char* get() { char p[] = "Hello world!" return p; } void main() { printf(“%s\n”,get()); }
时间: 2024-05-08 19:20:45 浏览: 16
这段代码有一个问题,函数`get`返回的是一个指向局部变量`p`的指针,而`p`是在函数内部定义的,当函数执行完毕后,`p`所占用的内存会被释放,这意味着指向`p`的指针将不再指向有效的内存地址。因此,当`main`函数调用`get`函数并尝试打印`p`指向的字符串时,很可能会出现未定义的行为和错误结果。为了避免这种问题,你可以将`p`定义为静态变量或者使用动态内存分配函数`malloc`来分配内存。比如,下面是一个修改后的代码示例:
```
char* get() {
static char p[] = "Hello world!";
return p;
}
int main() {
printf("%s\n", get());
return 0;
}
```
或者使用动态内存分配函数`malloc`分配内存,示例代码如下:
```
char* get() {
char* p = (char*) malloc(sizeof(char) * 13);
strcpy(p, "Hello world!");
return p;
}
int main() {
printf("%s\n", get());
free(get()); // 释放内存
return 0;
}
```
相关问题
用C语言实现试采用KMP算法编写程序,在串s中找到串p第一次出现的位置
以下是用C语言实现KMP算法的程序,用于在串s中找到串p第一次出现的位置。
```c
#include <stdio.h>
#include <string.h>
void get_next(char *p, int *next) {
int i = 0, j = -1;
next[0] = -1;
while (p[i]) {
if (j == -1 || p[i] == p[j]) {
i++;
j++;
next[i] = j;
} else {
j = next[j];
}
}
}
int kmp(char *s, char *p) {
int i = 0, j = 0;
int slen = strlen(s);
int plen = strlen(p);
int next[plen];
get_next(p, next);
while (i < slen && j < plen) {
if (j == -1 || s[i] == p[j]) {
i++;
j++;
} else {
j = next[j];
}
}
if (j == plen) {
return i - j;
} else {
return -1;
}
}
int main() {
char s[] = "hello world";
char p[] = "world";
int pos = kmp(s, p);
if (pos == -1) {
printf("Not found\n");
} else {
printf("Found at position %d\n", pos);
}
return 0;
}
```
我们首先实现一个 `get_next` 函数,用于生成模式串 `p` 的 next 数组。在主函数中调用 `kmp` 函数,使用双指针 `i` 和 `j` 分别指向字符串 `s` 和 `p` 的头部,从前往后匹配字符串。如果字符匹配成功,则将双指针同时后移;否则将模式串 `p` 的指针 `j` 按照 next 数组的值进行回溯,将匹配指针 `i` 不变。如果匹配指针 `j` 移到了模式串的头部,说明匹配成功,返回匹配位置;否则匹配失败,返回 -1。
基于ebpf写一个hello_world
以下是基于eBPF写一个hello_world的步骤:
1.安装依赖项和工具链
```shell
sudo apt-get update
sudo apt-get install -y build-essential linux-headers-$(uname -r) libelf-dev clang llvm
```
2.创建一个名为helloworld.bpf.c的文件,并将以下代码复制到文件中:
```c
#include <linux/bpf.h>
#include <linux/version.h>
#include <stddef.h>
#include <stdint.h>
char _license[] SEC("license") = "GPL";
int _version SEC("version") = LINUX_VERSION_CODE;
SEC("kprobe/sys_clone")
int bpf_prog(void *ctx)
{
char msg[] = "Hello, World!";
bpf_trace_printk(msg, sizeof(msg));
return 0;
}
```
3.创建一个名为helloworld.c的文件,并将以下代码复制到文件中:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <linux/bpf.h>
#include <bpf/libbpf.h>
int main(int argc, char **argv)
{
struct bpf_object *obj; int prog_fd, err;
/* Load the BPF object file */
err = bpf_prog_load("helloworld.bpf.o", BPF_PROG_TYPE_KPROBE, &obj, &prog_fd);
if (err) {
fprintf(stderr, "Failed to load BPF object file: %s\n", strerror(-err));
return EXIT_FAILURE;
}
/* Attach the BPF program to the kprobe/sys_clone kernel function */
err = bpf_attach_kprobe(prog_fd, BPF_PROBE_ENTRY, "sys_clone");
if (err) {
fprintf(stderr, "Failed to attach BPF program to kprobe/sys_clone: %s\n", strerror(-err));
return EXIT_FAILURE;
}
/* Wait for the user to press Enter */
printf("Press Enter to detach the BPF program...\n");
getchar();
/* Detach the BPF program from the kprobe/sys_clone kernel function */
err = bpf_detach_kprobe(prog_fd, BPF_PROBE_ENTRY, "sys_clone");
if (err) {
fprintf(stderr, "Failed to detach BPF program from kprobe/sys_clone: %s\n", strerror(-err));
return EXIT_FAILURE;
}
/* Clean up */
bpf_object__close(obj);
return EXIT_SUCCESS;
}
```
4.编译和链接BPF程序
```shell
clang -O2 -target bpf -c helloworld.bpf.c -o helloworld.bpf.o
```
5.编译和链接用户空间程序
```shell
clang helloworld.c -o helloworld -lbpf
```
6.运行用户空间程序
```shell
sudo ./helloworld
```
7.在另一个终端窗口中查看BPF程序的输出
```shell
sudo cat /sys/kernel/debug/tracing/trace_pipe
```
相关推荐
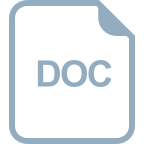








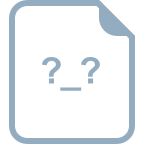
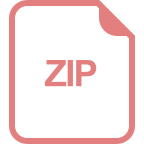
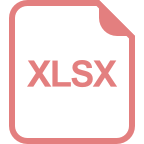