ubuntu20.04下如何用cpp代码使usb设备下电
时间: 2024-03-01 08:55:54 浏览: 157
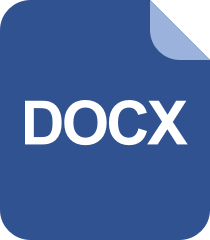
ubuntu20.04下QT安装
在Ubuntu 20.04中,可以使用 libusb 库来控制 USB 设备。下面是一个简单的 C++ 代码示例,它使用 libusb 库来控制 USB 设备的上电和下电:
```c++
#include <iostream>
#include <libusb-1.0/libusb.h>
int main()
{
libusb_context* context = NULL;
libusb_device_handle* device_handle = NULL;
// 初始化 libusb 库
int result = libusb_init(&context);
if (result < 0)
{
std::cerr << "libusb initialization failed: " << libusb_error_name(result) << std::endl;
return -1;
}
// 打开 USB 设备
device_handle = libusb_open_device_with_vid_pid(context, VENDOR_ID, PRODUCT_ID);
if (device_handle == NULL)
{
std::cerr << "Failed to open device" << std::endl;
libusb_exit(context);
return -1;
}
// 下电
result = libusb_detach_kernel_driver(device_handle, 0);
if (result == LIBUSB_SUCCESS)
{
std::cout << "Device detached from kernel driver" << std::endl;
}
else if (result == LIBUSB_ERROR_NOT_FOUND)
{
std::cout << "No kernel driver attached to device" << std::endl;
}
else
{
std::cerr << "Failed to detach kernel driver: " << libusb_error_name(result) << std::endl;
libusb_close(device_handle);
libusb_exit(context);
return -1;
}
// 上电
result = libusb_attach_kernel_driver(device_handle, 0);
if (result == LIBUSB_SUCCESS)
{
std::cout << "Device attached to kernel driver" << std::endl;
}
else if (result == LIBUSB_ERROR_NOT_FOUND)
{
std::cout << "No kernel driver available for device" << std::endl;
}
else
{
std::cerr << "Failed to attach kernel driver: " << libusb_error_name(result) << std::endl;
libusb_close(device_handle);
libusb_exit(context);
return -1;
}
// 关闭设备并清理资源
libusb_close(device_handle);
libusb_exit(context);
return 0;
}
```
在上面的代码示例中,`VENDOR_ID` 和 `PRODUCT_ID` 分别是你要控制的 USB 设备的厂商和产品 ID。你需要将它们替换为你自己设备的值。代码中的 `libusb_detach_kernel_driver()` 和 `libusb_attach_kernel_driver()` 分别用于下电和上电设备。请注意,这些函数的返回值将指示执行结果是否成功。
运行代码时,请确保你的用户帐户具有使用 USB 设备的权限。你可以将你的用户添加到 `plugdev` 组中,以便具有这些权限。
阅读全文
相关推荐
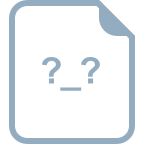














