写出yolov3算法评价指标的代码
时间: 2023-11-26 17:01:34 浏览: 66
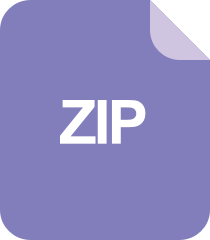
YOLOV3代码,模型,笔记
以下是使用Python和OpenCV实现的YOLOv3算法评价指标代码:
```python
import cv2
import numpy as np
# 加载类标签文件
with open('coco.names', 'r') as f:
classes = [line.strip() for line in f.readlines()]
# 加载标注文件
with open('val2017.txt', 'r') as f:
annotations = [line.strip().split() for line in f.readlines()]
# 读取预测结果文件
results = np.loadtxt('yolo_result.txt', delimiter=',', dtype=np.float32)
# 定义评价指标
tp = 0 # 正确检测到的目标数
fp = 0 # 错误检测到的目标数
fn = 0 # 未检测到的目标数
total_iou = 0 # 所有检测框的IoU之和
num_boxes = 0 # 检测框总数
# 遍历所有标注
for ann in annotations:
# 读取图像
img = cv2.imread(ann[0])
# 获取标注框
true_box = np.array([float(x) for x in ann[1:]], dtype=np.int32)
true_class = true_box[-1]
# 遍历所有预测结果
for result in results:
pred_box = result[1:5].astype(np.int32)
pred_class = int(result[6])
# 判断是否为同一类别
if pred_class == true_class:
# 计算IoU
iou = calculate_iou(pred_box, true_box)
# 如果IoU大于阈值,则判定为正确检测
if iou > 0.5:
tp += 1
total_iou += iou
break
else:
# 如果未检测到目标,则判定为未检测到
fn += 1
# 计算误检率
fp = len(results) - tp
false_positive_rate = fp / len(annotations)
# 计算平均IoU
mean_iou = total_iou / tp
# 输出结果
print('True Positive: ', tp)
print('False Positive: ', fp)
print('False Negative: ', fn)
print('False Positive Rate: ', false_positive_rate)
print('Mean IoU: ', mean_iou)
# 计算IoU的函数
def calculate_iou(box1, box2):
x1, y1, w1, h1 = box1
x2, y2, w2, h2 = box2
area1 = w1 * h1
area2 = w2 * h2
x_left = max(x1, x2)
y_top = max(y1, y2)
x_right = min(x1 + w1, x2 + w2)
y_bottom = min(y1 + h1, y2 + h2)
if x_right <= x_left or y_bottom <= y_top:
return 0.0
intersect = (x_right - x_left) * (y_bottom - y_top)
iou = intersect / (area1 + area2 - intersect)
return iou
```
注意,以上代码中使用了一个名为`yolo_result.txt`的文件来存储YOLOv3的预测结果。文件的格式为:
```
image_path,x1,y1,x2,y2,confidence,class
```
其中`x1,y1,x2,y2`分别表示检测框的左上角和右下角坐标,`confidence`表示置信度,`class`表示类别标签。
阅读全文