(3)客户管理 运用C++语言描述客户类、地区类,每一个类应包含数据成员和成员函数。设计基类和派生类,并运用多态性和虚函数的知识。注重面向对象程序设计理论知识的理解与实际的动手编程能力,要求学生设计具有继承与派生以及多态性的类,理解面向对象程序设计的核心的概念。 本课程设计要实现的主要功能如下: 1)建立客户类、地区类 2)客户、地区信息的初始化 3)客户、地区信息的添加、修改、删除、查找 4)客户、地区信息的输出 5)将客户信息保存为文件
时间: 2024-03-04 21:49:38 浏览: 20
好的,这是一个关于客户管理的C++程序设计。我们需要设计一个客户类和地区类,每个类都应该包含数据成员和成员函数。此外,我们需要设计一个基类和派生类,并且运用多态性和虚函数的知识。我们需要注重面向对象程序设计的理论知识和实际的动手编程能力,设计具有继承与派生以及多态性的类,理解面向对象程序设计的核心概念。
在这个程序中,我们需要实现以下主要功能:
1. 建立客户类和地区类,包括数据成员和成员函数。
2. 初始化客户和地区信息。
3. 添加、修改、删除、查找客户和地区信息。
4. 输出客户和地区信息。
5. 将客户信息保存到文件中。
下面是一个基本的程序框架,你可以根据需要进行修改和完善:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
using namespace std;
// 客户基类
class Customer {
public:
Customer(string name, string address, string phone);
virtual ~Customer() {}
virtual void display() const;
virtual void update();
virtual void saveToFile(ofstream& ofs) const;
private:
string mName;
string mAddress;
string mPhone;
};
// 地区派生类
class Region : public Customer {
public:
Region(string name, string address, string phone, string region);
void display() const override;
void update() override;
void saveToFile(ofstream& ofs) const override;
private:
string mRegion;
};
// 客户管理类
class CustomerManager {
public:
CustomerManager();
~CustomerManager();
void addCustomer(Customer* customer);
void removeCustomer(const string& name);
void updateCustomer(const string& name);
void displayCustomers() const;
void saveToFile(const string& filename) const;
void loadFromFile(const string& filename);
private:
vector<Customer*> mCustomers;
};
// 客户基类实现
Customer::Customer(string name, string address, string phone)
: mName(name), mAddress(address), mPhone(phone)
{
}
void Customer::display() const
{
cout << "Name: " << mName << endl;
cout << "Address: " << mAddress << endl;
cout << "Phone: " << mPhone << endl;
}
void Customer::update()
{
cout << "Enter new name: ";
getline(cin, mName);
cout << "Enter new address: ";
getline(cin, mAddress);
cout << "Enter new phone: ";
getline(cin, mPhone);
}
void Customer::saveToFile(ofstream& ofs) const
{
ofs << mName << endl;
ofs << mAddress << endl;
ofs << mPhone << endl;
}
// 地区派生类实现
Region::Region(string name, string address, string phone, string region)
: Customer(name, address, phone), mRegion(region)
{
}
void Region::display() const
{
Customer::display();
cout << "Region: " << mRegion << endl;
}
void Region::update()
{
Customer::update();
cout << "Enter new region: ";
getline(cin, mRegion);
}
void Region::saveToFile(ofstream& ofs) const
{
Customer::saveToFile(ofs);
ofs << mRegion << endl;
}
// 客户管理类实现
CustomerManager::CustomerManager()
{
}
CustomerManager::~CustomerManager()
{
for (auto customer : mCustomers) {
delete customer;
}
}
void CustomerManager::addCustomer(Customer* customer)
{
mCustomers.push_back(customer);
}
void CustomerManager::removeCustomer(const string& name)
{
for (auto it = mCustomers.begin(); it != mCustomers.end(); ++it) {
if ((*it)->getName() == name) {
delete *it;
mCustomers.erase(it);
break;
}
}
}
