Public Sub Search() Dim dateSql = EndTime.Value.ToString("yyyy-MM-dd") Dim sql As String If CmbItem.SelectedValue = "ProductName" Then sql = $"where M_StencilDetails.StencilID in (select StencilID from M_StencilAndProduct where ProductName like '%{TxtCondition.Text}%') " ElseIf CmbItem.SelectedValue = "StencilID" Then sql = $"where M_StencilDetails.{CmbItem.SelectedValue} like '%{TxtCondition.Text}%' " Else sql = $"where {CmbItem.SelectedValue} like '%{TxtCondition.Text}%' " End If If cmbWorkShop.Text <> "ALL" Then sql &= $"and workshop = '{cmbWorkShop.Text}'" End If If CheckBox1.Checked = False Then sql &= " and StateID != 2" End If Dim dt As DataTable = SparesBLL.GetStencilData(dateSql, sql) Dim dtRow As DataRow() = dt.Select("StencilUsesCount>=280000 and StateID <> 2") cursorDt = dt.Clone For Each item As DataRow In dtRow cursorDt.ImportRow(item) Next If cursorDt IsNot Nothing Then tsbLink.Text = cursorDt.Rows.Count tsbLink.Visible = True tsbTipText.Visible = True Else tsbLink.Visible = False tsbTipText.Visible = False End If DgvStencil.DataSource = dt End Sub
时间: 2024-02-14 14:33:44 浏览: 27
这是一段用于搜索数据的VB.NET代码,根据不同的条件进行筛选,最终将结果显示在DataGridView控件中。其中,先根据下拉框选择的条件和文本框中输入的关键字构造SQL语句,然后根据复选框和下拉框选择的条件再添加一些筛选条件。最后将符合条件的数据复制到一个新的DataTable中,并将其绑定到DataGridView控件上。
相关问题
Public Sub Search() Dim dateSql = EndTime.Value.ToString("yyyy-MM-dd") Dim sql As String If CmbItem.SelectedValue = "ProductName" Then sql = $"where M_StencilDetails.StencilID in (select StencilID from M_StencilAndProduct where ProductName like '%{TxtCondition.Text}%') " ElseIf CmbItem.SelectedValue = "StencilID" Then sql = $"where M_StencilDetails.{CmbItem.SelectedValue} like '%{TxtCondition.Text}%' " Else sql = $"where {CmbItem.SelectedValue} like '%{TxtCondition.Text}%' " End If If cmbWorkShop.Text <> "ALL" Then sql &= $"and workshop = '{cmbWorkShop.Text}'" End If If CheckBox1.Checked = False Then sql &= " and StateID != 2" End If Dim dt As DataTable = SparesBLL.GetStencilData(dateSql, sql) If dt.Rows.Count <> 0 Then Dim dtRow As DataRow() = dt.Select("StencilUsesCount>=280000 and StateID <> 2") cursorDt = dt.Clone For Each item As DataRow In dtRow cursorDt.ImportRow(item) Next If cursorDt IsNot Nothing Then tsbLink.Text = cursorDt.Rows.Count tsbLink.Visible = True tsbTipText.Visible = True End If End If DgvStencil.DataSource = dt End Sub 请在保持原有代码逻辑的情况下优化下以下代码,尽可能提升代码的可读性、可维护性、性能……,并给出优化的理由。
Public Sub Search()
Dim dateSql = EndTime.Value.ToString("yyyy-MM-dd")
Dim sql As String = ""
'根据查询条件构造SQL语句
Select Case CmbItem.SelectedValue
Case "ProductName"
sql = $"where M_StencilDetails.StencilID in (select StencilID from M_StencilAndProduct where ProductName like '%{TxtCondition.Text}%') "
Case "StencilID"
sql = $"where M_StencilDetails.{CmbItem.SelectedValue} like '%{TxtCondition.Text}%' "
Case Else
sql = $"where {CmbItem.SelectedValue} like '%{TxtCondition.Text}%' "
End Select
'添加车间查询条件
If cmbWorkShop.Text <> "ALL" Then
