用Java制作一个贪吃蛇游戏
时间: 2024-05-05 11:21:44 浏览: 97
好的,下面我给你提供一份简单的 Java 贪吃蛇游戏代码,希望能帮助到你:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.util.Random;
public class SnakeGame extends JFrame implements ActionListener {
private final int SIZE = 20;
private final int WIDTH = 20;
private final int HEIGHT = 20;
private final int DOT_SIZE = 10;
private final int ALL_DOTS = 900;
private int[] x = new int[ALL_DOTS];
private int[] y = new int[ALL_DOTS];
private int dots;
private int apple_x;
private int apple_y;
private boolean leftDirection = false;
private boolean rightDirection = true;
private boolean upDirection = false;
private boolean downDirection = false;
private boolean inGame = true;
private Timer timer;
public SnakeGame() {
setTitle("贪吃蛇游戏");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(SIZE * WIDTH, SIZE * HEIGHT);
setResizable(false);
setLocationRelativeTo(null);
setVisible(true);
addKeyListener(new TAdapter());
initGame();
}
public void initGame() {
dots = 3;
for (int i = 0; i < dots; i++) {
x[i] = 50 - i * 10;
y[i] = 50;
}
locateApple();
timer = new Timer(140, this);
timer.start();
}
public void checkApple() {
if ((x[0] == apple_x) && (y[0] == apple_y)) {
dots++;
locateApple();
}
}
public void locateApple() {
int r = new Random().nextInt(SIZE);
apple_x = r * DOT_SIZE;
r = new Random().nextInt(SIZE);
apple_y = r * DOT_SIZE;
}
public void checkCollision() {
for (int i = dots; i > 0; i--) {
if ((i > 4) && (x[0] == x[i]) && (y[0] == y[i])) {
inGame = false;
}
}
if (y[0] >= HEIGHT * DOT_SIZE) {
inGame = false;
}
if (y[0] < 0) {
inGame = false;
}
if (x[0] >= WIDTH * DOT_SIZE) {
inGame = false;
}
if (x[0] < 0) {
inGame = false;
}
if (!inGame) {
timer.stop();
}
}
public void move() {
for (int i = dots; i > 0; i--) {
x[i] = x[i - 1];
y[i] = y[i - 1];
}
if (leftDirection) {
x[0] -= DOT_SIZE;
}
if (rightDirection) {
x[0] += DOT_SIZE;
}
if (upDirection) {
y[0] -= DOT_SIZE;
}
if (downDirection) {
y[0] += DOT_SIZE;
}
}
public void paint(Graphics g) {
super.paint(g);
if (inGame) {
g.setColor(Color.red);
g.fillOval(apple_x, apple_y, DOT_SIZE, DOT_SIZE);
for (int i = 0; i < dots; i++) {
if (i == 0) {
g.setColor(Color.green);
g.fillRect(x[i], y[i], DOT_SIZE, DOT_SIZE);
} else {
g.setColor(Color.yellow);
g.fillRect(x[i], y[i], DOT_SIZE, DOT_SIZE);
}
}
Toolkit.getDefaultToolkit().sync();
} else {
gameOver(g);
}
}
public void gameOver(Graphics g) {
String msg = "Game Over";
Font small = new Font("Helvetica", Font.BOLD, 14);
FontMetrics metr = getFontMetrics(small);
g.setColor(Color.white);
g.setFont(small);
g.drawString(msg, (WIDTH * SIZE - metr.stringWidth(msg)) / 2, HEIGHT * SIZE / 2);
}
public void actionPerformed(ActionEvent e) {
if (inGame) {
checkApple();
checkCollision();
move();
}
repaint();
}
private class TAdapter extends KeyAdapter {
public void keyPressed(KeyEvent e) {
int key = e.getKeyCode();
if ((key == KeyEvent.VK_LEFT) && (!rightDirection)) {
leftDirection = true;
upDirection = false;
downDirection = false;
}
if ((key == KeyEvent.VK_RIGHT) && (!leftDirection)) {
rightDirection = true;
upDirection = false;
downDirection = false;
}
if ((key == KeyEvent.VK_UP) && (!downDirection)) {
upDirection = true;
rightDirection = false;
leftDirection = false;
}
if ((key == KeyEvent.VK_DOWN) && (!upDirection)) {
downDirection = true;
rightDirection = false;
leftDirection = false;
}
}
}
public static void main(String[] args) {
new SnakeGame();
}
}
```
这份代码中,我们使用了 Java 的 Swing 图形库来实现 GUI 界面,使用了计时器来控制游戏的刷新频率。在游戏中,贪吃蛇的身体由一系列方格组成,我们定义了四个方向变量来控制贪吃蛇的移动方向,使用键盘监听器来监听用户输入,实现贪吃蛇的控制。同时,我们在游戏中添加了随机生成苹果的功能,当贪吃蛇吃到苹果时,贪吃蛇的身体长度会增加。在游戏过程中,我们对贪吃蛇与边界以及自身碰撞进行了检测,当贪吃蛇出现碰撞时,游戏结束,弹出游戏结束的提示框。
阅读全文
相关推荐

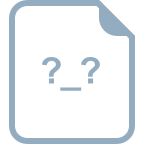
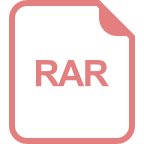
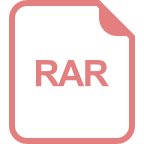
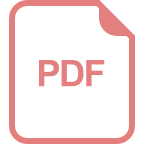
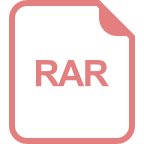
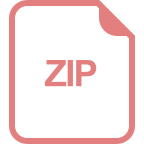
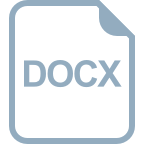
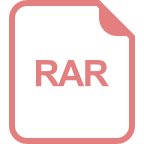
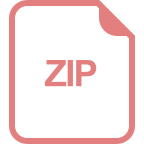
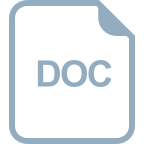
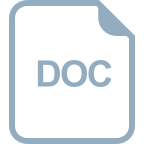
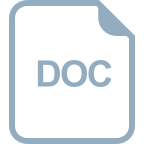

