cartopy.crs如何设置地图显示经度范围为跨180度经线从100度到-100度,纬度从10到50度
时间: 2024-10-29 10:20:34 浏览: 105
在Cartopy库中,`cartopy.crs`模块用于处理地理坐标系统( projections)。如果你想要创建一个跨180度经线、纬度在10到50度的地图,你可以使用Mercator投影或者Mollweide投影,并对经纬度轴进行适当的设置。这里是一个示例:
```python
import cartopy.crs as ccrs
import matplotlib.pyplot as plt
# 设置地图范围
central_longitude = 90 # Mercator中心经度,可以是100度或者-100度
central_latitude = 30 # 纬度范围中间点
min_latitude = 10
max_latitude = 50
fig = plt.figure(figsize=(10, 6))
ax = fig.add_subplot(1, 1, 1, projection=ccrs.Mercator(central_longitude))
# 调整经度范围跨越180度
ax.set_global()
ax.extend_domain(crs.PlateCarree(), start_longitude=-180, end_longitude=180)
# 设置纬度范围
ax.set_extent([-180, 180, min_latitude, max_latitude], crs=ccrs.PlateCarree())
plt.show()
相关问题
如何使用Python编程语言,基于WGS84坐标系和给定的经度范围(0到359.9度,分辨率0.1度)以及纬度范围(90.0到-90.0度,同样分辨率0.1度),准确计算每个网格的地球表面面积?
在Python中,我们可以使用地理空间库如`geopandas`或`rasterio`来处理经纬度范围并计算每个网格的地球表面面积。这里我们假设你想要生成的是一个个正方形的网格,而不是地球曲面上的实际经纬度间距。以下是使用`geopandas`的一个基本步骤:
首先,安装必要的库:
```bash
pip install geopandas rasterio
```
然后,创建网格函数:
```python
import numpy as np
from shapely.geometry import Polygon
from geopandas import GeoDataFrame
def create_grids(longitude_range, latitude_range, resolution):
# 创建经度和纬度数组
lon = np.arange(longitude_range[0], longitude_range[1] + resolution, resolution)
lat = np.arange(latitude_range[0], latitude_range[1] + resolution, resolution)
# 经纬度转换成二维网格
longitudes, latitudes = np.meshgrid(lon, lat)
# 将经纬度转换为GeoSeries
geometry = [Polygon([(lon[i][j], lat[i][j]) for j in range(len(lat[i]))]) for i in range(len(lon))]
# 创建GeoDataFrame
grids = GeoDataFrame({'geometry': geometry}, crs={'init': 'epsg:4326'}) # WGS84坐标系
return grids
# 定义经纬度和分辨率
longitude_range = (0, 360) # 注意0到360度是为了包含360度经线
latitude_range = (-90, 90)
resolution = 0.1
grids = create_grids(longitude_range, latitude_range, resolution)
```
接下来,你需要计算每个网格的面积。由于`geopandas`已经将几何对象设置为`Polygon`,可以直接调用其`area`属性:
```python
areas = grids['geometry'].apply(lambda x: x.area)
grids['area'] = areas
```
现在,`grids` DataFrame包含了每个网格的经度、纬度和对应的地球表面面积。
注意:上述代码仅适用于近似计算,并未考虑地球的真实形状(球体或椭球体),对于精确计算,你需要使用大地测量学的公式,或者利用专门的地表覆盖数据(如DEM数据)进行计算。
ccrs.PlateCarree()如何移动中心经度
在使用Cartopy的PlateCarree坐标系(ccrs.PlateCarree())时,它的默认中心位置是全球中央(即0°经度、0°纬度),如果你想更改中心经度,你可以创建一个新的 PlateCarree projection 实例并指定新的中心经纬度作为`central_longitude`参数。
以下是一个例子:
```python
import matplotlib.pyplot as plt
import cartopy.crs as ccrs
# 定义新的中心经度,例如东半球的中心(约75°E)
central_longitude = 75
# 创建新的PlateCarree实例,并设置中心经度
custom_projection = ccrs.PlateCarree(central_longitude=central_longitude)
fig, ax = plt.subplots(subplot_kw={'projection': custom_projection})
# 绘制地图,中心经线会自动对齐到新的中心位置
ax.stock_img()
# 如果需要添加经纬度格网,也需要相应调整
gl = ax.gridlines(draw_labels=True, crs=custom_projection,
xlabels_top=False, ylabels_right=False,
linestyle=':', linewidth=0.5, color='lightgrey',
alpha=0.5)
# 可能还需要调整其他地图元素的位置,比如标题和标注
ax.set_global()
plt.title(f"Centered at {central_longitude:.1f}°E")
plt.show()
```
在这个例子中,地图的中心经线会被设置为你指定的值,同时地图上的其他元素如经纬度标签也会相对于新的中心位置调整。
阅读全文
相关推荐
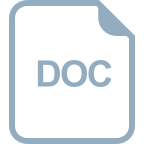
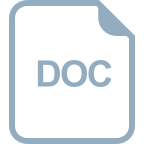
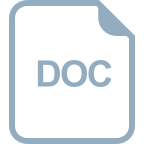
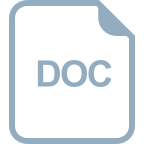
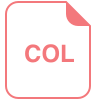
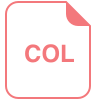
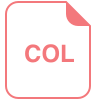
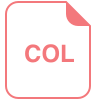
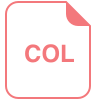
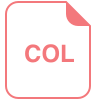

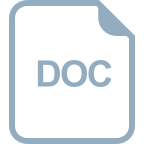
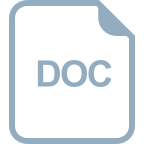
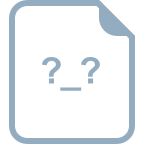
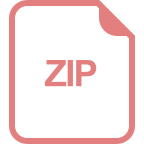
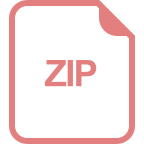
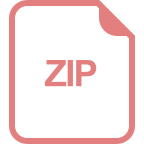