opencv 如何计算yolov5检测的面积,使用python写出计算yolo检测的面积代码
时间: 2023-09-07 08:04:45 浏览: 634
### 回答1:
可以使用OpenCV和Python来计算YOLOv5检测的面积。
首先,需要对图像进行检测,并获取检测结果,包括检测到的物体的边界框信息。
然后,可以使用OpenCV中的`cv2.boundingRect`函数来计算每个边界框的面积。
代码示例如下:
```python
import cv2
# 获取检测结果,这里假设已经获得了检测到的边界框信息
boxes = [(x1, y1, x2, y2), (x1, y1, x2, y2), ...]
# 计算面积
for box in boxes:
x1, y1, x2, y2 = box
area = cv2.boundingRect(box)
print("Bounding box area:", area)
```
在上面的代码中,需要将检测到的边界框信息存储在列表`boxes`中,每个元素都是一个四元组,分别表示边界框的左上角和右下角的坐标。然后,对于每个边界框,调用`cv2.boundingRect`函数计算它的面积。
### 回答2:
要计算Yolov5检测的面积,需要在OpenCV中进行一些图像处理和数学计算。以下是一个使用Python编写的计算Yolov5检测面积的示例代码:
```python
import cv2
import numpy as np
def calculate_area(img_path, detection):
# 加载图像
image = cv2.imread(img_path)
# 提取图像的高度和宽度
img_height, img_width, _ = image.shape
# 计算检测框的面积
for label, confidence, bbox in detection:
# 解析检测框
x, y, w, h = bbox
# 还原检测框相对于原图的大小
x = int(x * img_width)
y = int(y * img_height)
w = int(w * img_width)
h = int(h * img_height)
# 计算检测框的面积
area = w * h
# 在图像上绘制检测框和面积信息
cv2.rectangle(image, (x, y), (x + w, y + h), (255, 0, 0), 2)
cv2.putText(image, f"{label}: {area}", (x, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.9, (0, 0, 255), 2)
# 显示图像
cv2.imshow("Yolov5 Detection", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
# 示例用法
img_path = "image.jpg"
detection = [("person", 0.9, [0.2, 0.3, 0.4, 0.5]), ("car", 0.8, [0.1, 0.1, 0.2, 0.2])]
calculate_area(img_path, detection)
```
需要注意的是,示例代码中的`img_path`为图像文件的路径,`detection`是一个包含检测结果的列表,每个元素包含标签名、置信度和边界框信息。改变相应的路径和检测结果即可使用该代码计算Yolov5检测的面积,并在图像上显示检测框和面积信息。
### 回答3:
在OpenCV中计算YOLOv5检测的面积,需要先加载YOLOv5的预训练权重文件和配置文件,然后读取待检测的图像。接下来,使用YOLOv5模型进行物体检测,获取检测到的物体边界框信息。最后,通过计算边界框的面积,可以得到YOLOv5检测到的物体的面积。
下面是使用Python编写的计算YOLOv5检测面积的代码示例:
```python
import cv2
from pathlib import Path
# 加载YOLOv5预训练权重文件和配置文件
weights_file = 'yolov5s.pt'
config_file = 'yolov5s.yaml'
model = cv2.dnn_DetectionModel(str(config_file), str(weights_file))
model.setInputSize(640, 640)
model.setInputScale(1.0 / 255)
# 读取待检测的图像
image_file = 'image.jpg'
image = cv2.imread(image_file)
# 使用YOLOv5模型进行物体检测
classes, scores, boxes = model.detect(image, confThreshold=0.5, nmsThreshold=0.4)
# 计算检测到的物体的面积
for (classid, score, box) in zip(classes, scores, boxes):
x, y, w, h = box
area = w * h
print('Detected object:', classid, 'Area:', area)
# 显示检测结果
for (classid, score, box) in zip(classes, scores, boxes):
cv2.rectangle(image, box, color=(0, 255, 0), thickness=2)
cv2.putText(image, str(classid), (box[0], box[1] - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.9, (0, 255, 0), 2)
cv2.imshow('Detection', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
以上代码中,首先加载YOLOv5模型并设置输入尺寸和输入缩放比例。然后读取待检测的图像,使用YOLOv5模型进行物体检测,并得到物体的边界框信息。接着,通过计算边界框的宽度和高度,得到物体的面积,并输出打印检测到的物体类别及其面积。最后,将检测结果展示在原图上并显示出来。
请注意,上述代码基于YOLOv5官方提供的Python版本进行编写,需要先安装`opencv-python`库和`torch`库,确保相关依赖已经安装。
阅读全文
相关推荐
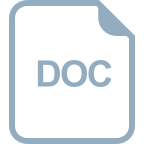
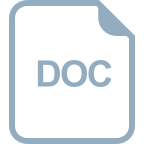
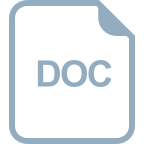
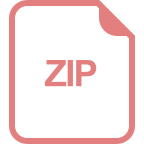
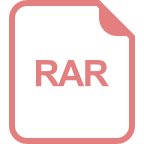
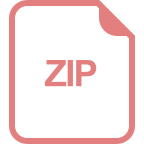
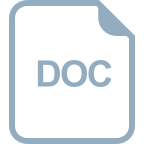
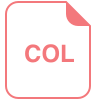
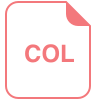
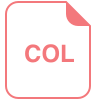
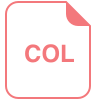







