在C#中,从我发布网站的服务器中的一张图片粘贴到另一台服务器的指定文件夹内,并输入另一台服务器的用户密码,代码事例
时间: 2024-09-09 20:14:00 浏览: 67
在C#中,你可以使用`HttpClient`或者`WebRequest`类来下载文件,并结合`Directory.CreateDirectory`和`File.WriteAllText`来复制图片到另一台服务器。由于跨域问题,通常不建议直接粘贴文件,而是下载然后上传。下面是一个基本的例子,假设已知源服务器图片URL、目标服务器地址和文件路径,以及目标服务器的用户名和密码:
```csharp
using System;
using System.IO;
using System.Net.Http;
class Program
{
static void Main(string[] args)
{
try
{
// 定义源图片URL,目标目录及用户名密码
string sourceImageUrl = "http://source-server.com/image.jpg";
string destinationServerAddress = "http://destination-server.com/destination_folder/";
string targetFileName = "image.jpg";
string targetUsername = "username";
string targetPassword = "password";
// 创建HttpClient实例
HttpClient httpClient = new HttpClient();
// 添加基本认证头,注意实际使用时应使用更安全的方式存储密码
httpClient.DefaultRequestHeaders.Authorization =
new System.Net.Http.Headers.AuthenticationHeaderValue("Basic",
Convert.ToBase64String(Encoding.ASCII.GetBytes($"{targetUsername}:{targetPassword}")));
// 下载图片到内存流
HttpResponseMessage response = httpClient.GetAsync(sourceImageUrl).Result;
if (response.IsSuccessStatusCode)
{
byte[] imageBytes = await response.Content.ReadAsByteArrayAsync();
// 将下载的图片保存到临时文件
using (FileStream tempFileStream = File.CreateTempFile("", ".jpg"))
{
tempFileStream.Write(imageBytes, 0, imageBytes.Length);
tempFileStream.Close();
// 构建完整的目标文件路径
string fullTargetPath = $"{destinationServerAddress}{targetFileName}";
// 使用基本HTTP上传到目标服务器
using (var client = new HttpClient())
{
client.DefaultRequestHeaders.Authorization = httpClient.DefaultRequestHeaders.Authorization;
// 这里通常是异步操作,使用await UploadFile(client, fullTargetPath, tempFileStream);
UploadFile(client, fullTargetPath, tempFileStream); // 假设UploadFile是一个自定义函数用于上传文件
Console.WriteLine($"图片成功复制到: {fullTargetPath}");
}
}
}
else
{
Console.WriteLine($"下载失败: {response.StatusCode}");
}
}
catch (Exception ex)
{
Console.WriteLine($"发生错误: {ex.Message}");
}
}
// 假设这是一个模拟的文件上传函数
private static async Task UploadFile(HttpClient client, string fullPath, FileStream fileStream)
{
// 实际上传代码会包含文件名、POST请求等细节,这里仅作示例
var content = new StreamContent(fileStream);
var request = new HttpRequestMessage(HttpMethod.Put, fullPath);
request.Content = content;
await client.SendAsync(request);
}
}
```
请注意,这个例子中存在潜在的安全风险,因为密码是以明文形式存储的。在生产环境中,应使用更安全的方式来管理认证信息,比如OAuth。
阅读全文
相关推荐











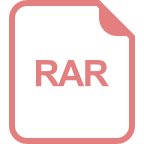