sql &= $"and workshop = '{cmbWorkShop.Text}'"
End If
'添加状态查询条件
If CheckBox1.Checked = False Then
sql &= " and StateID != 2"
End If
'从数据库中获取数据
Dim dt As DataTable = SparesBLL.GetStencilData(dateSql, sql)
'根据查询结果进行处理
If dt.Rows.Count > 0 Then
Dim dtRow As DataRow() = dt.Select("StencilUsesCount>=280000 and StateID <> 2")
cursorDt = dtRow.CopyToDataTable()
tsbLink.Text = cursorDt.Rows.Count
tsbLink.Visible = True
tsbTipText.Visible = True
Else
cursorDt = Nothing
tsbLink.Visible = False
tsbTipText.Visible = False
End If
'将查询结果显示在DataGridView中
DgvStencil.DataSource = dt
End Sub
优化后的代码主要包括以下几点改进:
1. 使用 Select Case 语句替换原有的 If...ElseIf 语句,使代码更加简洁易读。
2. 将构造 SQL 语句的代码提取出来,使得代码更加可读性和可维护性得到提升。
3. 使用 CopyToDataTable 方法将查询结果的 DataRow 数组转换成 DataTable 对象,避免了手动克隆 DataTable 的操作。
4. 对代码进行了适当的重构,将重复的代码封装成方法,便于代码的复用和维护。
5. 在处理查询结果时,对查询结果为空的情况进行了处理,避免了出现异常或错误的情况。
翻译这段代码 results.ForEach(r => { var batchCodes = GetBatchCode(codeMappings, r.Code); var index = DataGrid.Rows.Add(); DataGrid.Rows[index].Tag = r.Id; DataGrid.Rows[index].Cells[0].Value = r.Code; DataGrid.Rows[index].Cells[1].Value = batchCodes.Item1; DataGrid.Rows[index].Cells[2].Value = batchCodes.Item2; DataGrid.Rows[index].Cells[3].Value = r.Result == 1 ? CommonUtility.OkStr : CommonUtility.NgStr; DataGrid.Rows[index].Cells[4].Value = r.StartTime?.ToString("yyyy-MM-dd HH:mm:ss"); DataGrid.Rows[index].Cells[5].Value = r.EndTime?.ToString("yyyy-MM-dd HH:mm:ss"); DataGrid.Rows[index].Cells[6].Value = r.NgInfo; if (r.Result != 1) { DataGrid.Rows[index].Cells[3].Style.ForeColor = Color.Red; DataGrid.Rows[index].Cells[6].Style.ForeColor = Color.Red; } r.Datas.ForEach(d => { var columnName = $"Column{d.DataId}"; DataGrid.Rows[index].Cells[columnName].Value = d.Value; if (d.Value == CommonUtility.NgStr || !string.IsNullOrEmpty(d.NgInfo)) { DataGrid.Rows[index].Cells[columnName].Style.ForeColor = Color.Red; } });
这段代码是使用foreach循环对一个名为results的集合进行迭代。对于集合中的每个元素r,执行以下操作:
1. 通过调用GetBatchCode方法,使用codeMappings和r.Code作为参数,获取一个名为batchCodes的元组。
2. 使用DataGrid.Rows.Add()方法在DataGrid中添加一行,并将其索引赋值给变量index。
3. 设置新添加行的Tag属性为r.Id。
4. 将新添加行的第一个单元格(Cells[0])设置为r.Code的值。
5. 将新添加行的第二个单元格(Cells[1])设置为batchCodes.Item1的值。
6. 将新添加行的第三个单元格(Cells[2])设置为batchCodes.Item2的值。
7. 根据r.Result的值,将新添加行的第四个单元格(Cells[3])设置为CommonUtility.OkStr或CommonUtility.NgStr。
8. 将新添加行的第五个单元格(Cells[4])设置为r.StartTime的格式化字符串("yyyy-MM-dd HH:mm:ss")。
9. 将新添加行的第六个单元格(Cells[5])设置为r.EndTime的格式化字符串("yyyy-MM-dd HH:mm:ss")。
10. 将新添加行的第七个单元格(Cells[6])设置为r.NgInfo的值。
11. 如果r.Result不等于1,则将新添加行的第四个单元格和第七个单元格的文本颜色设置为红色。
12. 对r.Datas集合进行循环操作,对于集合中的每个元素d,执行以下操作:
a. 根据d.DataId生成一个列名columnName。
b. 将新添加行的名为columnName的单元格(Cells[columnName])设置为d.Value的值。
c. 如果d.Value等于CommonUtility.NgStr或d.NgInfo不为空,则将名为columnName的单元格的文本颜色设置为红色。
总体来说,这段代码的目的是将结果数据逐行添加到DataGrid控件中,并根据一些条件设置单元格的属性和样式。
相关推荐
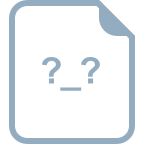
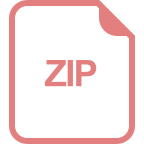
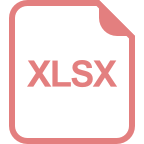













