运用C++类的功能编写康威生命游戏代码 规则: 每个细胞处于两种可能状态中的一种,活的或死的 每个细胞与它的8个邻居相互作用: 1. 任何活细胞如果邻居少于两个就会死亡。 2. 任何一个活的细胞,只要有两到三个活的邻居,就会延续到下一代。 3.任何有三个以上邻居的活细胞都会死到下一代。 4. 任何一个死去的细胞只要有恰好三个活着的邻居,就会成为一个活细胞,延续到下一代。 要求: 1.用户能够自定义创建网格大小 2.用户可以在演化进行时暂停和恢复游戏的进程 3.用黑白方块代表细胞的状态 4.先进行随机演化之后在演化过程中可以改变细胞的状态
时间: 2024-03-21 08:38:55 浏览: 92
好的,我会根据您的需求回答问题。以下是一个可能的康威生命游戏的C++类实现:
```cpp
#include <iostream>
#include <vector>
#include <cstdlib>
#include <ctime>
class LifeGame {
public:
LifeGame(int width, int height);
void init();
void run();
void pause();
void resume();
void changeCellState(int x, int y);
void print();
private:
int width_;
int height_;
bool paused_;
std::vector<std::vector<bool>> cells_;
std::vector<std::vector<bool>> nextCells_;
int countLiveNeighbors(int x, int y);
};
LifeGame::LifeGame(int width, int height) {
width_ = width;
height_ = height;
paused_ = false;
cells_ = std::vector<std::vector<bool>>(height, std::vector<bool>(width, false));
nextCells_ = std::vector<std::vector<bool>>(height, std::vector<bool>(width, false));
}
void LifeGame::init() {
// 随机生成初始状态
std::srand(std::time(nullptr));
for (int i = 0; i < height_; ++i) {
for (int j = 0; j < width_; ++j) {
cells_[i][j] = (std::rand() % 2 == 0);
}
}
}
void LifeGame::run() {
while (true) {
if (!paused_) {
// 计算下一个状态
for (int i = 0; i < height_; ++i) {
for (int j = 0; j < width_; ++j) {
int liveNeighbors = countLiveNeighbors(i, j);
if (cells_[i][j]) {
nextCells_[i][j] = (liveNeighbors >= 2 && liveNeighbors <= 3);
} else {
nextCells_[i][j] = (liveNeighbors == 3);
}
}
}
// 更新状态
cells_ = nextCells_;
}
print();
}
}
void LifeGame::pause() {
paused_ = true;
}
void LifeGame::resume() {
paused_ = false;
}
void LifeGame::changeCellState(int x, int y) {
cells_[y][x] = !cells_[y][x];
}
void LifeGame::print() {
system("clear"); // 清除屏幕
for (int i = 0; i < height_; ++i) {
for (int j = 0; j < width_; ++j) {
std::cout << (cells_[i][j] ? "■" : "□");
}
std::cout << std::endl;
}
}
int LifeGame::countLiveNeighbors(int x, int y) {
int count = 0;
for (int i = -1; i <= 1; ++i) {
for (int j = -1; j <= 1; ++j) {
if (i == 0 && j == 0) {
continue;
}
int nx = x + i;
int ny = y + j;
if (nx >= 0 && nx < height_ && ny >= 0 && ny < width_ && cells_[nx][ny]) {
count++;
}
}
}
return count;
}
```
这个类提供了以下功能:
- 构造函数需要传入网格的宽度和高度。
- init() 方法随机生成初始状态。
- run() 方法开始演化过程,每次更新状态并打印出来。
- pause() 和 resume() 方法可以暂停和恢复演化过程。
- changeCellState() 方法可以改变指定细胞的状态。
- print() 方法打印当前状态。
您可以根据需要自定义调用这些方法,实现您想要的康威生命游戏。
阅读全文
相关推荐
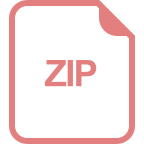
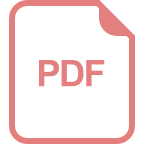
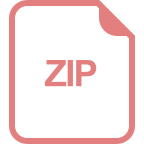

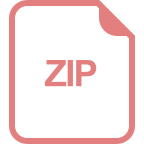
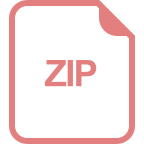
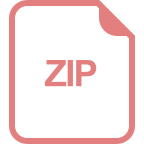
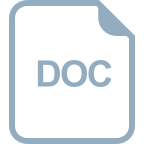
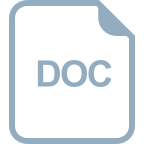
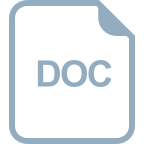
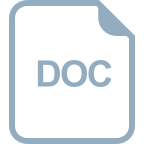


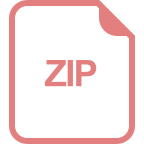