使用java代码实现 PolyLine 类包含-一个Line数组(折线是由一些首尾相连的线段组合而成),还包含计算折线总长度的方法(调用Line中计算线段长度的方法)、折线平移的方法(调用线段的平移方法)←
时间: 2024-05-15 14:18:38 浏览: 156
好的,以下是使用Java代码实现PolyLine类的示例:
```java
public class PolyLine {
private Line[] lines;
public PolyLine(Line[] lines) {
this.lines = lines;
}
public double getLength() {
double length = 0;
for (Line line : lines) {
length += line.getLength();
}
return length;
}
public void translate(double dx, double dy) {
for (Line line : lines) {
line.translate(dx, dy);
}
}
}
```
在上面的示例中,我们定义了PolyLine类,其中包含一个Line数组和两个方法:getLength和translate。getLength方法遍历每条线段并调用其getLength方法,最终返回折线的总长度。translate方法遍历每条线段并调用其translate方法,将折线平移指定的距离。注意,我们假设Line类已经实现了getLength和translate方法。
相关问题
C++定义折线类(polyline),成员变量为point指针数组(长度5),定义构造函数,输入各点的x,y坐标数据,new动态创建point对象,增加成员函数,计算折线的长度(各线段长度之和),定义析构函数,delete各点;
好的,我来帮您解答这个问题。
以下是您所需的折线类的定义:
```cpp
class Polyline {
private:
Point* point[5];
public:
Polyline(double x[], double y[]);
~Polyline();
double length();
};
```
其中,`Point` 类表示点,您需要先定义好。
在构造函数中,我们需要使用 `new` 关键字动态创建 `Point` 对象,并将其存储在 `point` 数组中:
```cpp
Polyline::Polyline(double x[], double y[]) {
for (int i=0; i<5; i++) {
point[i] = new Point(x[i], y[i]);
}
}
```
在计算折线长度时,我们需要计算各线段长度之和。可以通过循环遍历 `point` 数组来实现:
```cpp
double Polyline::length() {
double len = 0;
for (int i=0; i<4; i++) {
len += point[i]->distanceTo(*point[i+1]);
}
return len;
}
```
在析构函数中,我们需要使用 `delete` 关键字释放 `new` 出来的内存:
```cpp
Polyline::~Polyline() {
for (int i=0; i<5; i++) {
delete point[i];
}
}
```
这样,您就完成了折线类的定义。
vue使用el-amap手动绘制折线方法
在 Vue 中使用 `el-amap` 组件库手动绘制折线,可以通过引入其中的 `AmapManager` 组件来实现。以下是一个简单的实现方法:
1. 安装 `el-amap` 组件库:
```
npm install el-amap --save
```
2. 在 Vue 中引入 `AmapManager` 组件:
```vue
<template>
<el-amap class="map-container">
<el-amap-manager :plugin="plugin"></el-amap-manager>
<el-button @click="drawPolyline">绘制折线</el-button>
</el-amap>
</template>
<script>
import { AmapManager } from 'el-amap';
export default {
components: {
AmapManager,
},
data() {
return {
plugin: ['AMap.PolyEditor'],
polyline: null,
};
},
methods: {
drawPolyline() {
const editor = new AMap.PolyEditor(this.map, this.polyline);
editor.open();
editor.on('end', () => {
const path = this.polyline.getPath();
console.log('绘制完成', path);
// 处理绘制结果
});
},
},
};
</script>
```
3. 在 Vue 中创建地图实例和折线实例:
```vue
<script>
import { AmapManager } from 'el-amap';
export default {
components: {
AmapManager,
},
data() {
return {
plugin: ['AMap.PolyEditor'],
mapOptions: {
center: [116.397428, 39.90923],
zoom: 13,
},
polylineOptions: {
path: [],
strokeColor: '#3366FF',
strokeWeight: 5,
},
polyline: null,
};
},
mounted() {
this.polyline = new AMap.Polyline(this.polylineOptions);
this.polyline.setMap(this.$amap.getMap());
this.$amap.plugin('AMap.MouseTool', () => {
const tool = new AMap.MouseTool(this.$amap.getMap());
tool.on('draw', (e) => {
const path = e.obj.getPath();
console.log('绘制完成', path);
// 处理绘制结果
this.polyline.setPath(path);
this.polyline.setMap(this.$amap.getMap());
});
});
},
methods: {
drawPolyline() {
const tool = new AMap.MouseTool(this.$amap.getMap());
tool.polyline();
},
},
};
</script>
```
以上是一个简单的实现方法,你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
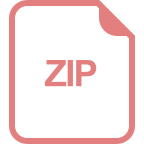
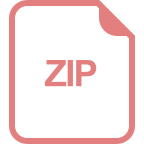
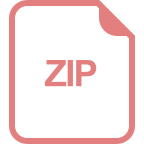
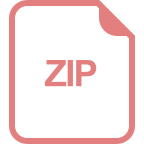
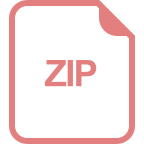
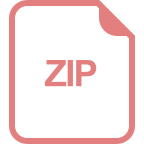
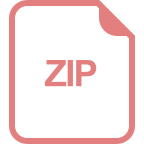
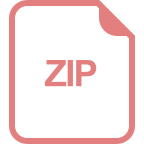
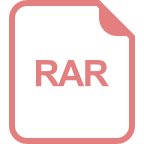
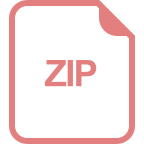
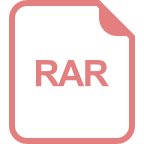
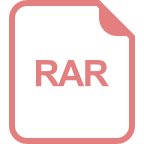
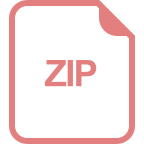
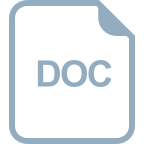
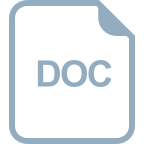
