用csv文件保存五个学生课程成绩,用python编写一个程序,从文件读取成绩,计算总分,按总分从高到低输出
时间: 2024-09-08 10:04:47 浏览: 113
为了用Python编写一个程序,读取CSV文件中的学生成绩并按照总分排序,我们可以遵循以下步骤:
首先,假设CSV文件的结构如下:
```
Name, Course1, Course2, Course3, Course4, Course5
张三, 85, 90, 80, 95, 88
李四, 92, 88, 93, 86, 90
王五, 78, 85, 75, 90, 82
赵六, 87, 91, 82, 92, 85
孙七, 90, 93, 87, 88, 91
```
你可以使用pandas库来轻松处理这个任务。以下是完整的代码示例:
```python
import pandas as pd
# 读取CSV文件
data = pd.read_csv('students_scores.csv')
# 将分数列相加得到总分
data['Total Score'] = data.iloc[:, 1:].sum(axis=1)
# 按照总分降序排序
sorted_data = data.sort_values('Total Score', ascending=False)
# 输出结果
for index, row in sorted_data.iterrows():
print(f"{row['Name']}: 总分={row['Total Score']}")
#
相关问题
分别用文本文件和CSV文件保存5个学生的课程成绩。编写一个程序,从文件读取成绩,计算总分,按总分按从高到低的顺序输出。
以下是Python代码示例:
使用文本文件保存成绩:
```python
# 读取文本文件
with open('scores.txt', 'r') as f:
lines = f.readlines()
scores = {}
# 解析数据
for line in lines:
items = line.strip().split(',')
name = items[0]
score = list(map(int, items[1:]))
scores[name] = score
# 计算总分
total_scores = {}
for name, score in scores.items():
total_scores[name] = sum(score)
# 排序并输出
sorted_scores = sorted(total_scores.items(), key=lambda x: x[1], reverse=True)
for name, total_score in sorted_scores:
print(f'{name}: {total_score}')
```
使用CSV文件保存成绩:
```python
import csv
# 读取CSV文件
with open('scores.csv', 'r') as f:
reader = csv.reader(f)
header = next(reader)
scores = {}
for row in reader:
name = row[0]
score = list(map(int, row[1:]))
scores[name] = score
# 计算总分
total_scores = {}
for name, score in scores.items():
total_scores[name] = sum(score)
# 排序并输出
sorted_scores = sorted(total_scores.items(), key=lambda x: x[1], reverse=True)
for name, total_score in sorted_scores:
print(f'{name}: {total_score}')
```
注意:在使用CSV文件时,需要使用Python标准库中的csv模块来读取文件。并且,第一行通常是标题行,需要跳过。
Python编写程序,读取CSV文件,计算每个学生的总分和平均分。
好的,可以使用Python内置的csv模块来读取CSV文件,然后进行计算。以下是示例代码:
```python
import csv
filename = "grades.csv" # CSV文件名
# 读取CSV文件
with open(filename, 'r') as file:
reader = csv.reader(file)
header = next(reader) # 获取表头
data = [row for row in reader] # 获取数据
# 计算每个学生的总分和平均分
for row in data:
total_score = sum(map(int, row[1:])) # 计算总分
avg_score = total_score / len(row[1:]) # 计算平均分
print("学生{}的总分为{},平均分为{}".format(row[0], total_score, avg_score))
```
在上述代码中,我们首先打开CSV文件并读取其中的数据,然后对于每一行数据,计算总分和平均分,并输出结果。其中,使用了`sum`函数和`map`函数来计算总分,`len`函数用来获取每个学生的科目数。你可以根据自己的CSV文件格式进行适当的修改。
阅读全文
相关推荐
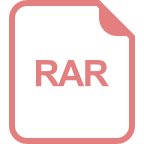
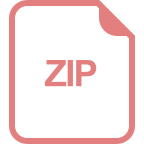
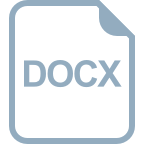













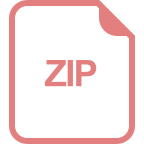