如何使用Python做一个四车道十字路口模拟图
时间: 2024-04-29 11:04:10 浏览: 14
要使用Python做一个四车道十字路口模拟图,可以使用Python的图形库Tkinter来实现。下面是一个简单的例子:
```python
from tkinter import *
import time
class Car:
def __init__(self, x, y, speed, color, direction):
self.x = x
self.y = y
self.speed = speed
self.color = color
self.direction = direction
def move(self):
if self.direction == "up":
self.y -= self.speed
elif self.direction == "down":
self.y += self.speed
elif self.direction == "left":
self.x -= self.speed
elif self.direction == "right":
self.x += self.speed
class Intersection:
def __init__(self):
self.cars = []
self.width = 600
self.height = 600
self.window = Tk()
self.canvas = Canvas(self.window, width=self.width, height=self.height, bg="white")
self.canvas.pack()
self.draw_roads()
self.window.after(0, self.run)
self.window.mainloop()
def draw_roads(self):
self.canvas.create_line(0, self.height/2, self.width, self.height/2, width=5)
self.canvas.create_line(self.width/2, 0, self.width/2, self.height, width=5)
def run(self):
self.move_cars()
self.draw_cars()
self.window.after(50, self.run)
def move_cars(self):
for car in self.cars:
car.move()
if car.direction == "up" and car.y < -50:
self.cars.remove(car)
elif car.direction == "down" and car.y > self.height + 50:
self.cars.remove(car)
elif car.direction == "left" and car.x < -50:
self.cars.remove(car)
elif car.direction == "right" and car.x > self.width + 50:
self.cars.remove(car)
def draw_cars(self):
self.canvas.delete("car")
for car in self.cars:
x1 = car.x - 20
y1 = car.y - 20
x2 = car.x + 20
y2 = car.y + 20
self.canvas.create_rectangle(x1, y1, x2, y2, fill=car.color, tags="car")
def add_car(self, direction):
if direction == "up":
x = self.width/2
y = self.height + 50
speed = 5
color = "blue"
elif direction == "down":
x = self.width/2
y = -50
speed = 5
color = "red"
elif direction == "left":
x = self.width + 50
y = self.height/2
speed = 5
color = "green"
elif direction == "right":
x = -50
y = self.height/2
speed = 5
color = "yellow"
car = Car(x, y, speed, color, direction)
self.cars.append(car)
if __name__ == "__main__":
intersection = Intersection()
```
这个例子中,我们定义了两个类:`Car`和`Intersection`。`Car`类表示一辆车,包括其位置、速度、颜色和运动方向。`Intersection`类表示一个十字路口模拟图,包括所有的车辆和绘制界面的函数。
在`Intersection`类中,我们使用Tkinter库创建了一个窗口和一个画布。在画布上绘制了四条道路,分别表示四个方向的车道。然后定义了一个`run`方法,用来重复执行`move_cars`和`draw_cars`方法,实现车辆的运动和绘制。
在`add_car`方法中,我们可以添加新的车辆,指定其运动方向和颜色。在`move`方法中,我们根据车辆的方向和速度更新其位置。如果车辆超出画布范围,我们将其从车辆列表中删除。
最后,在`if __name__ == "__main__":`语句中创建一个`Intersection`对象,即可运行程序。可以通过调用`add_car`方法来添加新的车辆。
相关推荐
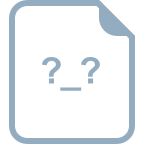
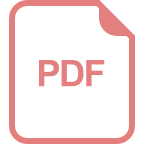














