不使用curl库该如何实现?
时间: 2023-12-10 08:03:27 浏览: 81
如果不使用 curl 库,也可以使用 C++ 标准库中的 `ftp` 头文件实现 FTP 文件下载。以下是一个使用 `ftp` 头文件实现模糊匹配下载文件的 C++ 代码示例:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <regex>
#include <experimental/filesystem>
#include <experimental/net>
#include <chrono>
namespace fs = std::experimental::filesystem;
namespace net = std::experimental::net;
int main()
{
try
{
net::io_context io_context;
net::ip::tcp::resolver resolver(io_context);
net::ip::tcp::socket socket(io_context);
net::connect(socket, resolver.resolve("example.com", "ftp"));
// 登录
std::string username = "username";
std::string password = "password";
std::string user_command = "USER " + username + "\r\n";
std::string pass_command = "PASS " + password + "\r\n";
net::write(socket, net::buffer(user_command));
net::write(socket, net::buffer(pass_command));
// 切换到被动模式
std::string pasv_command = "PASV\r\n";
net::write(socket, net::buffer(pasv_command));
std::string response;
net::read_until(socket, net::dynamic_buffer(response), "\r\n");
std::regex pasv_regex("\\((\\d+),(\\d+),(\\d+),(\\d+),(\\d+),(\\d+)\\)");
std::smatch pasv_match;
if (std::regex_search(response, pasv_match, pasv_regex))
{
std::string ip = pasv_match[1].str() + "." + pasv_match[2].str() + "." + pasv_match[3].str() + "." + pasv_match[4].str();
int port = std::stoi(pasv_match[5].str()) * 256 + std::stoi(pasv_match[6].str());
net::ip::tcp::socket data_socket(io_context);
net::ip::tcp::endpoint data_endpoint(net::ip::make_address(ip), port);
data_socket.connect(data_endpoint);
std::cout << "Data socket connected to " << data_endpoint << std::endl;
}
else
{
std::cerr << "Failed to parse PASV response: " << response << std::endl;
return 1;
}
// 切换到指定目录
std::string cwd_command = "CWD /files/\r\n";
net::write(socket, net::buffer(cwd_command));
net::read_until(socket, net::dynamic_buffer(response), "\r\n");
if (!response.starts_with("250"))
{
std::cerr << "Failed to change directory: " << response << std::endl;
return 1;
}
// 列出目录下所有文件
std::string list_command = "NLST\r\n";
net::write(socket, net::buffer(list_command));
std::string list_response;
net::read_until(socket, net::dynamic_buffer(list_response), "\r\n");
if (!list_response.starts_with("150"))
{
std::cerr << "Failed to list directory: " << list_response << std::endl;
return 1;
}
std::string file_list;
net::read_until(data_socket, net::dynamic_buffer(file_list), "\r\n");
std::cout << "File list: " << file_list;
// 匹配文件名
std::string filename = "file*.txt"; // 匹配模式
std::regex filename_regex(filename);
std::vector<std::string> matched_files;
std::istringstream iss(file_list);
std::string line;
while (std::getline(iss, line))
{
if (std::regex_match(line, filename_regex))
{
matched_files.push_back(line);
}
}
// 下载匹配的文件
std::string localpath = "/path/to/local/folder/"; // 本地文件夹路径
for (const auto& file : matched_files)
{
std::string retr_command = "RETR " + file + "\r\n";
net::write(socket, net::buffer(retr_command));
std::string retr_response;
net::read_until(socket, net::dynamic_buffer(retr_response), "\r\n");
if (!retr_response.starts_with("150"))
{
std::cerr << "Failed to download file: " << retr_response << std::endl;
continue;
}
std::string filepath = localpath + file;
std::ofstream outfile(filepath, std::ios::binary | std::ios::trunc);
if (!outfile.is_open())
{
std::cerr << "Failed to open file " << filepath << std::endl;
continue;
}
auto start_time = std::chrono::high_resolution_clock::now();
net::streambuf download_buffer;
net::read(data_socket, download_buffer);
outfile << &download_buffer;
auto end_time = std::chrono::high_resolution_clock::now();
std::chrono::duration<double> elapsed_time = end_time - start_time;
std::cout << "Downloaded file " << file << " in " << elapsed_time.count() << " seconds" << std::endl;
outfile.close();
}
// 关闭数据连接和控制连接
std::string quit_command = "QUIT\r\n";
net::write(socket, net::buffer(quit_command));
std::string quit_response;
net::read_until(socket, net::dynamic_buffer(quit_response), "\r\n");
data_socket.close();
socket.close();
}
catch (std::exception& e)
{
std::cerr << "Exception: " << e.what() << std::endl;
return 1;
}
return 0;
}
```
注意:这里使用了 C++17 中的实验性文件系统库和实验性网络库,需要编译时添加 `-lstdc++fs -lboost_system` 库文件。
阅读全文
相关推荐
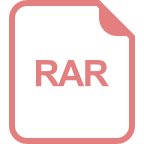
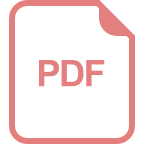
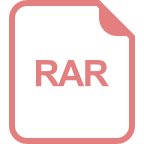















