IDEA TimeZone.setDefault 报红色
时间: 2024-03-08 17:46:00 浏览: 59
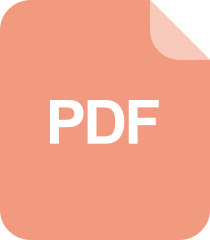
Server returns invalid timezone. Go to ‘Advanced’ tab and set ‘serverTimezone’ property manually.

如果在 IDEA 中使用 `TimeZone.setDefault()` 方法报红色,可能是因为该方法已被标记为过时(deprecated)。在 Java 9 中,该方法已被替换为 `ZoneId.systemDefault()` 方法。
建议使用新的方法 `ZoneId.systemDefault()`,它返回代表系统默认时区的 `ZoneId` 对象。示例代码如下:
```
ZoneId defaultZoneId = ZoneId.systemDefault();
```
如果你必须使用旧的方法 `TimeZone.setDefault()`,你可以通过在方法名前添加 `@SuppressWarnings("deprecation")` 注解来抑制警告。示例代码如下:
```
@SuppressWarnings("deprecation")
TimeZone.setDefault(timezone);
```
阅读全文
相关推荐
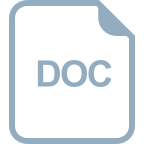
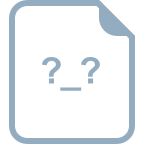



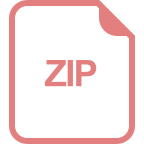
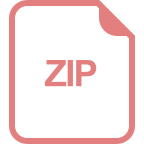
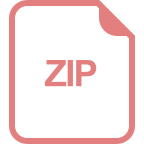
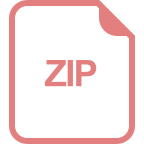
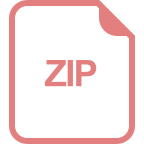
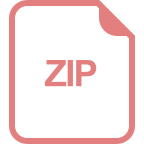
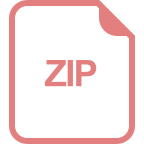
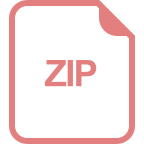