def forward(self, x): input_shape = x.shape[-2:] x1 = x[:, :3, ...] x2 = x[:, 3:, ...] features1 = self.backbone1(x1) features2 = self.backbone2(x2)
时间: 2024-02-03 15:02:53 浏览: 65
这段代码是一个 PyTorch 的模型的前向传播函数。该模型输入一个张量 x,将其拆分成两个部分 x1 和 x2,并分别对它们进行特征提取。x1 和 x2 的维度分别为 (batch_size, 3, H, W) 和 (batch_size, C-3, H, W),其中 C 是 x 的通道数,H 和 W 分别是 x 的高度和宽度。特征提取是通过调用该模型中的两个 backbone 进行的,分别是 self.backbone1 和 self.backbone2。最终,该函数返回两个特征张量 features1 和 features2。
相关问题
class SelfAttention(nn.Module): def init(self, in_channels, reduction=4): super(SelfAttention, self).init() self.avg_pool = nn.AdaptiveAvgPool1d(1) # print("in_channels", in_channels) print("in_channels:", in_channels) print("reduction:", reduction) self.fc1 = nn.Conv1d(in_channels, in_channels // reduction, 1, bias=False) self.relu = nn.ReLU(inplace=True) self.fc2 = nn.Conv1d(in_channels // reduction, in_channels, 1, bias=False) self.sigmoid = nn.Sigmoid() def forward(self, x): b, c, n = x.size() print("x.shape=", x.shape) y = self.avg_pool(x) print("y.shape=", y.shape) # print("channel_out", channel_out) y = self.fc1(y) y = self.relu(y) y = self.fc2(y) y = self.sigmoid(y) return x * y.expand_as(x) def get_model(input_channels=6, use_xyz=True): return Pointnet2MSG(input_channels=input_channels, use_xyz=use_xyz) class Pointnet2MSG(nn.Module): def init(self, input_channels=6, use_xyz=True): super(Pointnet2MSG, self).init() self.SA_modules = nn.ModuleList() channel_in = input_channels # print("channel_in=", channel_in) skip_channel_list = [input_channels] for k in range(cfg.RPN.SA_CONFIG.NPOINTS.len()): mlps = cfg.RPN.SA_CONFIG.MLPS[k].copy() channel_out = 0 # print("channel_in=", channel_in) for idx in range(mlps.len()): mlps[idx] = [channel_in] + mlps[idx] channel_out += mlps[idx][-1] mlps.append(channel_out) # print(mlps) print("channel_out", channel_out) self.SA_modules.append( nn.Sequential( PointnetSAModuleMSG( npoint=cfg.RPN.SA_CONFIG.NPOINTS[k], radii=cfg.RPN.SA_CONFIG.RADIUS[k], nsamples=cfg.RPN.SA_CONFIG.NSAMPLE[k], mlps=mlps[:2], use_xyz=use_xyz, bn=cfg.RPN.USE_BN, ), SelfAttention(channel_out) ) ) skip_channel_list.append(channel_out) channel_in = channel_out根据以上代码块,自注意力机制模块中forward函数所接受的x参数的值到底是什么?是SA模块的输出张量吗?
是的,根据代码块中的逻辑,我们可以看出自注意力机制模块的输入张量x是SA模块的输出张量。在forward函数中,首先对输入张量的尺寸进行了打印,然后将输入张量通过平均池化层进行降采样,并对降采样后的张量进行了打印,接着将降采样后的张量通过两个1x1卷积层进行通道数的变换,并对变换后的张量进行了sigmoid激活函数的处理,最后将变换后的张量与原始输入张量进行点乘操作并返回,可以看出这里的输入张量就是SA模块的输出张量。
定义卷积神经网络实现宝石识别 # --------------------------------------------------------补充完成网络结构定义部分,实现宝石分类------------------------------------------------------------ class MyCNN(nn.Layer): def init(self): super(MyCNN,self).init() self.conv0=nn.Conv2D(in_channels=3, out_channels=64, kernel_size=3, stride=1) self.pool0=nn.MaxPool2D(kernel_size=2, stride=2) self.conv1=nn.Conv2D(in_channels=64, out_channels=128, kernel_size=4, stride=1) self.pool1=nn.MaxPool2D(kernel_size=2, stride=2) self.conv2=nn.Conv2D(in_channels=128, out_channels=50, kernel_size=5) self.pool2=nn.MaxPool2D(kernel_size=2, stride=2) self.conv3=nn.Conv2D(in_channels=50, out_channels=50, kernel_size=5) self.pool3=nn.MaxPool2D(kernel_size=2, stride=2) self.conv4=nn.Conv2D(in_channels=50, out_channels=50, kernel_size=5) self.pool4=nn.MaxPool2D(kernel_size=2, stride=2) self.fc1=nn.Linear(in_features=5033, out_features=25) def