GeometrySplitter的例子
时间: 2024-01-20 12:04:02 浏览: 56
以下是一个使用GeometrySplitter类的简单示例:
```cpp
#include <osg/Geometry>
#include <osg/Geode>
#include <osgUtil/GeometrySplitter>
osg::ref_ptr<osg::Node> createGeometry()
{
osg::ref_ptr<osg::Geometry> geom = new osg::Geometry;
// 添加顶点数据
osg::ref_ptr<osg::Vec3Array> vertices = new osg::Vec3Array;
vertices->push_back(osg::Vec3(0.0f, 0.0f, 0.0f));
vertices->push_back(osg::Vec3(1.0f, 0.0f, 0.0f));
vertices->push_back(osg::Vec3(1.0f, 1.0f, 0.0f));
vertices->push_back(osg::Vec3(0.0f, 1.0f, 0.0f));
geom->setVertexArray(vertices.get());
// 添加法线数据
osg::ref_ptr<osg::Vec3Array> normals = new osg::Vec3Array;
normals->push_back(osg::Vec3(0.0f, 0.0f, 1.0f));
geom->setNormalArray(normals.get());
geom->setNormalBinding(osg::Geometry::BIND_OVERALL);
// 添加纹理坐标数据
osg::ref_ptr<osg::Vec2Array> texcoords = new osg::Vec2Array;
texcoords->push_back(osg::Vec2(0.0f, 0.0f));
texcoords->push_back(osg::Vec2(1.0f, 0.0f));
texcoords->push_back(osg::Vec2(1.0f, 1.0f));
texcoords->push_back(osg::Vec2(0.0f, 1.0f));
geom->setTexCoordArray(0, texcoords.get());
// 设置绘制模式
geom->addPrimitiveSet(new osg::DrawArrays(GL_QUADS, 0, 4));
osg::ref_ptr<osg::Geode> geode = new osg::Geode;
geode->addDrawable(geom.get());
// 将几何体划分为子几何体
osgUtil::GeometrySplitter splitter;
splitter.splitByMaximumTriangleCount(geode.get(), 3);
return geode.get();
}
```
在上面的示例中,我们首先创建了一个包含四个顶点的矩形几何体,然后将其添加到一个Geode节点中。接着,我们使用GeometrySplitter类将该几何体划分为最多包含三个三角形的子几何体。最后返回Geode节点作为示例的结果。
需要注意的是,该示例中的划分算法为splitByMaximumTriangleCount,它将尽可能均匀地将几何体划分为多个子几何体,以便于同时渲染和优化性能。
阅读全文
相关推荐
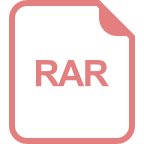



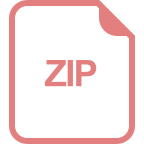
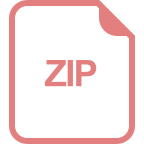
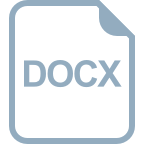
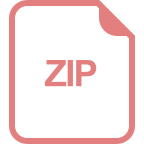
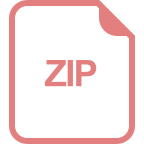
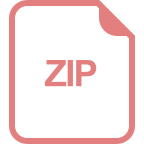