怎么将训练好的模型加载到Jetson Nano上,并使用OpenCV来处理实时视频流
时间: 2023-05-26 10:06:03 浏览: 131
要将训练好的模型加载到Jetson Nano上并使用OpenCV处理实时视频流,您需要遵循以下步骤:
1. 将训练后的模型拷贝到Jetson Nano上,可以通过SCP或者FTP等传输工具来完成。
2. 在Jetson Nano上安装必要的库和工具包,如OpenCV等。可以使用以下命令安装:
```
sudo apt-get install python3-opencv
```
3. 在Python代码中加载模型文件,并使用OpenCV来实时处理视频流。以下是一个示例代码,可以替换为您自己的模型文件和数据流来源。
```
import cv2
import numpy as np
# Load the trained model
model = cv2.dnn.readNetFromCaffe('model.prototxt', 'model.caffemodel')
# Open the video stream
cap = cv2.VideoCapture(0)
while True:
# Read a frame from the video stream
ret, frame = cap.read()
# Preprocess the input frame
blob = cv2.dnn.blobFromImage(frame, scalefactor=1.0, size=(300, 300), mean=(104.0, 177.0, 123.0))
# Set the input to the model
model.setInput(blob)
# Forward pass through the model
detections = model.forward()
# Postprocess the detections
for i in range(detections.shape[2]):
confidence = detections[0, 0, i, 2]
if confidence > 0.5:
box = detections[0, 0, i, 3:7] * np.array([frame.shape[1], frame.shape[0], frame.shape[1], frame.shape[0]])
box = box.astype('int')
cv2.rectangle(frame, (box[0], box[1]), (box[2], box[3]), (0, 255, 0), thickness=2)
# Display the output frame
cv2.imshow('Output', frame)
# Exit on 'q' key press
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# Cleanup the resources
cap.release()
cv2.destroyAllWindows()
```
上面的代码中,首先加载了模型文件,然后打开了实时视频流,并在每个帧上进行前向传递,并进行检测后显示输出帧。最后,通过按下‘q'键退出循环并清理资源。
可以根据需要更改模型文件和其他参数来适应不同的任务和数据源。
相关推荐
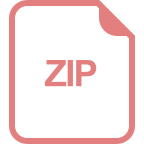
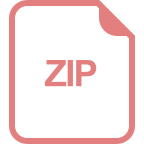
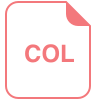
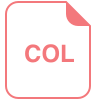
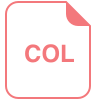