forward(self,input): print("input.shape:",input.shape) # 进行第一次卷积和池化操作 x=self.conv0(input) print("x.shape:",x.shape) x=self.pool0(x) print('x0.shape:',x.shape) # 进行第二次卷积和池化操作 x=self.conv1(x) print(x.shape) x=self.pool1(x) print('x1.shape:',x.shape) # 进行第三次卷积和池化操作 x=self.conv2(x) print(x.shape) x=self.pool2(x) print('x2.shape:',x.shape) # 进行第四次卷积和池化操作 x=self.conv3(x) print(x.shape) x=self.pool3(x) print('x3.shape:',x.shape) # 进行第五次卷积和池化操作 x=self.conv4(x) print(x.shape) x=self.pool4(x) print('x4.shape:',x.shape) # 将卷积层的输出展开成一维向量 x=paddle.reshape(x, shape=[-1, 5033]) print('x3.shape:',x.shape) # 进行全连接层操作 y=self.fc1(x) print('y.shape:', y.shape) return y改进代码
好的,这里是一个改进后的卷积神经网络实现宝石分类的代码:
```
import paddle
import paddle.nn as nn
class MyCNN(nn.Layer):
def __init__(self):
super(MyCNN, self).__init__()
self.conv1 = nn.Conv2D(in_channels=3, out_channels=32, kernel_size=3, padding=1)
self.bn1 = nn.BatchNorm2D(num_features=32)
self.relu1 = nn.ReLU()
self.pool1 = nn.MaxPool2D(kernel_size=2, stride=2)
self.conv2 = nn.Conv2D(in_channels=32, out_channels=64, kernel_size=3, padding=1)
self.bn2 = nn.BatchNorm2D(num_features=64)
self.relu2 = nn.ReLU()
self.pool2 = nn.MaxPool2D(kernel_size=2, stride=2)
self.conv3 = nn.Conv2D(in_channels=64, out_channels=128, kernel_size=3, padding=1)
self.bn3 = nn.BatchNorm2D(num_features=128)
self.relu3 = nn.ReLU()
self.pool3 = nn.MaxPool2D(kernel_size=2, stride=2)
self.conv4 = nn.Conv2D(in_channels=128, out_channels=256, kernel_size=3, padding=1)
self.bn4 = nn.BatchNorm2D(num_features=256)
self.relu4 = nn.ReLU()
self.pool4 = nn.MaxPool2D(kernel_size=2, stride=2)
self.fc1 = nn.Linear(in_features=256*6*6, out_features=512)
self.relu5 = nn.ReLU()
self.dropout1 = nn.Dropout(p=0.5)
self.fc2 = nn.Linear(in_features=512, out_features=256)
self.relu6 = nn.ReLU()
self.dropout2 = nn.Dropout(p=0.5)
self.fc3 = nn.Linear(in_features=256, out_features=25)
def forward(self, input):
x = self.conv1(input)
x = self.bn1(x)
x = self.relu1(x)
x = self.pool1(x)
x = self.conv2(x)
x = self.bn2(x)
x = self.relu2(x)
x = self.pool2(x)
x = self.conv3(x)
x = self.bn3(x)
x = self.relu3(x)
x = self.pool3(x)
x = self.conv4(x)
x = self.bn4(x)
x = self.relu4(x)
x = self.pool4(x)
x = paddle.flatten(x, start_axis=1, stop_axis=-1)
x = self.fc1(x)
x = self.relu5(x)
x = self.dropout1(x)
x = self.fc2(x)
x = self.relu6(x)
x = self.dropout2(x)
x = self.fc3(x)
return x
```
这个卷积神经网络包括了四个卷积层和三个全连接层。卷积层用于提取图像特征,全连接层用于将特征映射到分类标签。在卷积层中,我们使用了卷积、批量归一化、ReLU激活和最大池化等操作。在全连接层中,我们使用了ReLU激活和Dropout正则化。最后一层全连接层输出25个类别的概率得分,对应于25种不同的宝石类型。
阅读全文
相关推荐
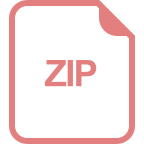
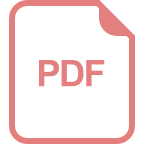


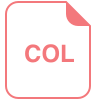
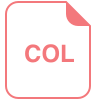
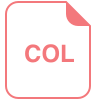
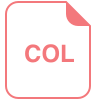
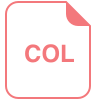
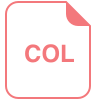
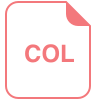
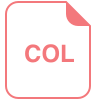


