lua continue
时间: 2023-11-03 14:07:10 浏览: 54
Lua中没有内置的continue关键字,但是可以通过一些技巧来实现continue的功能。常见的方法有三种:
1. 在循环中再定义一个循环,并且这个循环只执行一次。
2. 利用for循环的break关键字实现。
3. 利用goto语句实现。
其中第一种方法需要在循环中再定义一个循环,并且这个循环只执行一次,通过在内层循环中使用break来实现continue的功能。第二种方法是在循环中使用while循环,通过在while循环中使用break来实现continue的功能。第三种方法是利用goto语句,在循环中使用goto continue来跳过本次循环,并且可以正常使用break功能。
需要注意的是,虽然这些方法可以实现continue的功能,但是在实际编程中应该尽量避免使用这些技巧,因为它们会使代码变得难以理解和维护。
相关问题
lua http 源码
以下是 Lua 5.1 版本的 http 模块源码,可以用来实现 HTTP 客户端请求:
```lua
-----------------------------------------------------------------------------
-- LuaSocket module for HTTP/1.1 client support
-- Diego Nehab, et al.
-- 04/11/2007
-----------------------------------------------------------------------------
local base = _G
local string = require("string")
local math = require("math")
local socket = require("socket")
local url = require("socket.url")
module("socket.http")
-- the default user agent string
USERAGENT = "LuaSocket 2.0.2"
-- timeout values
TIMEOUT = 60
TIMEOUT_CONNECT = socket._TIMEOUT
-- code 204 is no content, but the server sent a response header
-- code 304 is not modified, so there's no need to resend the content
-- code 205 is a reset content request, we must accept the new content
-- code 206 is a partial content response, we must adjust our range
-- code 301 is a redirect, we must look for the location header
-- code 302 is a redirect, but some buggy servers send content along
-- code 303 is a redirect, but with a get method
-- code 307 is a redirect, but we must keep the method
-- code 401 is a authentication request, we must resend with proper creds
-- code 407 is a proxy authentication request, same as 401
NOCONTENT_CODES = "204 304 205 206"
REDIRECT_CODES = "301 302 303 307"
AUTHREQUIRED_CODES = "401 407"
DEFAULT_REDIRECT_TIMES = 5
-- the default port for each protocol
PORT = { http = 80, https = 443, }
-- get a connection object
local function get_connection(u, redir)
local proxy = base._PROXY or socket._PROXY
local conn = {
try = socket.tcp(),
proxy = proxy and url.parse(proxy),
ssl = u.scheme == "https",
live = true,
redirected = false,
redirectcount = 0,
redirectstring = "",
host = u.host,
port = u.port or PORT[u.scheme],
method = "GET",
url = u,
sink = nil,
headers = {
["user-agent"] = USERAGENT,
["host"] = u.host,
},
source = socket.source("close-when-done", conn.try)
}
if conn.proxy then
conn.host = conn.proxy.host
conn.port = conn.proxy.port
conn.headers["host"] = u.authority
end
if redir then
conn.redirected = true
conn.redirectcount = redir.redirectcount + 1
conn.redirectstring = redir.redirectstring.."\n"..u.request
end
return conn
end
-- close a connection
local function close_connection(c)
if c.try then c.try:close() end
c.try = nil
c.live = nil
end
-- receive a line from a connection or a sink
local function receive(fd, pat, t)
local st, chunk
local buffer = {}
local receive_chunk = fd.receive or fd.read or fd
t = t or TIMEOUT
repeat
st, chunk = receive_chunk(fd, pat)
if st then
buffer[#buffer + 1] = chunk
else
return nil, chunk
end
until string.find(buffer[#buffer] or "", pat, nil, true) or st == nil
return table.concat(buffer)
end
-- send data through a connection or a source
local function send(fd, data)
if not fd.send then
if type(fd) ~= "function" then
error("invalid send source")
end
fd(data)
else
fd:send(data)
end
end
-- convert headers to a string
local function headers_to_string(headers)
local buffer = {}
for field, value in pairs(headers) do
buffer[#buffer + 1] = string.format("%s: %s", field, value)
end
buffer[#buffer + 1] = ""
return table.concat(buffer, "\r\n")
end
-- convert headers from a string to a table
local function headers_from_string(header_string)
local headers = {}
local pos = 1
local eol = string.find(header_string, "\n", pos, true)
while eol do
local line = string.sub(header_string, pos, eol - 1)
line = string.gsub(line, "[\r\n]+$", "")
pos = eol + 1
eol = string.find(header_string, "\n", pos, true)
if line ~= "" then
local field, value = string.match(line, "^(.-):%s*(.*)$")
if field then
field = string.lower(field)
if headers[field] then
headers[field] = headers[field]..", "..value
else
headers[field] = value
end
end
else
break
end
end
return headers
end
-- perform a generic HTTP request
