pthread实现多线程矩阵
时间: 2023-10-07 13:05:24 浏览: 70
下面是一个使用 pthread 库实现多线程矩阵乘法的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define N 3
int A[N][N] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
int B[N][N] = {{10, 11, 12}, {13, 14, 15}, {16, 17, 18}};
int C[N][N] = {{0, 0, 0}, {0, 0, 0}, {0, 0, 0}};
typedef struct {
int row;
int col;
} args;
void *mult(void *arg) {
args *data = (args *)arg;
for (int i = 0; i < N; i++) {
C[data->row][data->col] += A[data->row][i] * B[i][data->col];
}
pthread_exit(NULL);
}
int main() {
pthread_t threads[N*N];
int count = 0;
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
args *data = (args *)malloc(sizeof(args));
data->row = i;
data->col = j;
pthread_create(&threads[count], NULL, mult, data);
count++;
}
}
for (int i = 0; i < N*N; i++) {
pthread_join(threads[i], NULL);
}
printf("C:\n");
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
printf("%d ", C[i][j]);
}
printf("\n");
}
return 0;
}
```
这个程序创建了 N*N 个线程,每个线程将矩阵 C 的一个元素计算出来。每个线程的参数是它要计算的元素的行和列号。在主函数中,使用 pthread_join 函数等待所有线程完成计算,然后打印结果矩阵 C。
需要注意的是,这个程序没有进行任何线程间的同步,因此可能会出现竞争条件。为了避免这种情况,可以使用互斥锁或其他同步机制来保护共享数据的访问。
阅读全文
相关推荐
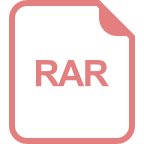
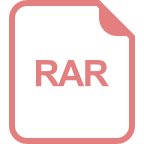
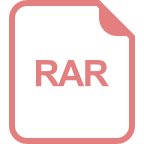
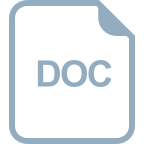




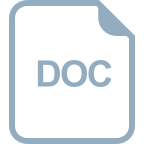
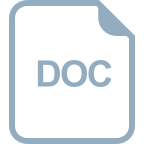
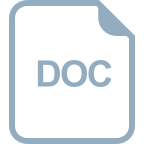
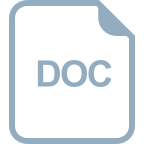
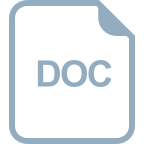
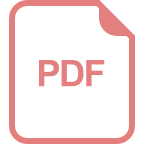
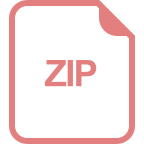