帮我用python写一个将极坐标转换为xy坐标的程序
时间: 2023-06-03 08:06:17 浏览: 186
可以的,请参考以下的Python程序:
```python
import math
def polar_to_cartesian(r, theta):
x = r * math.cos(theta)
y = r * math.sin(theta)
return x, y
radius = float(input("请输入半径: "))
angle = float(input("请输入角度: "))
x, y = polar_to_cartesian(radius, math.radians(angle))
print("x坐标为:", x)
print("y坐标为:", y)
```
输入半径和角度,程序将返回对应的x坐标和y坐标。
相关问题
python极坐标如何将网格去除,换成十字坐标,90度设为箭头,标注北
在Python的matplotlib库中,如果你想在极坐标图上呈现网格,通常网格会默认存在,但是可以手动设置`grid`属性为`False`来去除网格。同时,为了实现十字坐标并用箭头标记90度方向,以及标注"北",我们需要自定义一些额外的元素,例如刻度、标签和箭头。
以下是代码示例:
```python
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.axes_grid1 import host_subplot
def add_crosshair(ax, x, y):
# 添加交叉线
ax.axvline(x=x, color="black", linestyle="--")
ax.axhline(y=y, color="black", linestyle="--")
# 添加箭头标记90度
ax.annotate("", xy=(x, y), xytext=(-15, 15),
arrowprops=dict(facecolor='black', shrink=0.05))
# 标注“北”
ax.text(x, y, "北", ha="center", va="bottom", rotation=0)
# 使用host_subplot创建无网格的主轴
host = host_subplot(111, polar=True)
plt.subplots_adjust(right=0.75) # 确保右下角有空间放置辅助轴
par = host.twin()
# 创建极坐标网格
host.axis["grid"] = False
# 转换三角形顶点为极坐标
vertices = [(0, 0), (0, 849), (np.radians(22.5), 1429)]
for v in vertices:
add_crosshair(host, *v)
# 绘制三角形的每一边
for i in range(len(vertices) - 1):
host.plot([vertices[i][1], vertices[i + 1][1]], [vertices[i][0], vertices[i + 1][0]])
# 添加辅助轴显示角度
par.axis["theta"].set_visible(True)
par.set_yticklabels(["0°", "90°", "180°", "270°"])
par.grid(False)
plt.show()
```
这个例子中,我们使用了`mpl_toolkits.axes_grid1.host_subplot`来创建一个无网格的主轴,然后手动添加十字线、箭头和标签。
python 中怎么在极坐标轴中绘制中心位置可自己设定的椭圆,椭圆长轴为3,短轴为1.8
你可以使用 Matplotlib 库中的 `Ellipse` 类来绘制椭圆。以下是一个示例代码,可以在极坐标轴中绘制中心位置可自己设定的椭圆,椭圆长轴为3,短轴为1.8:
``` python
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.patches import Ellipse
# 极坐标系下的椭圆参数
center = (2, np.pi/3) # 中心位置
width = 3 # 长轴
height = 1.8 # 短轴
# 极坐标系下的椭圆转换为直角坐标系下的椭圆
angle = center[1]
x, y = center[0] * np.cos(angle), center[0] * np.sin(angle)
ell = Ellipse(xy=(x, y), width=width, height=height, angle=np.rad2deg(angle))
# 绘制极坐标轴
fig = plt.figure()
ax = fig.add_subplot(111, projection='polar')
# 绘制椭圆
ax.add_artist(ell)
ell.set_clip_box(ax.bbox)
ell.set_alpha(0.5)
ell.set_facecolor('red')
# 显示图像
plt.show()
```
运行结果如下图所示:

阅读全文
相关推荐
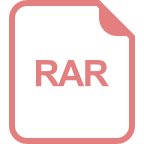
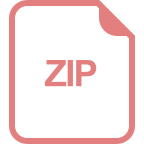
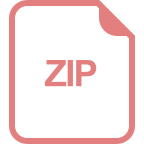
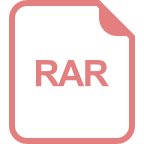
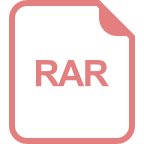
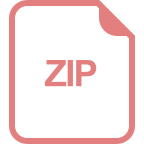
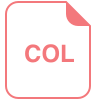
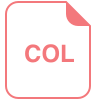
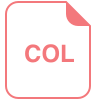





