2.Reliability and Maintainability Simulation involves a large number of random variables. The use of dynamic arrays will greatly improve the efficiency of simulation and the scale of problem solving. Please design Vector. This problem requires the implementation of a vector class template, which can realize the storage and access of data. (1) [] operator can only access the existing elements. (2) The add method can automatically expand the internal storage space when accessing. Note that the behavior of this vector is different from that of std:: vector. Function interface definition: template <class T> class Vector { ... } Example of referee test procedure: #include <iostream> using namespace std; /* Todo: write down your code here! */ int main() { Vector<int> vint; int n,m; cin >> n >> m; for ( int i=0; i<n; i++ ) { // add() can inflate the vector automatically vint.add(i); } // get_size() returns the number of elements stored in the vector cout << vint.get_size() << endl; cout << vint[m] << endl; // remove() removes the element at the index which begins from zero vint.remove(m); cout << vint.add(-1) << endl; cout << vint[m] << endl; Vector<int> vv = vint; cout << vv[vv.get_size()-1] << endl; vv.add(m); cout << vint.get_size() << endl; } Input example: 100 50 Output example: 100 50 99 51 -1 100
时间: 2024-02-01 10:02:41 浏览: 66
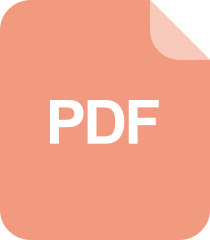
The effect of omitting a limited number of subtests on the full scale reliability of the WISC-R
Here is the implementation of the Vector class template:
```
template<class T>
class Vector {
private:
T* data; // pointer to the actual data
int capacity; // current capacity of the vector
int size; // current size of the vector
public:
// constructor
Vector() {
data = nullptr;
capacity = 0;
size = 0;
}
// copy constructor
Vector(const Vector& other) {
capacity = other.capacity;
size = other.size;
data = new T[capacity];
for (int i = 0; i < size; i++) {
data[i] = other.data[i];
}
}
// destructor
~Vector() {
if (data != nullptr) {
delete[] data;
}
}
// [] operator for accessing elements
T& operator[](int index) {
if (index >= size) {
throw std::out_of_range("Index out of range");
}
return data[index];
}
// add method for adding elements
void add(const T& value) {
if (size == capacity) {
int newCapacity = capacity == 0 ? 1 : capacity * 2;
T* newData = new T[newCapacity];
for (int i = 0; i < size; i++) {
newData[i] = data[i];
}
if (data != nullptr) {
delete[] data;
}
data = newData;
capacity = newCapacity;
}
data[size++] = value;
}
// remove method for removing elements
void remove(int index) {
if (index < 0 || index >= size) {
throw std::out_of_range("Index out of range");
}
for (int i = index; i < size - 1; i++) {
data[i] = data[i + 1];
}
size--;
}
// get_size method for getting the current size of the vector
int get_size() const {
return size;
}
};
```
In the main function, we first create a Vector object `vint` of type `int`. We read in two integers `n` and `m` from the input, and then use a for loop to add `n` elements to the vector using the `add` method. We then print the size of the vector using the `get_size` method, and print the element at index `m` using the `[]` operator. We then remove the element at index `m` using the `remove` method, and add a new element with value `-1` using the `add` method. We print the new element at index `m` using the `[]` operator. We create a new Vector object `vv` by copying `vint`, and print the last element of `vv` using the `[]` operator. Finally, we add an element with value `m` to `vv`, and print the size of `vint` using the `get_size` method.
I hope this helps!
阅读全文
相关推荐
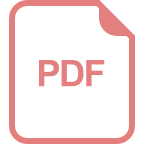
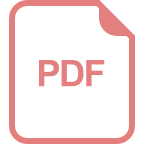


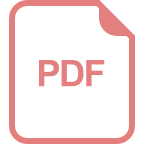
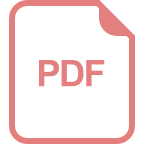
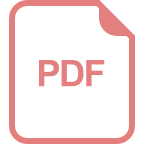
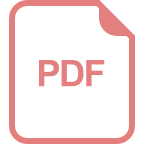
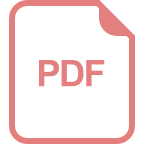
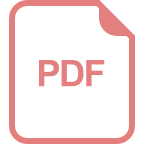
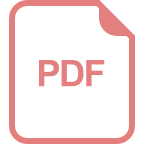
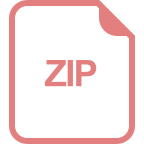
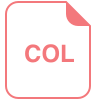

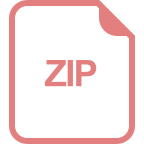
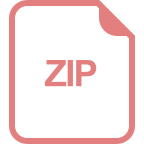