local function request(req)
local u = url.parse(req.url)
local c = get_connection(u, req.redirection)
if not c.try then return nil, "unable to connect to "..u.host end
c.try:settimeout(TIMEOUT_CONNECT, "t")
local res = { }
local pat = "^(.-)\r?\n"
-- send request line
local reqline = string.format("%s %s HTTP/1.1", req.method, u.path or "/")
if u.query then reqline = reqline.."?"..u.query end
send(c.try, string.format("%s\r\n", reqline))
-- add headers
if req.source then
c.headers["transfer-encoding"] = "chunked"
c.headers["connection"] = "close"
c.headers["expect"] = "100-continue"
end
for i, header in ipairs(req.headers) do
local name, value = string.match(header, "^(.-):%s*(.*)$")
if name then
c.headers[string.lower(name)] = value
end
end
if not c.headers["host"] then
c.headers["host"] = u.authority
end
send(c.try, headers_to_string(c.headers))
send(c.try, "\r\n")
-- send request body
if req.source then
local source = req.source
while true do
local chunk = source()
if not chunk then
send(c.try, "0\r\n\r\n")
break
end
send(c.try, string.format("%x\r\n", string.len(chunk)))
send(c.try, chunk)
send(c.try, "\r\n")
end
end
c.try:settimeout(TIMEOUT, "t")
-- receive response
local status
local headers = {}
local body
status = receive(c.try, pat)
if status then
local ver, code, message = string.match(status, "^(%S+)%s+(%S+)%s+(.-)\r?$")
if ver and code and message then
status = {
major = tonumber(string.match(ver, "HTTP/(%d)%.%d")),
minor = tonumber(string.match(ver, "HTTP/%d%.(%d)")),
code = tonumber(code),
message = message
}
-- receive headers
local header_string, err = receive(c.try, "\r?\n\r?\n")
if header_string then
headers = headers_from_string(header_string)
-- handle 100 Continue responses
if status.code == 100 then
status, headers, body = request(req)
-- handle 300 redirects
elseif string.find(REDIRECT_CODES, code, 1, true) then
local location = headers.location
if location then
location = url.absolute(u, location)
if req.redirection then
if req.redirection.redirectcount >= DEFAULT_REDIRECT_TIMES then
return nil, "too many redirections"
end
if req.redirection.redirectstring:find(location.request, 1, true) then
return nil, "infinite redirection loop"
end
else
req.redirection = {
redirectcount = 0,
redirectstring = req.url.request,
}
end
req.url = location
close_connection(c)
return request(req)
end
-- handle 401 and 407 authentication requests
elseif string.find(AUTHREQUIRED_CODES, code, 1, true) then
if req.auth and c.headers.authorization then
return nil, "invalid authentication credentials"
end
local auth = headers["www-authenticate"]
or headers["proxy-authenticate"]
if auth then
local realm = string.match(auth, "realm=\"([^\"]*)\"")
if not realm then
realm = string.match(auth, "realm=([^,]*)")
end
if realm then
local user, password = req.auth(realm)
if user then
c.headers.authorization =
socket.try(socket.url.build({
scheme = "basic",
user = user,
password = password
}))
close_connection(c)
return request(req)
end
end
end
end
-- get response body
local length = tonumber(headers["content-length"])
if headers["transfer-encoding"] == "chunked" then
local decoder = socket.protect(function(chunk)
local size = tonumber(chunk, 16)
if size == 0 then return "" end
return receive(c.try, string.format("^([^\r\n]*)\r?\n(.*)\r?\n.{0,%d}$", size - 1), TIMEOUT)
end)
body = socket.sink("into-string")
while true do
local chunk, err = decoder()
if err or chunk == "" then break end
body(chunk)
end
elseif length then
body = receive(c.try, string.format("^%(.{%d})$", length), TIMEOUT)
elseif headers.connection == "close" then
body = receive(c.try, nil, TIMEOUT)
end
end
else
status = nil
end
if not status then
close_connection(c)
return nil, "invalid server response"
end
res.status = status
res.headers = headers
res.body = body
res.request = req
return res
else
close_connection(c)
return nil, "unable to receive status line"
end
end
-- HTTP/1.1 methods
function request_uri(u, req)
req = req or {}
req.method = req.method or "GET"
req.headers = req.headers or {}
req.url = url.absolute(u, req.url)
return request(req)
end
function request_string(u, s)
local req = {
method = "GET",
headers = {},
url = u,
source = socket.source("string", s),
}
