已经用itertools.combinations(leaves_select, 4)获得了组合,如果我想把每个组合转化成列表并且逐个读取列表,应该怎么写python代码?
时间: 2024-05-15 09:18:52 浏览: 47
可以使用for循环遍历每个组合,并将其转化为列表。代码示例如下:
```
import itertools
leaves_select = [1, 2, 3, 4, 5, 6]
comb = itertools.combinations(leaves_select, 4)
for c in comb:
c_list = list(c)
print(c_list)
```
输出结果为:
```
[1, 2, 3, 4]
[1, 2, 3, 5]
[1, 2, 3, 6]
[1, 2, 4, 5]
[1, 2, 4, 6]
[1, 2, 5, 6]
[1, 3, 4, 5]
[1, 3, 4, 6]
[1, 3, 5, 6]
[1, 4, 5, 6]
[2, 3, 4, 5]
[2, 3, 4, 6]
[2, 3, 5, 6]
[2, 4, 5, 6]
[3, 4, 5, 6]
```
相关问题
python3.11的itertools模块中itertools.combinations_with_replacement()函数的作用?以列表方式写出该函数必传参数和所有可选参数并说明含义?一个简单的案例并加上注释?
`itertools.combinations_with_replacement(iterable, r)`函数可以返回可重复组合的迭代器,其中`iterable`是输入迭代器, `r`是组合元素个数。
必传参数:
- `iterable`:要进行组合的可迭代对象。
可选参数:
- `r`:组合元素个数,如果不传入该参数,则默认为`len(iterable)`。
下面是一个简单的案例:
```python
import itertools
# 创建一个列表
lst = ['A', 'B', 'C']
# 返回由可重复组合的元素构成的迭代器
combinations = itertools.combinations_with_replacement(lst, 2)
# 遍历迭代器并打印结果
for c in combinations:
print(c)
```
输出结果为:
```
('A', 'A')
('A', 'B')
('A', 'C')
('B', 'B')
('B', 'C')
('C', 'C')
```
以上代码中,我们使用了`combinations_with_replacement()`函数对列表`lst`进行了可重复组合,其中每个组合中有2个元素。最后我们遍历了返回的迭代器并打印了所有的组合结果。
itertools.combinations
itertools.combinations 是 Python 标准库中的一个函数,它接受两个参数:一个可迭代对象和一个整数 n,返回该可迭代对象中长度为 n 的所有子集。该函数产生的是一个迭代器,而不是一个列表。
阅读全文
相关推荐
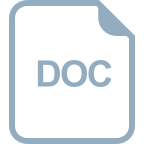
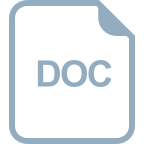
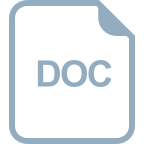













