实现父进程创建一个子进程,父进程通过无名管道向子进程发送数据“This is parent’s Message!\n”。子进程接收到信息后,输出接收到的数据,延迟2s(sleep(2)),然后向父进程发送数据“Child has received Message!\n”,然后退出,当父进程也接收到数据后也打印输出,并退出。
时间: 2024-02-12 11:02:45 浏览: 55
好的,下面是代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#define MAX_BUF_SIZE 1024
int main()
{
int pipe_fd[2];
pid_t pid;
char parent_buf[MAX_BUF_SIZE] = "This is parent's message!";
char child_buf[MAX_BUF_SIZE] = "Child has received message!";
if (pipe(pipe_fd) < 0) {
perror("pipe error");
exit(1);
}
if ((pid = fork()) < 0) {
perror("fork error");
exit(1);
}
if (pid > 0) {
// 父进程
close(pipe_fd[0]); // 关闭读端
printf("Parent: send message to child\n");
write(pipe_fd[1], parent_buf, strlen(parent_buf) + 1);
close(pipe_fd[1]); // 关闭写端
printf("Parent: waiting for child's response\n");
char child_response[MAX_BUF_SIZE];
read(pipe_fd[0], child_response, sizeof(child_response));
printf("Parent: receive message from child: %s\n", child_response);
close(pipe_fd[0]); // 关闭读端
} else if (pid == 0) {
// 子进程
close(pipe_fd[1]); // 关闭写端
char parent_message[MAX_BUF_SIZE];
read(pipe_fd[0], parent_message, sizeof(parent_message));
printf("Child: receive message from parent: %s\n", parent_message);
sleep(2); // 延迟2秒
printf("Child: send response to parent\n");
write(pipe_fd[1], child_buf, strlen(child_buf) + 1);
close(pipe_fd[0]); // 关闭读端
}
return 0;
}
```
在这个代码中,首先创建了一个无名管道。然后,使用 fork() 函数创建了一个子进程。在父进程中,向管道中写入了一条消息,并等待子进程的回应。在子进程中,从管道中读取了父进程发送的消息,并打印输出,然后延迟2秒,并向父进程发送了一条消息。最后,父进程再次从管道中读取了子进程发送的消息,并打印输出。
需要注意的是,管道是一种半双工的通信方式,即同一时间只能进行一种通信,如果需要双向通信,需要创建两个管道。此外,写入数据时,需要使用 write() 函数,读取数据时,需要使用 read() 函数。
希望这个回答能够帮到你。如果还有不清楚的地方,欢迎继续提问。
阅读全文
相关推荐
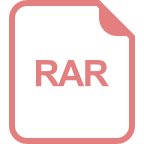
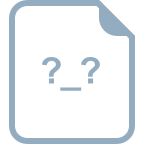
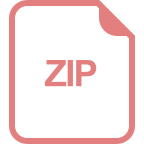
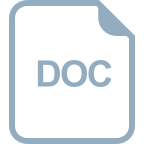
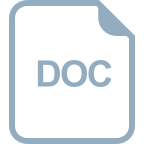
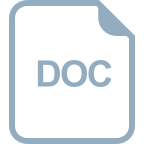
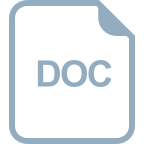
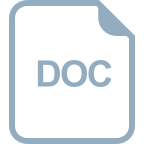







