,获取当前光标所在行的文本,并根据该文本获取匹配的代码提示列表。
3. 将匹配的提示项添加到 ListBox 中,并显示在 RichTextBox 下方。
4. 监听 ListBox 的 SelectedIndexChanged 事件,当选中一个提示项时,将其插入到 RichTextBox 中。
以下是一个简单的示例代码:
```
private void richTextBox1_TextChanged(object sender, EventArgs e)
{
// 获取当前光标所在行的文本
int index = richTextBox1.SelectionStart;
int line = richTextBox1.GetLineFromCharIndex(index);
string text = richTextBox1.Lines[line];
// 根据文本获取匹配的代码提示列表
List<string> suggestions = GetSuggestions(text);
// 清空 ListBox,并添加提示项
listBox1.Items.Clear();
listBox1.Items.AddRange(suggestions.ToArray());
// 显示 ListBox
if (suggestions.Count > 0)
{
listBox1.Visible = true;
listBox1.Width = richTextBox1.Width;
listBox1.Top = richTextBox1.Top + (line + 1) * richTextBox1.Font.Height;
listBox1.Left = richTextBox1.Left;
}
else
{
listBox1.Visible = false;
}
}
private void listBox1_SelectedIndexChanged(object sender, EventArgs e)
{
// 将选中的提示项插入到 RichTextBox 中
int index = richTextBox1.SelectionStart;
int line = richTextBox1.GetLineFromCharIndex(index);
int start = richTextBox1.GetFirstCharIndexFromLine(line);
int length = index - start;
string suggestion = listBox1.SelectedItem.ToString();
richTextBox1.SelectionStart = start;
richTextBox1.SelectionLength = length;
richTextBox1.SelectedText = suggestion;
listBox1.Visible = false;
}
private List<string> GetSuggestions(string text)
{
// 根据文本获取匹配的代码提示列表
List<string> suggestions = new List<string>();
if (text.StartsWith("using "))
{
suggestions.Add("System");
suggestions.Add("System.Collections");
suggestions.Add("System.IO");
suggestions.Add("System.Linq");
suggestions.Add("System.Text");
}
else if (text.StartsWith("Console."))
{
suggestions.Add("Write");
suggestions.Add("WriteLine");
suggestions.Add("Read");
suggestions.Add("ReadLine");
}
return suggestions;
}
```
在 GetSuggestions 方法中,可以根据实际需求生成代码提示列表,例如从一个 XML 文件中读取提示项。
相关推荐
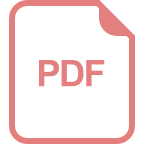







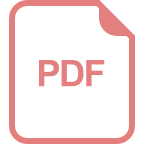
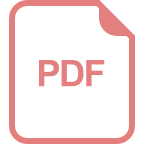
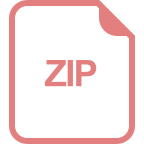