c语言单链表选择排序法
时间: 2023-07-21 09:09:36 浏览: 45
以下是C语言单链表选择排序法的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct node {
int data;
struct node *next;
} Node;
// 创建节点
Node *create_node(int data) {
Node *node = (Node *)malloc(sizeof(Node));
node->data = data;
node->next = NULL;
return node;
}
// 插入节点
void insert_node(Node **head, Node *node) {
if (*head == NULL) {
*head = node;
} else {
Node *temp = *head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = node;
}
}
// 选择排序
void selection_sort(Node **head) {
Node *current = *head;
while (current != NULL) {
Node *min = current;
Node *temp = current->next;
while (temp != NULL) {
if (temp->data < min->data) {
min = temp;
}
temp = temp->next;
}
// 交换节点数据
int temp_data = current->data;
current->data = min->data;
min->data = temp_data;
current = current->next;
}
}
// 打印链表
void print_list(Node *head) {
while (head != NULL) {
printf("%d ", head->data);
head = head->next;
}
printf("\n");
}
int main() {
Node *head = NULL;
insert_node(&head, create_node(5));
insert_node(&head, create_node(2));
insert_node(&head, create_node(8));
insert_node(&head, create_node(3));
insert_node(&head, create_node(9));
printf("排序前的链表:\n");
print_list(head);
selection_sort(&head);
printf("排序后的链表:\n");
print_list(head);
return 0;
}
```
在上面的代码中,我们定义了链表节点结构体 Node,并创建了 create_node() 函数来创建节点,insert_node() 函数来插入节点,print_list() 函数来打印链表。同时,我们实现了 selection_sort() 函数来对链表进行选择排序。在 selection_sort() 函数中,我们使用两个指针 current 和 min 来遍历链表,并找到链表中的最小节点,然后交换节点数据。最后,我们在 main() 函数中进行测试,输出排序前和排序后的链表。
相关推荐
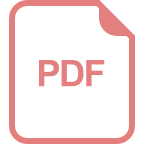
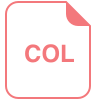
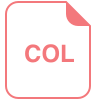
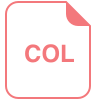
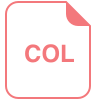
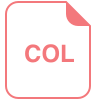








