基于二叉树二叉链表的存储结构定义,编写函数创建一棵二叉树,实现二叉树结点信息的录入,用c语言代码实现
时间: 2024-10-28 17:15:01 浏览: 45
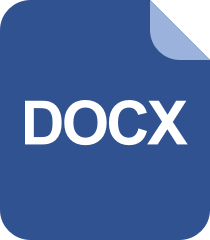
头歌数据结构二叉树的二叉链表存储及基本操作

在C语言中,我们可以首先定义一个简单的二叉树节点结构体,包含一个值(通常是整型或其他类型)、指向左子节点和右子节点的指针。然后,可以编写一个函数来创建二叉树。这里是一个基础的实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树节点结构体
typedef struct TreeNode {
int val; // 节点值
struct TreeNode* left; // 左子节点
struct TreeNode* right; // 右子节点
} TreeNode;
// 创建一个新的二叉树节点
TreeNode* createNode(int data) {
TreeNode* newNode = (TreeNode*)malloc(sizeof(TreeNode));
if (!newNode) {
printf("Memory allocation failed.\n");
return NULL;
}
newNode->val = data;
newNode->left = NULL;
newNode->right = NULL;
return newNode;
}
// 录入二叉树结点信息,输入根节点值
void insertIntoTree(TreeNode** root, int data) {
if (*root == NULL) { // 如果根节点为空,创建新节点并设为根
*root = createNode(data);
} else if (data < (*root)->val) { // 否则递归左子树
insertIntoTree(&(*root)->left, data);
} else { // 如果数据大于根节点,递归右子树
insertIntoTree(&(*root)->right, data);
}
}
int main() {
// 示例:创建一个空二叉树,并插入一些元素
TreeNode* root = NULL;
insertIntoTree(&root, 5); // 插入根节点5
insertIntoTree(&root, 3); // 插入3到左子树
insertIntoTree(&root, 7); // 插入7到右子树
insertIntoTree(&root, 2); // 插入2到左子树
insertIntoTree(&root, 4); // 插入4到左子树
insertIntoTree(&root, 6); // 插入6到右子树
// 打印树的信息
inOrderTraversal(root); // 自己实现中序遍历以打印节点值
return 0;
}
// 中序遍历辅助函数,用于实际打印节点值
void inOrderTraversal(TreeNode* node) {
if (node != NULL) {
inOrderTraversal(node->left);
printf("%d ", node->val); // 输出节点值
inOrderTraversal(node->right);
}
}
```
这个例子中,`createNode`函数负责创建新的节点,`insertIntoTree`函数负责将给定的数据插入到二叉树的适当位置。`main`函数展示了如何创建一个二叉树并插入节点。
阅读全文
相关推荐
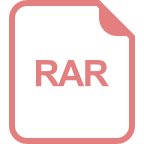
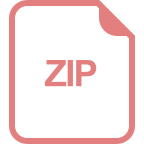















