从共享内存读取json数据
时间: 2023-08-16 21:07:09 浏览: 48
从共享内存读取JSON数据需要进行以下步骤:
1. 获取共享内存的指针。
2. 从共享内存指针所指向的内存空间读取JSON数据。
3. 解析JSON数据。
4. 关闭共享内存。
以下是一个示例代码,演示如何从共享内存读取JSON数据:
```c++
#include <iostream>
#include <cstring>
#include <sys/ipc.h>
#include <sys/shm.h>
#include <jsoncpp/json/json.h>
using namespace std;
int main() {
// 获取共享内存的指针
key_t key = ftok("data.json", 'R');
int shmid = shmget(key, 0, 0666);
char *shm = (char*) shmat(shmid, NULL, 0);
// 从共享内存指针所指向的内存空间读取JSON数据
string jsonContent(shm, strlen(shm));
// 解析JSON数据
Json::Value root;
Json::Reader reader;
bool parsingSuccessful = reader.parse(jsonContent, root);
if (!parsingSuccessful) {
cout << "Failed to parse JSON data." << endl;
return 1;
}
// 输出JSON数据
cout << "JSON data:" << endl
<< root.toStyledString() << endl;
// 关闭共享内存
shmdt(shm);
return 0;
}
```
注意,这个示例代码仅仅是演示如何从共享内存读取JSON数据,实际应用中还需要考虑并发访问、内存大小等问题。
相关推荐
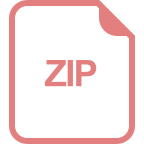
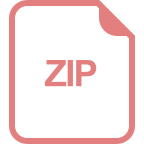
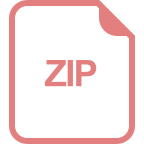
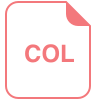
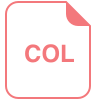
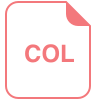
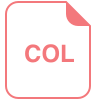
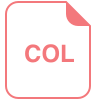









