import os import time# 获取当前目录下的所有文件 files = os.listdir('.')# 遍历文件 for file in files: # 获取文件的创建时间 time_created = os.path.getctime(file) # 计算文件创建时距离当前时间的天数 day_passed = (time.time() - time_created) // 86400 # 如果文件是PNG格式的 if file.endswith('.png'): # 如果创建时间距离当前时间超过五天,则删除文件 if day_passed > 5: os.remove(file) # 如果文件是JPG格式的 elif file.endswith('.jpg'): # 如果创建时间距离当前时间超过30天,则删除文件 if day_passed > 30: os.remove(file)
时间: 2023-03-20 21:00:58 浏览: 155
Hello! It looks like you're trying to write some Python code, but the code you've provided isn't a valid Python program yet. Here's an example of how you could modify your code to make it a valid Python program that imports the `os` module and the `time` module:
```
import os
import time
```
This code will allow you to use functions and classes from the `os` and `time` modules in your program. If you have any specific questions or issues, feel free to ask!
相关问题
import os import pandas as pd os.chdir('C:\\Excel') cwd = os.path.abspath('') files = os.listdir(cwd) #方法一:获取所给文件的第一个工作表 df = pd.DataFrame() for file in files: if file.endswith('.xlsx'): df = df.append(pd.read_excel(file),ignore_index=True) df.head() df.to_excel('total_sales.xlsx') #方法二:获取所给文件的所有工作表 df_total = pd.DataFrame() #遍历所有Excel文件 for file in files: if file.endswith('.xlsx'): Excel_file = pd.ExcelFile(file) sheets = Excel_file.sheet_names #遍历Excel文件中的工作表' for sheet in sheets: df = Excel_file.parse(sheet_name = sheet) df_total = df_total.append(df) df_total.to_excel('combined_file.xlsx') print(df_total)
这段代码是用来将多个 Excel 文件中的数据合并到一个文件中。第一种方法是将每个 Excel 文件的第一个工作表读入一个 DataFrame 中,并使用 `append` 方法将多个 DataFrame 合并到一个 DataFrame 中,最后将合并后的 DataFrame 写入到一个新的 Excel 文件中。第二种方法是遍历每个 Excel 文件中的所有工作表,并使用 `parse` 方法将每个工作表读入到一个 DataFrame 中,然后使用 `append` 方法将所有 DataFrame 合并到一个 DataFrame 中,最后将合并后的 DataFrame 写入到一个新的 Excel 文件中。这两种方法都是使用 `pandas` 库实现的。
import os # 获取文件夹路径 folder_path = 'label' folder_path1 = 'new' # 获取文件夹中的所有txt文件 files = [f for f in os.listdir(folder_path) if f.endswith('.txt')] # 遍历所有txt文件 for filename in files: file_path = os.path.join(folder_path, filename) filename=filename[7:13] id='' for c in filename: if c.isdigit(): id += c if len(id)<6: id = int(id) filename = str(id) file_path = os.path.join(folder_path, filename) with open(filename, 'r', encoding='utf-8') as file: content = file.read() # 替换第一个字符串 content = content.replace(content.split()[0], str(start_num)) # 写入修改后的内容 new_filename = filename.replace('.txt', '.txt') new_file_path = os.path.join(folder_path1, new_filename) with open(new_file_path, 'w', encoding='utf-8') as new_file: new_file.write(content) start_num = 0 increment = 1 # 更新起始数值 if id % 3 == 0: start_num += increment为什么找不到文件了,有什么办法解决
根据你提供的代码,找不到文件的问题可能是因为文件路径没有正确设置。具体来说,你在打开文件时使用了`filename`作为文件路径,但是`filename`在此之前已经被修改为一个数字或字符串,而不是完整的文件路径。
为了解决这个问题,你可以使用`file_path`变量来打开文件和写入修改后的内容。将以下代码段:
```
with open(filename, 'r', encoding='utf-8') as file:
content = file.read()
```
替换为:
```
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
```
同样地,将以下代码段:
```
new_filename = filename.replace('.txt', '.txt')
new_file_path = os.path.join(folder_path1, new_filename)
with open(new_file_path, 'w', encoding='utf-8') as new_file:
new_file.write(content)
```
替换为:
```
new_filename = filename.replace('.txt', '.txt')
new_file_path = os.path.join(folder_path1, new_filename)
with open(os.path.join(folder_path1, new_file_path), 'w', encoding='utf-8') as new_file:
new_file.write(content)
```
这样修改后,文件路径应该被正确地设置,可以找到并操作文件。如果问题仍然存在,请提供更多错误信息以便进一步排查。
阅读全文
相关推荐
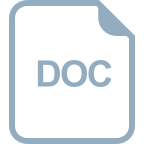
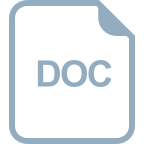
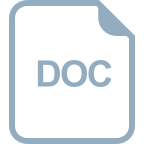














