Android 设置二级列表的adapter 父列表一个 子列表多个 使用list数据 的demo
时间: 2024-03-11 11:50:02 浏览: 12
好的,下面是一个基于 List 数据的 Android 二级列表的 Adapter 示例代码:
```java
public class MyExpandableListAdapter extends BaseExpandableListAdapter {
private Context context;
private List<String> parentList;
private Map<String, List<String>> childMap;
public MyExpandableListAdapter(Context context, List<String> parentList, Map<String, List<String>> childMap) {
this.context = context;
this.parentList = parentList;
this.childMap = childMap;
}
@Override
public int getGroupCount() {
return parentList.size();
}
@Override
public int getChildrenCount(int groupPosition) {
String parent = parentList.get(groupPosition);
List<String> childList = childMap.get(parent);
return childList.size();
}
@Override
public Object getGroup(int groupPosition) {
return parentList.get(groupPosition);
}
@Override
public Object getChild(int groupPosition, int childPosition) {
String parent = parentList.get(groupPosition);
List<String> childList = childMap.get(parent);
return childList.get(childPosition);
}
@Override
public long getGroupId(int groupPosition) {
return groupPosition;
}
@Override
public long getChildId(int groupPosition, int childPosition) {
return childPosition;
}
@Override
public boolean hasStableIds() {
return false;
}
@Override
public View getGroupView(int groupPosition, boolean isExpanded, View convertView, ViewGroup parent) {
if (convertView == null) {
convertView = LayoutInflater.from(context).inflate(R.layout.list_parent_item, parent, false);
}
TextView tvParent = convertView.findViewById(R.id.tv_parent);
tvParent.setText(parentList.get(groupPosition));
return convertView;
}
@Override
public View getChildView(int groupPosition, int childPosition, boolean isLastChild, View convertView, ViewGroup parent) {
if (convertView == null) {
convertView = LayoutInflater.from(context).inflate(R.layout.list_child_item, parent, false);
}
TextView tvChild = convertView.findViewById(R.id.tv_child);
String parent = parentList.get(groupPosition);
List<String> childList = childMap.get(parent);
tvChild.setText(childList.get(childPosition));
return convertView;
}
@Override
public boolean isChildSelectable(int groupPosition, int childPosition) {
return true;
}
}
```
在这个示例中,我们传入了一个上下文 Context,一个父列表的 List,以及一个子列表的 Map,Map 中存储的是每个父列表对应的多个子列表。在 Adapter 中,我们通过实现一系列方法来实现二级列表的显示和交互。
在 getGroupCount 方法中,我们返回父列表的数量;在 getChildrenCount 方法中,我们通过父列表的位置获取对应的子列表,并返回子列表的数量。在 getGroup 和 getChild 方法中,我们分别返回父列表和子列表中对应位置的数据。在 getGroupId 和 getChildId 方法中,我们返回对应位置的 ID,这里我们简单地返回了位置的数值。在 isChildSelectable 方法中,我们返回 true 表示子列表可选中。
在 getGroupView 方法中,我们通过 convertView 参数来复用已经存在的父列表 Item View,如果 convertView 为空,则使用 LayoutInflater 创建一个新的 Item View。在这个方法中,我们设置了父列表 Item View 中的 TextView 显示对应的父列表数据。在 getChildView 方法中,我们同样使用 convertView 来复用已经存在的子列表 Item View,如果 convertView 为空,则使用 LayoutInflater 创建一个新的 Item View。在这个方法中,我们通过父列表的位置获取对应的子列表,并设置显示对应的子列表数据。
最后,我们就可以在 Activity 中使用这个 Adapter 了,示例代码如下:
```java
public class MainActivity extends AppCompatActivity {
private ExpandableListView expandableListView;
private MyExpandableListAdapter adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
expandableListView = findViewById(R.id.expandable_list_view);
List<String> parentList = new ArrayList<>();
parentList.add("Parent 1");
parentList.add("Parent 2");
parentList.add("Parent 3");
Map<String, List<String>> childMap = new HashMap<>();
List<String> childList1 = new ArrayList<>();
childList1.add("Child 1-1");
childList1.add("Child 1-2");
childList1.add("Child 1-3");
childMap.put(parentList.get(0), childList1);
List<String> childList2 = new ArrayList<>();
childList2.add("Child 2-1");
childList2.add("Child 2-2");
childList2.add("Child 2-3");
childMap.put(parentList.get(1), childList2);
List<String> childList3 = new ArrayList<>();
childList3.add("Child 3-1");
childList3.add("Child 3-2");
childList3.add("Child 3-3");
childMap.put(parentList.get(2), childList3);
adapter = new MyExpandableListAdapter(this, parentList, childMap);
expandableListView.setAdapter(adapter);
}
}
```
在这个示例中,我们首先通过 findViewById 获取了 ExpandableListView 的实例。然后我们创建了一个包含父列表和子列表数据的 List 和 Map,这里我们简单地使用了硬编码的方式来创建。最后,我们创建了一个 MyExpandableListAdapter 的实例,并设置为 ExpandableListView 的 Adapter,完成了二级列表的设置。
相关推荐
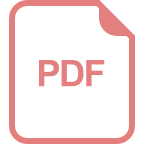
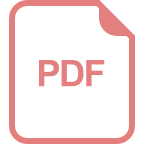
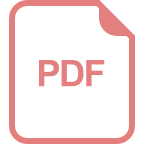














