用c++编写康威生命游戏,要求定义class Cell,并且用户可以在运行时更改单元格的状态。模拟可以回滚(恢复之前状态)
时间: 2024-03-10 15:45:24 浏览: 58
好的,我将为您提供一个C++康威生命游戏的实现。首先,我们需要定义一个Cell类,用来表示每个细胞的状态。
```cpp
class Cell {
public:
enum State {
DEAD,
ALIVE
};
Cell(State state = DEAD) : m_state(state), m_prevState(state) {}
void setState(State state) {
m_prevState = m_state;
m_state = state;
}
void rollbackState() {
m_state = m_prevState;
}
bool isAlive() const {
return m_state == ALIVE;
}
private:
State m_state;
State m_prevState;
};
```
在这个类中,我们定义了两个状态:DEAD(死亡)和ALIVE(存活)。类中包含了当前状态和上一个状态的变量,以及相应的设置状态和回滚状态的函数。
接下来,我们需要实现康威生命游戏的主体逻辑。我们可以定义一个Board类来表示整个游戏的状态。
```cpp
class Board {
public:
Board(int rows, int cols) : m_rows(rows), m_cols(cols) {
m_cells = new Cell*[m_rows];
for (int i = 0; i < m_rows; ++i) {
m_cells[i] = new Cell[m_cols];
}
}
~Board() {
for (int i = 0; i < m_rows; ++i) {
delete[] m_cells[i];
}
delete[] m_cells;
}
void setCellState(int row, int col, Cell::State state) {
m_cells[row][col].setState(state);
}
void rollbackCellState(int row, int col) {
m_cells[row][col].rollbackState();
}
Cell::State getCellState(int row, int col) const {
return m_cells[row][col].isAlive() ? Cell::ALIVE : Cell::DEAD;
}
void update() {
Board temp(m_rows, m_cols);
for (int i = 0; i < m_rows; ++i) {
for (int j = 0; j < m_cols; ++j) {
int count = countAliveNeighbors(i, j);
if (m_cells[i][j].isAlive()) {
if (count < 2 || count > 3) {
temp.setCellState(i, j, Cell::DEAD);
} else {
temp.setCellState(i, j, Cell::ALIVE);
}
} else {
if (count == 3) {
temp.setCellState(i, j, Cell::ALIVE);
}
}
}
}
swap(temp);
}
private:
void swap(Board& other) {
std::swap(m_rows, other.m_rows);
std::swap(m_cols, other.m_cols);
std::swap(m_cells, other.m_cells);
}
int countAliveNeighbors(int row, int col) const {
int count = 0;
for (int i = -1; i <= 1; ++i) {
for (int j = -1; j <= 1; ++j) {
if (i == 0 && j == 0) {
continue;
}
int r = row + i;
int c = col + j;
if (r >= 0 && r < m_rows && c >= 0 && c < m_cols) {
if (m_cells[r][c].isAlive()) {
++count;
}
}
}
}
return count;
}
int m_rows;
int m_cols;
Cell** m_cells;
};
```
在这个类中,我们定义了一个二维数组来存储每个细胞的状态。类中包含了设置和获取单元格状态的函数,以及更新整个游戏状态的函数。在更新游戏状态时,我们使用了一个临时的Board对象来保存更新后的状态,并最终将其替换为当前状态。
最后,我们可以在主函数中使用这些类来模拟康威生命游戏。
```cpp
int main() {
Board board(10, 10);
// 初始化一些细胞状态
board.setCellState(5, 5, Cell::ALIVE);
board.setCellState(5, 6, Cell::ALIVE);
board.setCellState(5, 7, Cell::ALIVE);
// 模拟游戏几次
board.update();
board.update();
board.update();
// 更改单元格状态
board.setCellState(4, 5, Cell::ALIVE);
// 恢复之前状态
board.rollbackCellState(4, 5);
return 0;
}
```
在这个例子中,我们初始化了一些细胞状态,并模拟了游戏几次。然后,我们更改了一个单元格的状态,并使用rollbackCellState函数将其恢复到之前的状态。
阅读全文
相关推荐
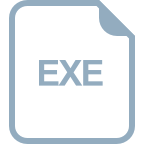
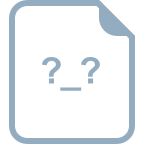





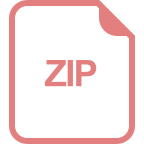
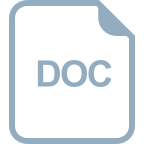
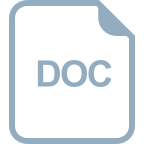
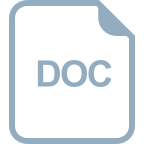
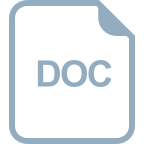
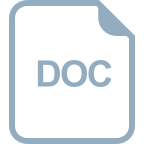
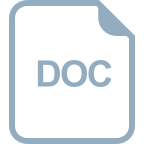
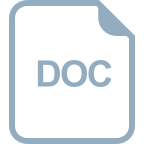


