帮我用python写一个计算24点的小程序,它的原理是在1~9之间随机生成4个数,他们经过加减乘除运算一定能等于24(不考虑带括号的情况),然后把这些数显示在GUI界面,用户可以通过键盘的光标键改变运算符使等式成立。 使用Python GUI编程实现该程序。
时间: 2024-03-19 18:43:19 浏览: 31
好的,这里给出一个Python的GUI程序,实现计算24点的小游戏。
```python
import random
import tkinter as tk
class Game24:
def __init__(self):
self.window = tk.Tk()
self.window.title("计算24点")
self.window.geometry("300x200")
self.numbers = [random.randint(1, 9) for _ in range(4)]
self.equation = tk.StringVar()
self.equation.set(self.get_equation())
self.label = tk.Label(self.window, textvariable=self.equation, font=("Arial", 18))
self.label.pack(pady=10)
self.operators = ["+", "-", "*", "/"]
self.current_operator = tk.StringVar()
self.current_operator.set(self.operators[0])
self.operator_label = tk.Label(self.window, textvariable=self.current_operator, font=("Arial", 18))
self.operator_label.pack(pady=10)
self.window.bind("<Left>", self.change_operator)
self.window.bind("<Right>", self.change_operator)
self.answer_entry = tk.Entry(self.window, font=("Arial", 18))
self.answer_entry.pack(pady=10)
self.check_button = tk.Button(self.window, text="检查答案", font=("Arial", 12), command=self.check_answer)
self.check_button.pack(pady=10)
self.new_game_button = tk.Button(self.window, text="开始新游戏", font=("Arial", 12), command=self.start_new_game)
self.new_game_button.pack(pady=10)
self.window.mainloop()
def get_equation(self):
equation = str(self.numbers[0])
for i in range(3):
equation += " " + self.operators[random.randint(0, 3)] + " " + str(self.numbers[i + 1])
return equation
def change_operator(self, event):
if event.keysym == "Left":
index = (self.operators.index(self.current_operator.get()) - 1) % 4
elif event.keysym == "Right":
index = (self.operators.index(self.current_operator.get()) + 1) % 4
self.current_operator.set(self.operators[index])
def check_answer(self):
answer = self.answer_entry.get()
try:
result = eval(self.equation.get().replace(self.current_operator.get(), answer))
if result == 24:
tk.messagebox.showinfo("恭喜", "答案正确!")
else:
tk.messagebox.showerror("很遗憾", "答案错误!")
except:
tk.messagebox.showerror("错误", "输入无效!")
def start_new_game(self):
self.numbers = [random.randint(1, 9) for _ in range(4)]
self.equation.set(self.get_equation())
self.current_operator.set(self.operators[0])
self.answer_entry.delete(0, tk.END)
if __name__ == "__main__":
game = Game24()
```
程序会生成一个Tkinter窗口,并在窗口中显示出等式和答案输入框。用户可以通过点击“开始新游戏”按钮来开始新的游戏,程序会重新生成四个随机数和一个等式。用户可以通过键盘的左右光标键来改变等式中的运算符,然后在答案输入框中输入答案,点击“检查答案”按钮来检查答案是否正确。
相关推荐
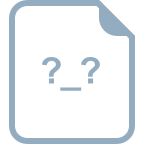
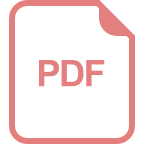
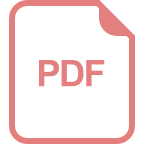














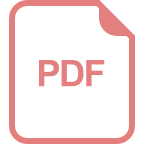