把上面的代码封装成一个函数
时间: 2023-02-09 09:31:51 浏览: 81
```python
def print_hello():
print("Hello, World!")
```
这是封装成一个函数的代码,函数名为 "print_hello"。使用时只需要调用这个函数即可。
```python
print_hello()
```
输出结果为:
```
Hello, World!
```
相关问题
将上面代码封装成一个函数
好的,我可以帮你将代码封装成一个函数。以下是一个示例:
```python
import pyautogui
import re
def process_text():
# 获取鼠标左键双击时的坐标
zuobiao_1 = pyautogui.position()
pyautogui.doubleClick(button='left')
# 控制电脑键盘操作快捷键ctrl+c
pyautogui.hotkey('ctrl', 'c')
# 获取剪切板内容赋值给变量text_1
text_1 = pyautogui.paste()
# 控制鼠标相对变量zuobiao_1向下移动45,并进行左键单击
pyautogui.moveRel(0, 45)
pyautogui.click(button='left')
# 获取此时鼠标左键单击时的坐标
zuobiao_2 = pyautogui.position()
# 控制鼠标相对变量zuobiao_2向右移动150,并进行左键双击
pyautogui.moveRel(150, 0)
pyautogui.doubleClick(button='left')
# 控制电脑键盘操作快捷键ctrl+c
pyautogui.hotkey('ctrl', 'c')
# 获取剪切板内容赋值给变量text_2
text_2 = pyautogui.paste()
# 使用正则匹配的方式对变量text_2中的文本进行处理
lines = text_2.split('\n')
text_3 = ''
for line in lines:
if re.search('局|处', line):
continue
if re.search('单位', line):
continue
text_3 += line + '\n'
# 将text_1作为第一行和text_3拼接在一起,赋值给变量text_2
text_2 = text_1 + '\n' + text_3
# 控制鼠标移动到屏幕特定位置,控制鼠标左键单击
pyautogui.moveTo(x, y)
pyautogui.click(button='left')
# 控制键盘操作ctrl+v
pyautogui.hotkey('ctrl', 'v')
# 控制键盘enter
pyautogui.press('enter')
# 调用函数进行处理
process_text()
```
你可以将需要封装的代码放入这个函数中,并根据你的实际需求进行调整。然后,你可以通过调用 `process_text()` 函数来执行这些操作。记得在调用函数之前,先确保安装了PyAutoGUI库,并提供正确的坐标值。
请把上面的这段代码封装为一个函数
好的,以下是封装好的函数:
```python
import cv2
import numpy as np
import matplotlib.pyplot as plt
def liquid_concentration_prediction(image_path):
# 读入图片
img = cv2.imread(image_path)
# 获取图片长宽
height, width = img.shape[:2]
# 计算每个圆的半径
width = max(width, height)
height = min(width, height)
a = int(width / 12) / 2
b = int(height / 8) / 2
c = int(a)
d = int(b)
r = min(c, d)
# 计算圆心坐标
centers = []
for j in range(8):
for i in range(12):
cx = 2 * r * j + r
cy = 2 * r * i + r
centers.append((cx, cy))
# 提取灰度值
gray_values = []
for i in range(96):
x, y = centers[i][0], centers[i][1]
mask = np.zeros_like(img)
cv2.circle(mask, (x, y), r, (255, 255, 255), -1)
masked_img = cv2.bitwise_and(img, mask)
gray_img = cv2.cvtColor(masked_img, cv2.COLOR_RGB2GRAY)
gray_value = np.mean(gray_img)
gray_values.append(gray_value)
# 拟合数据
x_values = gray_values[:16] # 16个用于训练的灰度值
x_prediction_values = gray_values[16:] # 80个用于预测的灰度值
y_values = [0.98, 0.93, 0.86, 0.79, 0.71, 0.64, 0.57, 0.50, 0.43, 0.36, 0.29, 0.21, 0.14, 0.07, 0.05, 0.01] # 16个液体浓度值
# 使用numpy的polyfit函数进行线性拟合
fit = np.polyfit(x_values, y_values, 1)
# 使用拟合系数构建线性函数
lin_func = np.poly1d(fit)
# 生成新的80个数据的x值
new_x = x_prediction_values
# 预测新的80个数据的y值
new_y = lin_func(new_x)
# 输出预测结果
result = list(new_y)
row3 = result[:8]
row4 = result[8:16]
row5 = result[16:24]
row6 = result[24:32]
row7 = result[32:40]
row8 = result[40:48]
row9 = result[48:56]
row10 = result[56:64]
row11 = result[64:72]
row12 = result[72:80]
print("第三列:", row3)
print("第四列:", row4)
print("第五列:", row5)
print("第六列:", row6)
print("第七列:", row7)
print("第八列:", row8)
print("第九列:", row9)
print("第十列:", row10)
print("第十一列:", row11)
print("第十二列:", row12)
```
这个函数接收一个图片路径作为参数,然后输出预测结果。你可以通过调用该函数来进行液体浓度的预测。
相关推荐
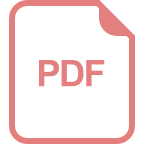
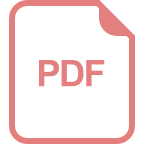
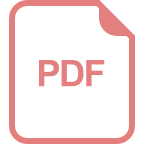