void CustomerManager::updateCustomer(const string& name)
{
for (auto customer : mCustomers) {
if (customer->getName() == name) {
customer->update();
break;
}
}
}
void CustomerManager::displayCustomers() const
{
for (auto customer : mCustomers) {
customer->display();
cout << endl;
}
}
void CustomerManager::saveToFile(const string& filename) const
{
ofstream ofs(filename);
if (ofs.is_open()) {
for (auto customer : mCustomers) {
customer->saveToFile(ofs);
}
ofs.close();
} else {
cerr << "Error: failed to open file " << filename << endl;
}
}
void CustomerManager::loadFromFile(const string& filename)
{
ifstream ifs(filename);
if (ifs.is_open()) {
string name, address, phone, region;
while (getline(ifs, name) && getline(ifs, address) && getline(ifs, phone)) {
getline(ifs, region);
if (region.empty()) {
addCustomer(new Customer(name, address, phone));
} else {
addCustomer(new Region(name, address, phone, region));
}
}
ifs.close();
} else {
cerr << "Error: failed to open file " << filename << endl;
}
}
// 主函数
int main()
{
CustomerManager manager;
manager.loadFromFile("customers.txt");
int choice = 0;
while (choice != 6) {
cout << "Customer Management System" << endl;
cout << "1. Add customer" << endl;
cout << "2. Remove customer" << endl;
cout << "3. Update customer" << endl;
cout << "4. Display customers" << endl;
cout << "5. Save to file" << endl;
cout << "6. Exit" << endl;
cout << "Enter your choice: ";
cin >> choice;
cin.ignore();
switch (choice) {
case 1: {
cout << "Enter name: ";
string name;
getline(cin, name);
cout << "Enter address: ";
string address;
getline(cin, address);
cout << "Enter phone: ";
string phone;
getline(cin, phone);
cout << "Is this a region? (y/n): ";
string answer;
getline(cin, answer);
if (answer == "y") {
cout << "Enter region: ";
string region;
getline(cin, region);
manager.addCustomer(new Region(name, address, phone, region));
} else {
manager.addCustomer(new Customer(name, address, phone));
}
break;
}
case 2: {
cout << "Enter name: ";
string name;
getline(cin, name);
manager.removeCustomer(name);
break;
}
case 3: {
cout << "Enter name: ";
string name;
getline(cin, name);
manager.updateCustomer(name);
break;
}
case 4: {
manager.displayCustomers();
break;
}
case 5: {
manager.saveToFile("customers.txt");
break;
}
case 6: {
cout << "Exiting..." << endl;
break;
}
default: {
cout << "Invalid choice. Try again." << endl;
break;
}
}
}
return 0;
}
```
这段代码包含了客户基类、地区派生类和客户管理类的实现。你可以根据需要进行修改和完善。其中,客户基类和地区派生类包含了虚函数`display()`、`update()`和`saveToFile()`,这些函数可以根据需要进行重写。客户管理类实现了添加、删除、修改、显示和保存客户信息的功能。注意,在保存客户信息到文件时,我们需要将客户类型区分开来,因此在读取和保存文件时需要做一些额外的处理。
这个程序只是一个基本的框架,你可以根据需要添加其他功能和完善细节。
相关推荐
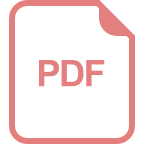
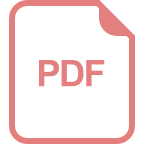








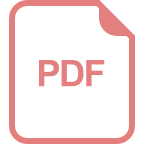
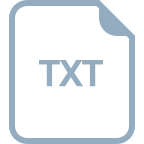
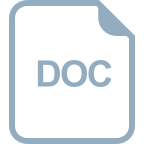
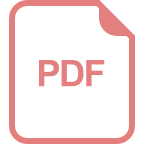
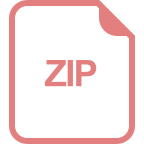
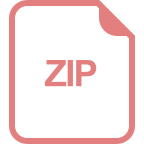