img should be PIL Image. Got <class 'numpy.ndarray'>
时间: 2024-03-06 16:50:38 浏览: 175
这个错误通常是因为使用了numpy数组代替了PIL Image对象。你可以将numpy数组转换为PIL Image对象,然后再进行操作。可以使用以下代码将numpy数组转换为PIL Image对象:
``` python
from PIL import Image
import numpy as np
# 将numpy数组转换为PIL Image对象
img = Image.fromarray(np.uint8(numpy_array))
```
在这里,`numpy_array`是你的numpy数组。通过这种方式将numpy数组转换为PIL Image对象后,就可以在不出现错误的情况下进行操作了。
相关问题
TypeError: img should be PIL Image. Got <class 'torchvision.transforms.transforms.RandomHorizontalFlip'>
### 回答1:
这个错误消息表明在代码中使用了一个错误类型的变量。在这种情况下,应该使用 PIL 图像 (PIL Image),但实际上使用的是 torchvision.transforms.transforms.RandomHorizontalFlip 类。因此,应该检查代码并确保正确使用 PIL 图像。
### 回答2:
这个错误提示是由torchvision库中的transforms模块引起的。它表明在使用transforms模块的RandomHorizontalFlip函数时,传入的参数类型应为PIL Image,但实际传入了torchvision.transforms.transforms.RandomHorizontalFlip类的对象。
解决这个问题的方法是将原始输入转换为PIL Image类型。可以通过以下步骤来实现:
1. 首先,确认输入的数据是一个PIL Image对象。可以使用PIL库中的Image类将其转换为PIL Image对象。例如,如果输入的是一个numpy数组,可以使用Image.fromarray()函数将其转换为PIL Image对象。
2. 进一步,确保传递给RandomHorizontalFlip的参数是PIL Image对象。如果之前的步骤已经将数据转换为PIL Image对象,那么传递给RandomHorizontalFlip的参数应该是这个PIL Image对象。如果不是,可以使用之前提到的Image.fromarray()函数将数据转换为PIL Image对象。
总结起来,解决这个错误的关键步骤是将输入的数据转换为PIL Image对象,并确保传递给RandomHorizontalFlip的参数是PIL Image对象。这样就可以避免出现TypeError: img should be PIL Image. Got <class 'torchvision.transforms.transforms.RandomHorizontalFlip'>的错误。
### 回答3:
这个错误是由于在调用函数时传入了错误的数据类型导致的。具体来说,你传入了一个torchvision.transforms.transforms.RandomHorizontalFlip对象作为参数,而实际上该函数要求传入一个PIL图像对象。
要解决这个问题,你需要将随机水平翻转对象转换为PIL图像对象,然后再将其传入函数。你可以使用该对象的`__call__`方法来实现转换。具体代码如下:
```python
from torchvision.transforms import transforms
from PIL import Image
# 创建一个随机水平翻转对象
flip = transforms.RandomHorizontalFlip()
# 创建一个示例图像
image_path = 'example.jpg'
image = Image.open(image_path)
# 将随机翻转对象转换为PIL图像对象
flipped_image = flip(image)
# 现在可以将flipped_image传入需要接受PIL图像的函数了
```
通过这种方式,你可以将随机水平翻转对象转换为PIL图像对象,从而避免了TypeError错误。希望这个解答能帮到你!
TypeError: pic should be Tensor or ndarray. Got <class 'PIL.Image.Image'>.
This error occurs when you are trying to pass a PIL Image object to a function or method that expects a Tensor or ndarray object.
To resolve this error, you can convert the PIL Image object to a Tensor or ndarray using the appropriate function. For example, if you are using PyTorch, you can use the torchvision.transforms.ToTensor() function to convert the PIL Image object to a Tensor.
Here is an example code snippet:
```
import torch
import torchvision.transforms as transforms
from PIL import Image
# Load the image using PIL
img = Image.open('image.jpg')
# Convert the image to a Tensor
tensor_img = transforms.ToTensor()(img)
# Pass the Tensor to the function/method that expects it
```
In this example, we first load the image using PIL, then convert it to a Tensor using the ToTensor() function from torchvision.transforms module. We can then pass the Tensor object to the function or method that expects it.
阅读全文
相关推荐
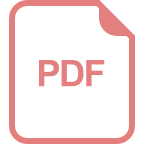
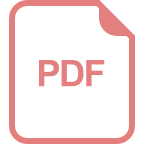














