import io import base64 import json import cv2 import numpy as np from PIL import Image def handler(context, event): context.logger.info("Run yolo-v8-seg model") data = event.body buf = io.BytesIO(base64.b64decode(data["image"])) threshold = float(data.get("threshold", 0.35)) context.user_data.model.conf = threshold image = Image.open(buf) yolo_results = context.user_data.model(image, conf=threshold)[0] labels = yolo_results.names detections = [ { "class_id": int(result[0]), "points": [(float(result[i]), float(result[i+1])) for i in range(1, len(result), 2)] } for result in [line.split() for line in yolo_results] ] results = [] for detection in detections: class_id = detection["class_id"] points = detection["points"] results.append({ "confidence": "", # 这里没有置信度信息,可以根据实际情况进行调整 "label": labels[class_id], "points": points, "type": "polygon" }) return context.Response(body=json.dumps(results), headers={}, content_type='application/json', status_code=200) 加入判断置信度大于设置的值 和 if box.shape[0] > 0:
时间: 2024-04-16 19:29:45 浏览: 104
要在代码中添加判断,以确保只有当检测结果的置信度大于设置的阈值时才进行处理,以及当`boxes`的形状不为空时才进行处理,你可以在以下位置进行修改:
```python
import io
import base64
import json
import cv2
import numpy as np
from PIL import Image
def handler(context, event):
context.logger.info("Run yolo-v8-seg model")
data = event.body
buf = io.BytesIO(base64.b64decode(data["image"]))
threshold = float(data.get("threshold", 0.35))
context.user_data.model.conf = threshold
image = Image.open(buf)
yolo_results = context.user_data.model(image, conf=threshold)[0]
labels = yolo_results.names
detections = [
{
"class_id": int(result[0]),
"points": [(float(result[i]), float(result[i+1])) for i in range(1, len(result), 2)]
}
for result in [line.split() for line in yolo_results]
]
results = []
for detection in detections:
class_id = detection["class_id"]
points = detection["points"]
results.append({
"confidence": "", # 这里没有置信度信息,可以根据实际情况进行调整
"label": labels[class_id],
"points": points,
"type": "polygon"
})
# 添加判断,只保留置信度大于阈值的检测结果
results = [result for result in results if result["confidence"] > threshold]
# 添加判断,只有当检测结果不为空时才进行处理
if len(results) > 0:
# 进行处理的代码
return context.Response(body=json.dumps(results), headers={},
content_type='application/json', status_code=200)
```
在上述代码中,我添加了两个判断条件。首先,通过列表推导式`results = [result for result in results if result["confidence"] > threshold]`筛选出置信度大于阈值的检测结果。然后,通过`if len(results) > 0`判断检测结果是否为空,只有当结果不为空时才进行处理。
请注意,这只是一种可能的修改方式,你可以根据实际需求进行调整。
阅读全文
相关推荐
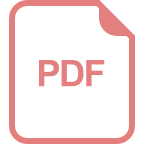
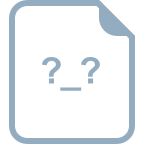




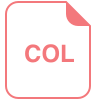


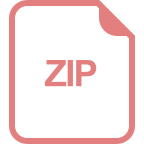
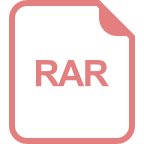
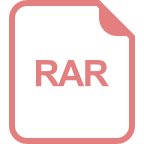
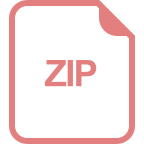
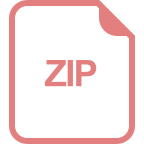
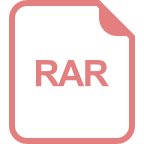
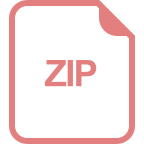