def aesEncrypt(self, text, secKey): pad = 16 - len(text) % 16 text = text + pad * chr(pad) encryptor = AES.new(secKey.encode('utf-8'), 2, '0102030405060708'.encode('utf-8')) ciphertext = encryptor.encrypt(text.encode('utf-8')) ciphertext = base64.b64encode(ciphertext).decode("utf-8") return ciphertext
时间: 2024-03-19 10:42:37 浏览: 28
这段代码是用于对文本进行AES加密的Python函数。它接受两个参数,分别为待加密的文本和密钥。在函数内部,首先根据文本长度计算出需要填充的字节数,然后在文本末尾填充相应数量的字符,使其长度为16的倍数。接下来,使用给定的密钥对文本进行AES加密,并将密文进行Base64编码后返回。需要注意的是,该函数中使用的AES加密模式为CBC,初始向量固定为'0102030405060708'。
相关问题
翻译def pad(self, s): return s + (AES.block_size - len(s) % AES.block_size) * chr(AES.block_size - len(s) % AES.block_size)
翻译如下:
```python
def pad(self, s):
return s + (AES.block_size - len(s) % AES.block_size) * chr(AES.block_size - len(s) % AES.block_size)
```
这段代码是一个Python类中的方法,用于对输入的字符串进行填充。在这里,方法首先计算需要填充的字节数,使用AES.block_size减去字符串长度除以AES.block_size取余得到。然后,方法将需要填充的字符(chr函数返回一个ASCII字符)重复相应次数并连接到原始字符串的末尾,以实现填充。最后,方法返回填充后的字符串。
python实现AES算法CBC模式加解密
好的,以下是Python实现AES算法CBC模式加解密的代码:
```python
from Crypto.Cipher import AES
import base64
BS = 16
pad = lambda s: s + (BS - len(s) % BS) * chr(BS - len(s) % BS)
unpad = lambda s: s[:-ord(s[len(s)-1:])]
class AESCipher:
def __init__(self, key):
self.key = key.encode('utf-8')
def encrypt(self, raw):
raw = pad(raw).encode('utf-8')
iv = b'0000000000000000'
cipher = AES.new(self.key, AES.MODE_CBC, iv)
return base64.b64encode(cipher.encrypt(raw)).decode('utf-8')
def decrypt(self, enc):
enc = base64.b64decode(enc.encode('utf-8'))
iv = b'0000000000000000'
cipher = AES.new(self.key, AES.MODE_CBC, iv)
return unpad(cipher.decrypt(enc)).decode('utf-8')
```
使用方法示例:
```python
key = '1234567890123456'
cipher = AESCipher(key)
# 加密
encrypted = cipher.encrypt('hello world')
print(encrypted)
# 解密
decrypted = cipher.decrypt(encrypted)
print(decrypted)
```
注意事项:
1. key的长度必须为16、24、32位,分别对应AES-128、AES-192、AES-256加密算法。
2. 由于CBC模式需要一个初始向量IV,这里默认使用16个0。实际使用中,IV应该是随机的,并且需要和密文一起传输。
阅读全文
相关推荐
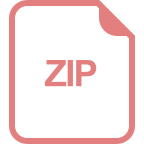
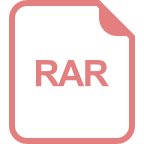
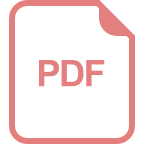
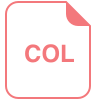
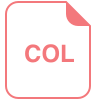




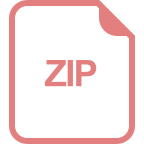
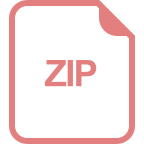
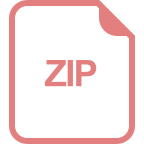
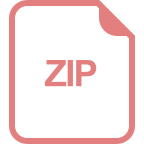
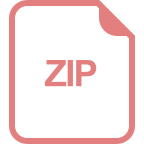