基于二维数组类的矩阵运算方法的实现
时间: 2023-10-04 08:14:05 浏览: 112
以下是基于二维数组类的矩阵运算方法的实现代码,包括矩阵加法、矩阵减法、矩阵乘法和矩阵转置:
```java
public class Matrix {
private int rows; // 矩阵行数
private int cols; // 矩阵列数
private double[][] data; // 矩阵数据
public Matrix(int rows, int cols) {
this.rows = rows;
this.cols = cols;
this.data = new double[rows][cols];
}
// 矩阵加法
public Matrix add(Matrix other) {
if (this.rows != other.rows || this.cols != other.cols) {
throw new IllegalArgumentException("Matrix sizes do not match.");
}
Matrix result = new Matrix(rows, cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
result.data[i][j] = this.data[i][j] + other.data[i][j];
}
}
return result;
}
// 矩阵减法
public Matrix subtract(Matrix other) {
if (this.rows != other.rows || this.cols != other.cols) {
throw new IllegalArgumentException("Matrix sizes do not match.");
}
Matrix result = new Matrix(rows, cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
result.data[i][j] = this.data[i][j] - other.data[i][j];
}
}
return result;
}
// 矩阵乘法
public Matrix multiply(Matrix other) {
if (this.cols != other.rows) {
throw new IllegalArgumentException("Invalid matrix sizes for multiplication.");
}
Matrix result = new Matrix(this.rows, other.cols);
for (int i = 0; i < this.rows; i++) {
for (int j = 0; j < other.cols; j++) {
double sum = 0;
for (int k = 0; k < this.cols; k++) {
sum += this.data[i][k] * other.data[k][j];
}
result.data[i][j] = sum;
}
}
return result;
}
// 矩阵转置
public Matrix transpose() {
Matrix result = new Matrix(cols, rows);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
result.data[j][i] = this.data[i][j];
}
}
return result;
}
}
```
以上是一个简单的实现。需要注意的是,矩阵运算需要满足一定的条件,如加法和减法的矩阵大小必须相同,乘法的左矩阵列数必须等于右矩阵行数。在实际应用中,还需要考虑更多的情况。
阅读全文
相关推荐
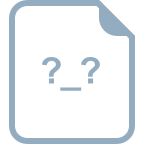
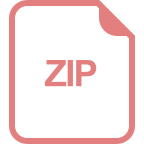
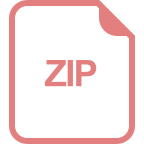
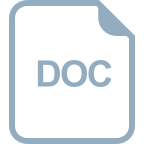
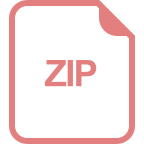
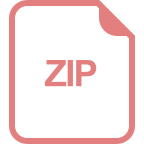
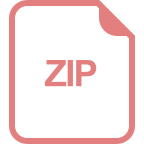
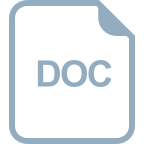
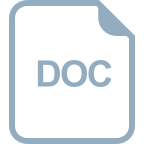
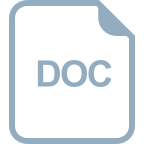
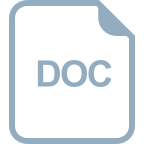
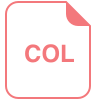
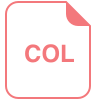
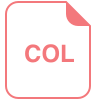
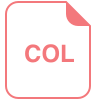
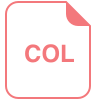
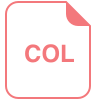