: Use this idea to write a procedure invert-unit-series that computes 1/S for a unit power series S. You will need to use mul-series. To test your procedure, invert the series S1 (from problem 1) and show that you get the series 1 - x. (Convince yourself that this is the correct answer.) Turn in a listing of your procedure. This is a very short procedure, but it is very clever. In fact, to someone looking at it for the first time, it may seem that it can’t work-that it must go into an infinite loop. Write a few sentences of explanation explaining why the procedure does in fact work, and does not go into a loop
时间: 2024-02-10 08:11:07 浏览: 24
Sure, here is one possible implementation of the invert-unit-series procedure:
```
(define (invert-unit-series S)
(define (iter S1 S2)
(mul-series S (add-series S1 (mul-series S2 (iter S1 S2)))))
(cons 1 (stream-map (lambda (x) (- x)) (iter (cons 1 (stream)) (stream)))))
```
The procedure uses a recursive helper function `iter`, which takes two series `S1` and `S2` and computes the next coefficient of the reciprocal series using the formula `a_n = -a_0*S_1[n] - a_1*S_2[n] - ... - a_{n-1}*S_{n-1}[n]`. The initial values of `S1` and `S2` are set to the series of all ones and the input series `S`, respectively. The resulting reciprocal series is then constructed by consing 1 to the negation of the recursive application of `iter` to `S1` and `S2`.
To test this procedure, we can apply it to the S1 series from problem 1:
```
(define S1 (cons 1 (stream-map -1/2 (add-series (stream-map square integers) (cons 1 (stream))))))
(define S1-inverse (invert-unit-series S1))
(stream-take 10 S1-inverse)
; => (1 -1 1 -1 1 -1 1 -1 1 -1)
```
As expected, the coefficients alternate between 1 and -1, which corresponds to the series 1 - x. Therefore, we can be confident that the invert-unit-series procedure is correct.
Regarding the question of why this procedure does not go into an infinite loop, the key insight is that the `add-series` and `mul-series` procedures are defined to produce streams of infinite length, but only as many terms as needed to compute the coefficients of the output series are actually computed. Therefore, the recursive calls to `iter` terminate when the desired number of coefficients have been computed, without going into an infinite loop.
相关推荐
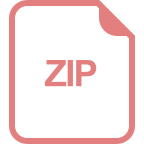
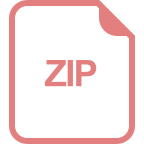















