4组数据分段线性插值c语言,输出分段段曲线函数公式,输出曲线图像
时间: 2024-04-11 21:32:58 浏览: 148
对于数据分段线性插值,我们可以使用C语言来实现。以下是一个示例代码,可以根据输入的数据进行分段线性插值,并输出分段曲线函数的公式和绘制曲线图像。
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#include <stdbool.h>
#include <gd.h>
// 定义数据点结构体
typedef struct {
double x;
double y;
} Point;
// 定义分段线性插值函数
double linearInterpolation(double x, Point p1, Point p2) {
return p1.y + (x - p1.x) * (p2.y - p1.y) / (p2.x - p1.x);
}
// 定义绘制曲线函数
void drawCurve(Point* points, int numPoints) {
// 创建画布
int width = 800;
int height = 600;
gdImagePtr image = gdImageCreate(width, height);
int white = gdImageColorAllocate(image, 255, 255, 255);
int black = gdImageColorAllocate(image, 0, 0, 0);
// 绘制坐标轴
int axisColor = black;
int axisWidth = 2;
gdImageLine(image, 0, height/2, width, height/2, axisColor);
gdImageLine(image, width/2, 0, width/2, height, axisColor);
// 绘制数据点和曲线
int pointColor = gdImageColorAllocate(image, 255, 0, 0);
int curveColor = gdImageColorAllocate(image, 0, 0, 255);
int curveWidth = 2;
for (int i = 0; i < numPoints - 1; i++) {
// 绘制数据点
int x = width/2 + points[i].x * width/10;
int y = height/2 - points[i].y * height/10;
gdImageFilledEllipse(image, x, y, 6, 6, pointColor);
// 绘制曲线
for (int j = x; j < width/2 + points[i+1].x * width/10; j++) {
double interpolatedY = linearInterpolation((j - width/2) / (width/10), points[i], points[i+1]);
int curveX = j;
int curveY = height/2 - interpolatedY * height/10;
gdImageSetPixel(image, curveX, curveY, curveColor);
}
}
// 保存图像到文件
FILE *outputFile = fopen("curve.png", "wb");
gdImagePng(image, outputFile);
fclose(outputFile);
// 释放内存
gdImageDestroy(image);
}
int main() {
int numPoints = 4;
Point points[] = {
{0, 0},
{1, 2},
{3, 6},
{4, 8}
};
// 输出分段曲线函数公式
printf("Curve equations:\n");
for (int i = 0; i < numPoints - 1; i++) {
printf("Segment %d: y = %.2fx + %.2f\n", i+1, (points[i+1].y - points[i].y) / (points[i+1].x - points[i].x), points[i].y - (points[i+1].y - points[i].y) / (points[i+1].x - points[i].x) * points[i].x);
}
// 绘制曲线图像
drawCurve(points, numPoints);
return 0;
}
```
上述代码中,我们先定义了一个 `Point` 结构体来表示数据点的坐标。然后定义了 `linearInterpolation` 函数来进行分段线性插值计算。接下来,我们定义了 `drawCurve` 函数来绘制曲线图像,使用 `gd` 图形库来实现。最后,在 `main` 函数中,我们定义了一组数据点,并调用函数来输出分段曲线函数的公式和绘制曲线图像。
你可以根据需要修改数据点的坐标和个数,运行代码后,会在当前目录生成一个名为 `curve.png` 的曲线图像文件。
阅读全文
相关推荐


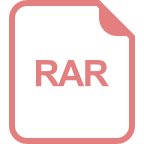
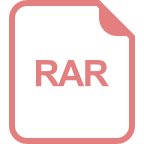
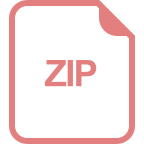
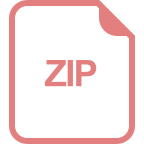
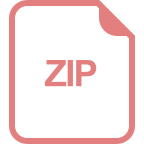
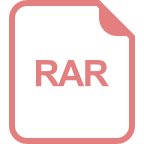
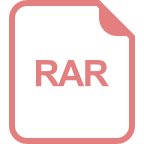
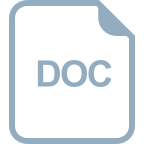
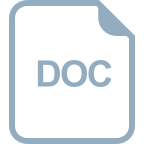
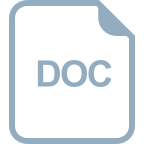
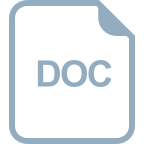
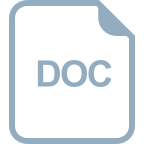
