计算两个矩阵的乘积的方法Matrix multiply(Matrix m)
时间: 2024-05-15 20:12:58 浏览: 110
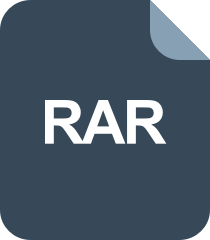
计算两个矩形的乘积
矩阵乘法的实现需要对两个矩阵中的每个元素进行遍历计算,具体实现步骤如下:
1. 首先判断两个矩阵是否满足矩阵乘法的条件,即左矩阵的列数等于右矩阵的行数。
2. 创建一个新的矩阵,其行数为左矩阵的行数,列数为右矩阵的列数。
3. 对于新矩阵中的每个元素,它的值为左矩阵中对应行与右矩阵中对应列的乘积之和。
4. 最后返回新矩阵。
下面是一个 Java 实现:
```java
public class Matrix {
private int[][] data;
private int rows;
private int cols;
public Matrix(int[][] data) {
this.data = data;
this.rows = data.length;
this.cols = data[0].length;
}
public Matrix multiply(Matrix m) {
if (this.cols != m.rows) {
throw new IllegalArgumentException("Cannot multiply matrices with incompatible sizes.");
}
int[][] result = new int[this.rows][m.cols];
for (int i = 0; i < this.rows; i++) {
for (int j = 0; j < m.cols; j++) {
int sum = 0;
for (int k = 0; k < this.cols; k++) {
sum += this.data[i][k] * m.data[k][j];
}
result[i][j] = sum;
}
}
return new Matrix(result);
}
}
```
阅读全文
相关推荐
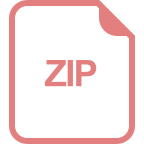
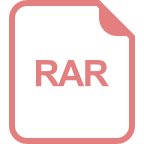













