python matplotlib axis
时间: 2023-08-27 14:22:52 浏览: 46
在 Matplotlib 中,`axis()` 函数用于设置坐标轴的可见性和刻度范围。
下面是一个示例代码,演示如何使用 `axis()` 函数:
```python
import matplotlib.pyplot as plt
# 创建 x 和 y 坐标的列表
x = [1, 2, 3, 4, 5]
y = [1, 3, 2, 4, 5]
# 创建一个 Figure 对象和一个 Axes 对象
fig, ax = plt.subplots()
# 绘制折线图
ax.plot(x, y)
# 设置 x 和 y 坐标轴的可见性和刻度范围
ax.axis([0, 6, 0, 6]) # 设置 x 和 y 坐标轴的刻度范围为 0 到 6,并将坐标轴设置为可见
# 显示图形
plt.show()
```
在这个示例中,我们使用 `axis([x_min, x_max, y_min, y_max])` 方法来设置 x 和 y 坐标轴的刻度范围,并将坐标轴设置为可见。你可以根据自己的需要调整刻度范围和其他坐标轴属性。
相关问题
pythonmatplotlib
Python中的Matplotlib是一个用于绘制二维图形的库。它提供了许多绘图选项,可以创建各种类型的图表,包括线图、散点图、柱状图、饼图等等。
要使用Matplotlib,首先需要安装它。可以使用pip命令在命令行中安装Matplotlib:
```
pip install matplotlib
```
安装完成后,就可以在Python脚本中引入Matplotlib库并开始绘图了。以下是一个简单的例子,演示如何使用Matplotlib绘制一个简单的折线图:
```python
import matplotlib.pyplot as plt
# 创建数据
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
# 绘制折线图
plt.plot(x, y)
# 添加标题和标签
plt.title("Simple Line Plot")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
# 显示图形
plt.show()
```
执行以上代码,将会弹出一个窗口显示绘制的折线图。这只是一个简单的例子,Matplotlib还有很多其他功能和选项,可以根据需要进行进一步的定制和调整。你可以参考Matplotlib的官方文档来获取更多信息和示例:[https://matplotlib.org/](https://matplotlib.org/)
python matplotlib实例
下面是一个简单的 Python Matplotlib 示例,绘制了一个折线图:
```python
import matplotlib.pyplot as plt
# 准备数据
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
# 创建图表
plt.plot(x, y)
# 添加标题和坐标轴标签
plt.title("Simple Line Plot")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
# 显示图表
plt.show()
```
在这个示例中,我们首先导入了 Matplotlib 中的 `pyplot` 模块,并使用 `plot` 函数绘制了 x 和 y 之间的折线图。我们还使用了 `title`,`xlabel` 和 `ylabel` 函数添加了图表的标题和坐标轴标签。最后,我们使用 `show` 函数显示了图表。
相关推荐
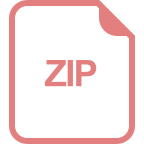
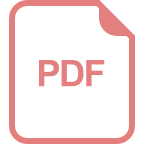
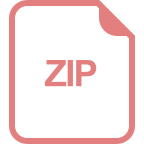









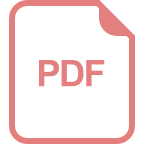
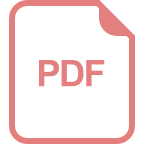
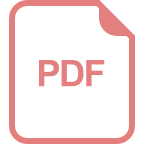
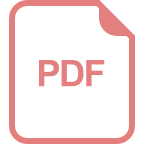