python写spea2的算法程序
时间: 2023-11-27 15:55:41 浏览: 71
以下是一个简单的Python SPEA2算法实现示例:
```python
import random
import math
class SPEA2:
def __init__(self, population_size, archive_size, max_generations, tournament_size, mutation_rate, crossover_rate, problem_size, bounds):
self.population_size = population_size
self.archive_size = archive_size
self.max_generations = max_generations
self.tournament_size = tournament_size
self.mutation_rate = mutation_rate
self.crossover_rate = crossover_rate
self.problem_size = problem_size
self.bounds = bounds
self.population = []
self.archive = []
self.distances = {}
self.fitness_values = {}
def initialize_population(self):
for i in range(self.population_size):
individual = [random.uniform(self.bounds[j][0], self.bounds[j][1]) for j in range(self.problem_size)]
self.population.append(individual)
def evaluate_population(self):
for individual in self.population:
fitness = self.evaluate_fitness(individual)
self.fitness_values[tuple(individual)] = fitness
def evaluate_fitness(self, individual):
# 这里是你的适应度函数,需要根据具体问题进行实现
pass
def environmental_selection(self):
combined_population = self.population + self.archive
self.distances = self.calculate_distances(combined_population)
self.fitness_values.update(self.calculate_raw_fitness(combined_population))
# 将个体按照适应度值排序
sorted_individuals = sorted(list(self.fitness_values.items()), key=lambda x: x[1])
# 选取前archive_size个个体作为存档
self.archive = [list(individual) for individual, _ in sorted_individuals[:self.archive_size]]
# 选取前population_size个个体作为下一代种群
self.population = [list(individual) for individual, _ in sorted_individuals[:self.population_size]]
def calculate_distances(self, population):
distances = {}
for i in range(len(population)):
for j in range(i+1, len(population)):
dist = math.sqrt(sum([(population[i][k] - population[j][k])**2 for k in range(self.problem_size)]))
distances[(tuple(population[i]), tuple(population[j]))] = dist
return distances
def calculate_raw_fitness(self, population):
fitness_values = {}
for individual in population:
fitness = 0
for other_individual in population:
if individual != other_individual:
fitness += self.calc_distance(individual, other_individual)
fitness_values[tuple(individual)] = fitness
return fitness_values
def calc_distance(self, individual1, individual2):
# 计算两个个体的距离
if (tuple(individual1), tuple(individual2)) in self.distances:
return self.distances[(tuple(individual1), tuple(individual2))]
elif (tuple(individual2), tuple(individual1)) in self.distances:
return self.distances[(tuple(individual2), tuple(individual1))]
else:
dist = math.sqrt(sum([(individual1[k] - individual2[k])**2 for k in range(self.problem_size)]))
self.distances[(tuple(individual1), tuple(individual2))] = dist
return dist
def tournament_selection(self, population):
tournament = random.sample(population, self.tournament_size)
tournament.sort(key=lambda x: self.fitness_values[tuple(x)])
return tournament[0]
def crossover(self, parent1, parent2):
if random.random() < self.crossover_rate:
crossover_point = random.choice(range(self.problem_size))
child1 = parent1[:crossover_point] + parent2[crossover_point:]
child2 = parent2[:crossover_point] + parent1[crossover_point:]
return child1, child2
else:
return parent1, parent2
def mutation(self, individual):
mutated = False
for i in range(self.problem_size):
if random.random() < self.mutation_rate:
individual[i] += random.gauss(0, 1) * (self.bounds[i][1] - self.bounds[i][0]) / 10.0
mutated = True
if mutated:
return individual
else:
return None
def run(self):
self.initialize_population()
self.evaluate_population()
for generation in range(self.max_generations):
offspring = []
for i in range(self.population_size):
parent1 = self.tournament_selection(self.population)
parent2 = self.tournament_selection(self.population)
child1, child2 = self.crossover(parent1, parent2)
mutated_child1 = self.mutation(child1)
mutated_child2 = self.mutation(child2)
if mutated_child1:
offspring.append(mutated_child1)
if mutated_child2:
offspring.append(mutated_child2)
self.population.extend(offspring)
self.evaluate_population()
self.environmental_selection()
```
在使用时,需要根据具体的问题进行适应度函数的实现,并设置算法的超参数。
相关推荐
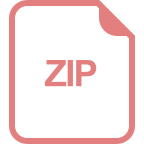














