def fun(args): a=args f=lambda x:x[0]**2*(a+x[1]**2)+x[1]*np.log(x[1]) return f
时间: 2023-11-06 14:07:37 浏览: 33
(args)
There seems to be a syntax error in the function definition. Here's a corrected version of the function:
import numpy as np
def fun(args):
a = args
f = lambda x: x[0]**2*(a*x[1]**2)*x[1]*np.log(x[1])
return f
相关问题
what is wrong with my codes def objective_fun(x, alpha,p): return -1*(x[0]**alpha)*(x[1]**(1-alpha)) p = [5,10] alpha = 0.3 args = (p, alpha) x=[5,10] cons={"type": "ineq", "fun": lambda x,p: 100 - p[0]*x[0] - p[1]*x[1], "args": (p,)} result= minimize(objective_fun,x,bounds=[(0,None), (0,None)], args=args, constraints=cons)
在这段代码中,存在一个问题:`objective_fun`函数中的参数`p`没有传递给`minimize`函数。为了将`p`传递给`objective_fun`函数,您需要将`args`参数的值从`(p, alpha)`改为`(alpha, p)`。这样,`alpha`将作为第一个参数传递给`objective_fun`函数,而`p`将作为第二个参数传递给`objective_fun`函数。修改后的代码如下:
```
def objective_fun(x, alpha, p):
return -1*(x[0]**alpha)*(x[1]**(1-alpha))
p = [5,10]
alpha = 0.3
args = (alpha, p)
x = [5,10]
cons = {"type": "ineq", "fun": lambda x, p: 100 - p[0]*x[0] - p[1]*x[1], "args": (p,)}
result = minimize(objective_fun, x, bounds=[(0,None), (0,None)], args=args, constraints=cons)
```
请注意,`cons`参数中的`lambda`函数也需要更新为使用`p`参数而不是`args`参数。
import numpy as np from scipy.optimize import minimize # 定义目标函数 def objective(x): return (x[0] - 2)**4 + (x[0] - 2*x[1])**2 # 定义约束条件 def constraint(x): return x[0]**2 - x[1] # 定义拉格朗日函数 def lagrangian(x, lambda_): return objective(x) + lambda_ * max(0, constraint(x)) # 定义拉格朗日函数的梯度 def lagrangian_gradient(x, lambda_): return np.array([4 * (x[0] - 2)**3 + 2 * (x[0]-2 * x[1]) + lambda_, -4 * (x[0] - 2 * x[1]) + lambda_]) # 定义约束条件的梯度 def constraint_gradient(x,lambda_,p): return np.array([2*x[0], 1]) # 定义初始点和初始拉格朗日乘子 x0 = np.array([2, 1]) lambda0 = 0 # 定义迭代过程记录列表 iteration_history = [] # 定义迭代回调函数 def callback(xk): iteration_history.append(xk) # 使用牛顿拉格朗日法求解优化问题 result = minimize(lagrangian, x0, args=(lambda0,), method='Newton-CG', jac=lagrangian_gradient, hessp=lambda x,lambda_,p:np.dot(constraint_gradient(x,lambda_,p),p), callback=callback) # 输出结果 print('拟合结果:') print('最优解:', result.x) print('目标函数值:', result.fun) print('约束条件:', constraint(result.x)) # 绘制迭代过程图 import matplotlib.pyplot as plt iteration_history = np.array(iteration_history) plt.plot(iteration_history[:, 0], iteration_history[:, 1], marker='o') plt.xlabel('x1') plt.ylabel('x2') plt.title('Iteration History') plt.show()
这段代码使用了牛顿拉格朗日法来求解一个带约束条件的优化问题,其中目标函数为 $(x_1-2)^4+(x_1-2x_2)^2$,约束条件为 $x_1^2-x_2\leq 0$。具体来说,代码中定义了目标函数、约束条件、拉格朗日函数、拉格朗日函数的梯度、约束条件的梯度等函数,并使用 `minimize` 函数来求解优化问题。在迭代过程中,使用回调函数记录迭代点,最后绘制迭代过程图。
相关推荐
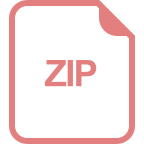
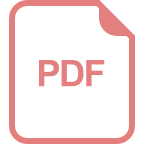












