我校接受xxx超市的一个项目,该项目旨在为xxx超市设计一套超市自助结算系统,实现顾客录入货品信息,系统显示付款总额,以及统计目前的营业总额等功能。 其中货品的基本信息包括:编号(例如001),名称(例如面包),单价(例如9.9,保留一位有效数字)等。 其中顾客的流水信息包括:流水编号(例如1),消费金额(例如86.7,保留一位有效数字)等。 请设计一个结算系统,实现如下功能: (1)添加、查询和删除货品的基本信息(货品种类上限设置为100); (2)输入某位顾客购买的所有货品及其数量,输出结算总额; (3)统计超市目前的营业总额,并输出。 要求:结合组合数据类型和之前学习的内容,使用列表、元组和字典等元素进行编程,
时间: 2024-03-21 09:44:36 浏览: 16
好的,这是一个比较完整的项目,我可以为您提供一些思路和代码实现。
首先,我们需要定义货品和顾客流水的数据结构。对于货品,我们可以使用字典来存储每个货品的基本信息,例如:
```
goods = {'001': {'name': '面包', 'price': 9.9},
'002': {'name': '牛奶', 'price': 12.5},
'003': {'name': '饼干', 'price': 6.8},
...}
```
对于顾客流水,我们可以使用列表,每个元素表示一条流水:
```
records = [{'id': 1, 'items': {'001': 2, '003': 1}, 'total': 27.6},
{'id': 2, 'items': {'002': 1, '004': 3}, 'total': 48.5},
...]
```
其中,每条流水记录包括流水编号、购买的货品及其数量、以及结算总额。
接下来,我们可以实现添加、查询和删除货品信息的功能。对于添加货品,我们可以使用字典的`update()`方法:
```python
def add_goods(goods, code, name, price):
if len(goods) >= 100:
print("货品种类已达上限!")
return
goods[code] = {'name': name, 'price': price}
print("货品添加成功!")
```
查询货品信息可以直接通过字典的键值来查找:
```python
def query_goods(goods, code):
if code in goods:
print("编号:{}\n名称:{}\n单价:{}".format(code, goods[code]['name'], goods[code]['price']))
else:
print("货品不存在!")
```
删除货品信息可以使用字典的`pop()`方法:
```python
def delete_goods(goods, code):
if code in goods:
goods.pop(code)
print("货品删除成功!")
else:
print("货品不存在!")
```
接下来是输入购买信息和计算结算总额的功能。我们可以使用一个函数来实现,参数包括购买货品的字典和货品信息的字典:
```python
def checkout(goods, items):
total = 0.0
for code, amount in items.items():
if code not in goods:
print("货品不存在:{}".format(code))
continue
price = goods[code]['price']
total += price * amount
print("{} x {} = {}".format(goods[code]['name'], amount, price * amount))
print("结算总额:{}".format(round(total, 1)))
return total
```
最后是统计营业总额的功能。我们只需要遍历所有的流水记录,并累加结算总额即可:
```python
def total_sales(records):
total = 0.0
for record in records:
total += record['total']
print("营业总额:{}".format(round(total, 1)))
return total
```
以上函数可以组合使用,实现一个完整的结算系统。完整代码如下:
相关推荐
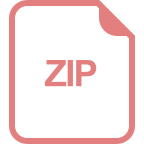














