使用c++语言设计一个模拟超市购物的计算机系统
时间: 2024-03-18 13:42:56 浏览: 27
好的,这是一个比较复杂的系统,需要设计多个模块和数据结构。下面是一个简单的设计方案,供参考。
1. 数据结构
超市商品信息结构体:
```
struct Item
{
string name; // 商品名称
double price; // 商品价格
int stock; // 商品库存
};
```
购物车商品信息结构体:
```
struct CartItem
{
Item item; // 商品信息
int quantity; // 商品数量
};
```
2. 模块设计
超市商品管理模块:实现商品信息的添加、删除、修改和查询等功能。
购物车管理模块:实现购物车中商品信息的添加、删除、修改和查询等功能。
结算管理模块:实现结算、支付和找零等功能。
3. 算法设计
商品信息添加算法:
```
item.name = "XXX";
item.price = 99.99;
item.stock = 100;
```
商品信息删除算法:
```
// 从商品列表中删除名称为name的商品
for (int i = 0; i < itemList.size(); i++)
{
if (itemList[i].name == name)
{
itemList.erase(itemList.begin() + i);
break;
}
}
```
商品信息修改算法:
```
// 修改名称为name的商品价格为newPrice
for (int i = 0; i < itemList.size(); i++)
{
if (itemList[i].name == name)
{
itemList[i].price = newPrice;
break;
}
}
```
商品信息查询算法:
```
// 查询名称为name的商品信息
for (int i = 0; i < itemList.size(); i++)
{
if (itemList[i].name == name)
{
cout << "名称:" << itemList[i].name << endl;
cout << "价格:" << itemList[i].price << endl;
cout << "库存:" << itemList[i].stock << endl;
break;
}
}
```
购物车商品添加算法:
```
// 添加名称为name的商品到购物车中,数量为quantity
for (int i = 0; i < itemList.size(); i++)
{
if (itemList[i].name == name)
{
if (itemList[i].stock >= quantity)
{
CartItem cartItem;
cartItem.item = itemList[i];
cartItem.quantity = quantity;
cartList.push_back(cartItem);
itemList[i].stock -= quantity;
break;
}
else
{
cout << "库存不足!" << endl;
break;
}
}
}
```
购物车商品删除算法:
```
// 从购物车中删除名称为name的商品
for (int i = 0; i < cartList.size(); i++)
{
if (cartList[i].item.name == name)
{
itemList[i].stock += cartList[i].quantity;
cartList.erase(cartList.begin() + i);
break;
}
}
```
购物车商品修改算法:
```
// 修改购物车中名称为name的商品数量为newQuantity
for (int i = 0; i < cartList.size(); i++)
{
if (cartList[i].item.name == name)
{
if (itemList[i].stock + cartList[i].quantity >= newQuantity)
{
int delta = newQuantity - cartList[i].quantity;
cartList[i].quantity = newQuantity;
itemList[i].stock -= delta;
break;
}
else
{
cout << "库存不足!" << endl;
break;
}
}
}
```
结算算法:
```
// 计算购物车中商品的总价和总数量
double totalPrice = 0;
int totalQuantity = 0;
for (int i = 0; i < cartList.size(); i++)
{
totalPrice += cartList[i].item.price * cartList[i].quantity;
totalQuantity += cartList[i].quantity;
}
// 输出总价和总数量
cout << "总价:" << totalPrice << endl;
cout << "总数量:" << totalQuantity << endl;
// 计算找零
double change = 0;
double paid = 0;
while (paid < totalPrice)
{
double amount;
cout << "请输入支付金额:";
cin >> amount;
paid += amount;
}
change = paid - totalPrice;
cout << "找零:" << change << endl;
```
4. 更完整的实现
以上只是一个简单的设计方案,实际的实现可能会更加复杂。完整的实现需要考虑以下问题:
- 如何保存商品信息和购物车信息,以便下次启动程序时可以继续使用?
- 如何处理并发访问和并发修改,以避免数据不一致的问题?
- 如何设计用户界面,使得用户可以方便地使用系统?
- 如何进行错误处理,以避免程序崩溃或产生不正确的结果?
- 如何进行性能优化,以提高系统的响应速度和吞吐量?
这些问题都需要仔细思考,并在实现过程中进行适当的调整和改进。
相关推荐
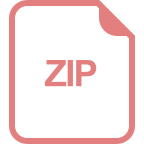















