写一个C++程序,实现以下功能利用stack容器判断括号表达式是否合法,即左右括号需匹配。如果表达式正确,输出true,如果表达式错误,输出false。 Sample Input: 3+((a+b)+(c*d)) ( Sample Output: false Sample Input: 3+((a+b)+(c*d)) Sample Output: true
时间: 2024-03-24 07:37:18 浏览: 69
下面是一个基于stack容器的C++程序,实现括号表达式的匹配检查:
```c++
#include <iostream>
#include <stack>
#include <string>
using namespace std;
// 判断左右括号是否匹配
bool isMatch(char left, char right) {
if (left == '(' && right == ')') {
return true;
} else if (left == '{' && right == '}') {
return true;
} else if (left == '[' && right == ']') {
return true;
}
return false;
}
// 判断括号表达式是否合法
bool isValid(string s) {
stack<char> stk;
for (char c : s) {
if (c == '(' || c == '{' || c == '[') {
stk.push(c);
} else if (c == ')' || c == '}' || c == ']') {
if (stk.empty() || !isMatch(stk.top(), c)) {
return false;
}
stk.pop();
}
}
return stk.empty();
}
int main() {
string s;
cin >> s;
if (isValid(s)) {
cout << "true" << endl;
} else {
cout << "false" << endl;
}
return 0;
}
```
该程序首先定义了一个辅助函数`isMatch()`用于判断左右括号是否匹配。然后定义了`isValid()`函数用于判断括号表达式是否合法,该函数使用了一个stack容器来存储左括号,并在遇到右括号时弹出相应的左括号进行匹配检查。最后在主函数中读入表达式并输出检查结果。
阅读全文
相关推荐
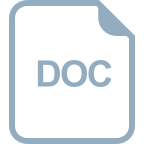
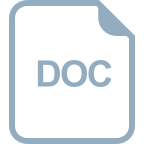
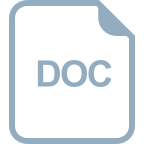



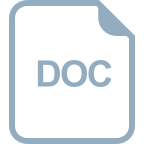











