用c++编写康威生命游戏,要求定义class Cell i.每个单元负责为下一代计算其步骤。2.单元格显示其状态,并且能够在运行过程改变一个细胞的生死状态
时间: 2024-02-12 18:08:16 浏览: 26
好的,我会尽力回答你的问题。首先,康威生命游戏是一种模拟细胞自动机的游戏,其中每个细胞有两种状态:生或死。每个细胞的状态取决于其周围八个细胞的状态,具体规则如下:
- 如果一个细胞周围有三个活细胞,则该细胞复活;
- 如果一个细胞周围有两个活细胞,则该细胞保持原样;
- 如果一个细胞周围有少于两个活细胞,则该细胞死亡;
- 如果一个细胞周围有超过三个活细胞,则该细胞死亡。
现在,让我们来看看如何用 C++ 编写康威生命游戏,具体要求如下:
1. 定义 class Cell,每个细胞负责为下一代计算其步骤。
```c++
class Cell {
public:
int x, y; // 细胞的坐标
bool state; // 细胞的状态
bool next_state; // 下一代细胞的状态
Cell(int x, int y, bool state); // 构造函数
void calculate_next_state(Cell** board, int rows, int cols); // 计算下一代细胞状态
void update_state(); // 更新细胞状态
};
```
2. 单元格显示其状态,并且能够在运行过程改变一个细胞的生死状态。
```c++
class Cell {
public:
// ...
void draw(); // 绘制细胞状态
void toggle_state(); // 切换细胞状态
};
```
下面是完整的代码实现:
```c++
#include <iostream>
#include <cstdlib>
#include <ctime>
#include <unistd.h>
class Cell {
public:
int x, y;
bool state;
bool next_state;
Cell(int x, int y, bool state) {
this->x = x;
this->y = y;
this->state = state;
}
void calculate_next_state(Cell** board, int rows, int cols) {
int count = 0;
for (int i = -1; i <= 1; i++) {
for (int j = -1; j <= 1; j++) {
if (i == 0 && j == 0) continue;
int row = x + i;
int col = y + j;
if (row < 0) row = rows - 1;
if (row >= rows) row = 0;
if (col < 0) col = cols - 1;
if (col >= cols) col = 0;
if (board[row][col].state) count++;
}
}
if (count == 3) {
next_state = true;
} else if (count == 2) {
next_state = state;
} else {
next_state = false;
}
}
void update_state() {
state = next_state;
}
void draw() {
if (state) {
std::cout << "O";
} else {
std::cout << ".";
}
}
void toggle_state() {
state = !state;
}
};
class GameOfLife {
public:
int rows, cols;
Cell** board;
GameOfLife(int rows, int cols) {
this->rows = rows;
this->cols = cols;
board = new Cell*[rows];
for (int i = 0; i < rows; i++) {
board[i] = new Cell[cols];
for (int j = 0; j < cols; j++) {
board[i][j] = Cell(i, j, false);
}
}
}
~GameOfLife() {
for (int i = 0; i < rows; i++) {
delete[] board[i];
}
delete[] board;
}
void randomize() {
srand(time(NULL));
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
board[i][j].state = rand() % 2 == 0;
}
}
}
void draw() {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
board[i][j].draw();
}
std::cout << std::endl;
}
}
void update() {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
board[i][j].calculate_next_state(board, rows, cols);
}
}
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
board[i][j].update_state();
}
}
}
};
int main() {
GameOfLife game(10, 10);
game.randomize();
while (true) {
game.draw();
game.update();
usleep(100000);
system("clear");
}
return 0;
}
```
在上面的代码中,我们定义了一个 `GameOfLife` 类,用于管理整个游戏的逻辑。我们还实现了 `randomize` 函数,用于随机生成初始细胞状态;`draw` 函数,用于绘制细胞状态;`update` 函数,用于更新细胞状态。此外,我们还实现了一个简单的游戏循环,以便在终端上运行游戏。
相关推荐
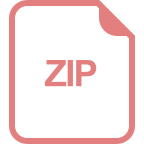
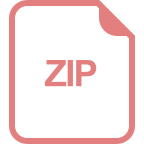
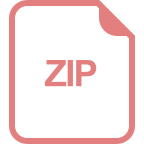










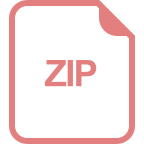
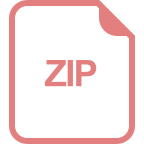
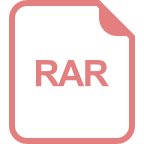