req.headers["content-length"] = string.len(s)
return request(req)
end
function request_file(u, f)
local req = {
method = "PUT",
headers = {},
url = u,
source = socket.source("file", f),
}
req.headers["content-length"] = socket.filesize(f)
return request(req)
end
-- HTTP/1.0.0 methods
function get(u, headers)
return request_uri(u, { method = "GET", headers = headers or {} })
end
function post(u, body, headers)
return request_uri(u, {
method = "POST",
headers = headers or {},
source = socket.source("string", body),
})
end
function put(u, body, headers)
return request_uri(u, {
method = "PUT",
headers = headers or {},
source = socket.source("string", body),
})
end
function delete(u, headers)
return request_uri(u, { method = "DELETE", headers = headers or {} })
end
function options(u, headers)
return request_uri(u, { method = "OPTIONS", headers = headers or {} })
end
function trace(u, headers)
return request_uri(u, { method = "TRACE", headers = headers or {} })
end
-- exports
_headers_to_string = headers_to_string
_headers_from_string = headers_from_string
```
该模块提供了一系列 HTTP 请求方法,可以根据需要进行调用。其中,`request_uri` 方法可以实现对 URL 的请求,`get`、`post`、`put`、`delete`、`options`、`trace` 方法则分别对应了 HTTP 协议中的常见请求方法。
lua写A星算法
以下是一个简单的 Lua 实现 A* 算法的示例代码:
```lua
-- 定义节点结构体
local Node = {
x = 0, -- 节点的 x 坐标
y = 0, -- 节点的 y 坐标
g = 0, -- 从起点到当前节点的实际代价
h = 0, -- 从当前节点到目标节点的估计代价
f = 0, -- f = g + h
parent = nil, -- 父节点
}
-- 计算曼哈顿距离
local function manhattanDistance(node, target)
return math.abs(node.x - target.x) + math.abs(node.y - target.y)
end
-- A* 算法
local function aStar(start, target, map)
-- 初始化 open 和 close 列表
local open = {}
local close = {}
-- 将起点加入 open 列表
table.insert(open, start)
while #open > 0 do
-- 选取 f 值最小的节点
local current = open[1]
for i = 2, #open do
if open[i].f < current.f then
current = open[i]
end
end
-- 如果当前节点为目标节点,则返回路径
if current.x == target.x and current.y == target.y then
local path = {}
while current.parent ~= nil do
table.insert(path, 1, current)
current = current.parent
end
table.insert(path, 1, start)
return path
end
-- 将当前节点从 open 列表中移除,并加入 close 列表
for i = #open, 1, -1 do
if open[i].x == current.x and open[i].y == current.y then
table.remove(open, i)
break
end
end
table.insert(close, current)
-- 遍历当前节点的邻居节点
local neighbors = {}
if map[current.x - 1] and map[current.x - 1][current.y] then
table.insert(neighbors, map[current.x - 1][current.y])
end
if map[current.x + 1] and map[current.x + 1][current.y] then
table.insert(neighbors, map[current.x + 1][current.y])
end
if map[current.x][current.y - 1] then
table.insert(neighbors, map[current.x][current.y - 1])
end
if map[current.x][current.y + 1] then
table.insert(neighbors, map[current.x][current.y + 1])
end
for _, neighbor in ipairs(neighbors) do
-- 如果邻居节点已经在 close 列表中,则忽略
local isInClose = false
for i = 1, #close do
if close[i].x == neighbor.x and close[i].y == neighbor.y then
isInClose = true
break
end
end
if isInClose then
goto continue
end
-- 如果邻居节点不可通行,则忽略
if not neighbor.walkable then
goto continue
end
-- 计算邻居节点的实际代价
local g = current.g + 1
-- 如果邻居节点不在 open 列表中,则添加到 open 列表
local isInOpen = false
for i = 1, #open do
if open[i].x == neighbor.x and open[i].y == neighbor.y then
isInOpen = true
break
end
end
if not isInOpen then
neighbor.parent = current
neighbor.g = g
neighbor.h = manhattanDistance(neighbor, target)
neighbor.f = g + neighbor.h
table.insert(open, neighbor)
else
-- 如果邻居节点已经在 open 列表中,则更新 f 值和父节点
if g < neighbor.g then
neighbor.parent = current
neighbor.g = g
neighbor.f = g + neighbor.h
end
end
::continue::
end
end
-- 没有找到路径,返回空表
return {}
end
```
在使用该算法时,需要先定义地图和起点、目标点的位置。地图可以用一个二维数组来表示,每个元素表示一个节点,包含节点的 x、y 坐标和是否可通行等信息。下面是一个简单的示例:
```lua
local map = {
{ {x=1, y=1, walkable=true}, {x=1, y=2, walkable=true}, {x=1, y=3, walkable=true} },
{ {x=2, y=1, walkable=true}, {x=2, y=2, walkable=false}, {x=2, y=3, walkable=true} },
{ {x=3, y=1, walkable=true}, {x=3, y=2, walkable=true}, {x=3, y=3, walkable=true} },
}
local start = map[1][1]
local target = map[3][3]
local path = aStar(start, target, map)
```
该示例中,起点为地图左上角节点,目标点为地图右下角节点。节点的 `walkable` 属性表示是否可通行,其中 `false` 表示不可通行。算法返回的结果是一个包含路径节点的数组,按顺序表示从起点到目标点的路径。
相关推荐
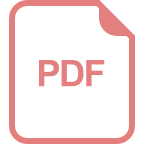
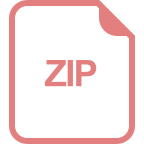












