如何使用C语言编写代码来计算并输出指定范围(数m和n之间)内的斐波那契数列?
时间: 2024-10-21 15:05:51 浏览: 19
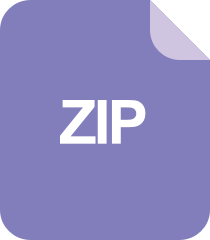
C语言计算斐波那契数列(Fibonacci sequence), vc 6++可运行源码

在C语言中,你可以使用循环结构来编写一个简单的函数,用于计算并输出指定范围内(从0到n)的斐波那契数列。以下是递归和迭代两种常见方法的示例:
**1. 递归法**(不太推荐,因为效率较低)
```c
#include <stdio.h>
// 计算斐波那契数列的递归函数
int fibonacci_recursive(int n) {
if (n <= 1)
return n;
else
return fibonacci_recursive(n - 1) + fibonacci_recursive(n - 2);
}
void print_fibonacci_recursively(int m, int n) {
for (int i = m; i <= n; ++i) {
printf("%d ", fibonacci_recursive(i));
}
printf("\n");
}
int main() {
int m = 0, n = 10; // 示例范围
print_fibonacci_recursively(m, n);
return 0;
}
```
**2. 迭代法**
```c
#include <stdio.h>
#define MAX_FIB 100
// 计算斐波那契数列的迭代函数
void print_fibonacci_iteratively(int m, int n) {
int t1 = 0, t2 = 1, next;
if (m > 0) {
printf("%d ", t1);
if (m > 1) printf("%d ", t2);
}
for (int i = 2; i < n && t1 + t2 <= MAX_FIB; ++i) {
next = t1 + t2;
printf("%d ", next);
t1 = t2;
t2 = next;
}
printf("\n");
}
int main() {
int m = 0, n = 10; // 示例范围
print_fibonacci_iteratively(m, n);
return 0;
}
```
在这两个例子中,`print_fibonacci_` 函数负责输出指定范围内的斐波那契数列。
阅读全文
相关推荐
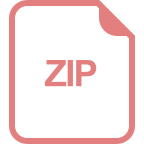
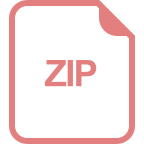















